Reference no: EM131043123
Objectives
- Analyse, develop, and implement software solutions
- Choose and compare appropriate data structures and algorithms in program design
- Apply classes, inheritance, polymorphism, and exception handling
- Test software implementations to ensure correctness and maintainability
Assessment Task
Part A
In this assignment, you are required to write a Java Application that uses an interactive Graphical User Interface (GUI) based on the JFrame class using SWING GUI components. You will also be designing and implementing the software solution using appropriate data structures and application of classes, inheritance, and exception handling. The case study for developing a solution is given below.
The food consumption of a person is to meet the daily energy requirements. The energy requirement is estimated using a person's height, weight, Basal metabolic rate (BMR), and physical activity level (PAL). There is data available on estimated energy requirements for various PALs, and BMR. So by choosing the correct height and PAL, the energy requirements can be found from the data set. In Assignment One you have developed a Java Application that lets the user to enter height and choose the most suitable PAL and thereby display the energy requirements in Mega Joules.
In this assignment you extend your first assignment so that the user can save the user details of name, height, age group, gender, PAL level, energy requirement, and expected weight to a database table named userEnergy. Also your application should display all the user's data from the database. In addition to the GUI components you have in Assignment One, include the following components.
1. Save Button in the bottom panel, to save the user data to the database.
2. Display All Button in the bottom panel to display the saved data of all users from the database.
The functions of two additional buttons are described below.
1. Save
The Save Button can be used to save the User Energy needs to the data base.
Note: Use the given data file named COIT20256Ass1Data.csv available on the course website and it contains the set of data.
2. Display All
This Button enables the user to view all the database records displaying user details and their energy requirements. The database records will have a unique id. It is not essential to display the id.
The screen with additional buttons are shown below.
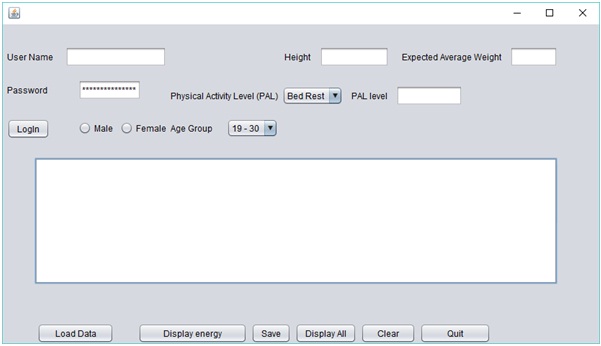
Initial Screen Display
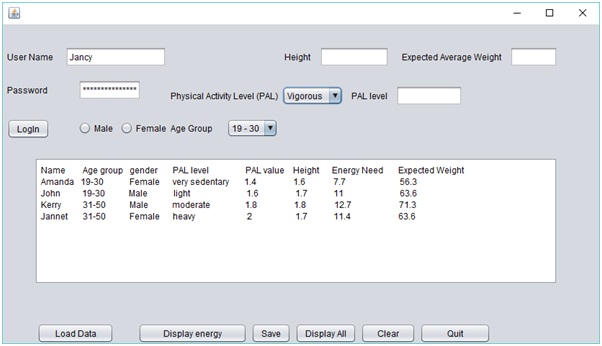
Screen Display on Clicking "Display All"
Data Structures
DatabaseUtility class: Create this class to implement all the tasks related to creation of database and table, extracting records from the database. Include a linkedList of UserEnergyNeeds class to store the extracted data from the database. Use Prepared statements to get the user input from GUI interface and use them for populating database. Also use prepared statement to execute query to extract data and store the records in a linked list.
Software Tools for Building the Application
You can build your application using the TextPad Editor or NetBeans. It is highly recommended that you create the GUI components using code rather than 'designer' and 'click and drag' of the NetBeans. This creates code which is not maintainable. Remember this is a good prototyping tool, but not recommended for coding.
Part B
Your task in this part of the Assignment is to demonstrate use of various data structures such as stack, priority queue, set, and map using the given data.
While using these data structures to store explain the benefits you can achieve by using the appropriate data structures. You need to complete the following steps in use of various data structures.
1. Read the names from the file and load in a priority queue. Display the values from the priority queue using the peek() method. Describe your observation of change to the list of names in the report file.
2. Move the list of names from the priority queue to stack. Move the list of names from stack while displaying the value to a set. Describe your observation of the stack list of names.
3. Display the names from the set and again describe your observation the list of names.
4. Create a map and store the names with associated student Id and display the values.Part B
Your task in this part of the Assignment is to demonstrate use of various data structures such as stack, priority queue, set, and map using the given data.
While using these data structures to store explain the benefits you can achieve by using the appropriate data structures. You need to complete the following steps in use of various data structures.
1. Read the names from the file and load in a priority queue. Display the values from the priority queue using the peek() method. Describe your observation of change to the list of names in the report file.
2. Move the list of names from the priority queue to stack. Move the list of names from stack while displaying the value to a set. Describe your observation of the stack list of names.
3. Display the names from the set and again describe your observation the list of names.
4. Create a map and store the names with associated student Id and display the values.
The reference should be in Harvard style.
Attachment:- COIT20256Ass2T1Data2016.csv