Background
Texturing is like wallpapering; you are pasting an image onto the OpenGL Quad primitive. Recall that GL_QUAD is specified by four vertices. An image, or a texture, also has four vertices. However, they have different coordinate systems:
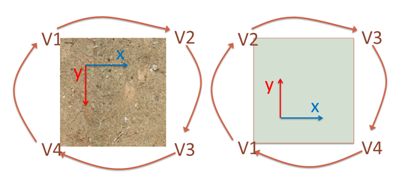
Make sure you align your image/texture vertices with the correct GL_QUAD vertices!!!
When we read our TGA image files, we start at the top, left corner and read left to right (like we normally read books!). So, for a texture, point (0,0) ALWAYS specifies the top, left corner. Then, (1,0), specifies the top, right corner; (0,1) is the x, y coordinate to specify the bottom, left; and (1,1) specifies the bottom right. When specifying texture vertices and GL_QUAD vertices, MAKE SURE YOU USE THE SAME WINDING ORDER (e.g., clockwise).
Can I specify a texture coordinate between 0 and 1? Yes! If you let the x coordinates be 0 and 0.5, you would draw from the far left side (where x==0) to the middle of the image (where x=0.5). Likewise for the y coordinate.
Can I specify a texture coordinate greater than 1? Yes! If the texture is set up to repeat, OpenGL will just repeat the texture that many times. So, if x was 0 to 4 and y was 0 to 2, OpenG would repeat the texture 4 times in the X direction and 2 times in the Y direction, on the single quad you specified with GL_QUADS. However, in this lab, except for GROUND, the textures are NOT set up to repeat.
The code provided for this Workshop has a lot going on. We read the image files, set up texturing within OpenGL, and we set up alpha (transparency). Most of this work is done outside the main program file. Check out Texture.cpp and Texture.h, if you are interested in all the dirty details.
If not, know that we have preloaded 15 textures for you to use today:
GROUND, LAKE , DUCK, FLOWER1, FLOWER2, WELL, MOUNTAINS, STONE, TREE1, TREE2, TREE3, PINETREE1, PINETREE2, CLOUD1, and CLOUD2
Let's assume we want to draw a ground texture. First, we need to tell OpenGL which texture we want to draw. Next, we draw the GL_QUAD and at the same time, we tell OpenGL how to paste the image onto the quad. Think of it like per-vertex coloring! So, to draw a 2x2 ground square, centered at zero, and textured with the GROUND texture, we would say:
// Tell OpenGL which texture you want to draw
glBindTexture(GL_TEXTURE_2D, textureStruct[GROUND].texID);
glBegin(GL_QUADS);
// Draw a ground square - 2x2
glTexCoord2f(1.0f, 1.0f); glVertex3f(-1.0f, 0.0f, -1.0f);
glTexCoord2f(0.0f, 1.0f); glVertex3f( 1.0f, 0.0f, -1.0f);
glTexCoord2f(0.0f, 0.0f); glVertex3f( 1.0f, 0.0f, 1.0f);
glTexCoord2f(1.0f, 0.0f); glVertex3f(-1.0f, 0.0f, 1.0f);
glEnd();
Interaction
This week the code base includes some keyboard interaction! Use the "up" and "down" arrows to move on the z-axis. The "PgUp" and "PgDn" buttons move along the y-axis. The "a" and "s" buttons move along the x-axis. Finally, the "left" and "right" arrows rotate left and right; that is, the left and right arrows rotate about the y-axis.
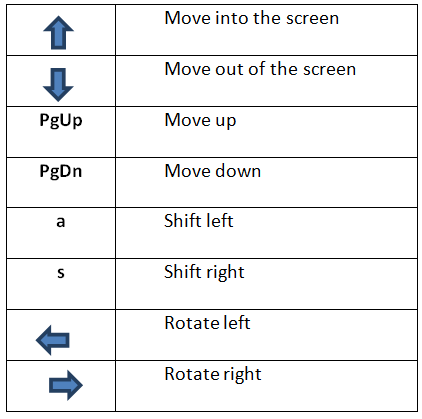
Deliverables - Exercise
1-Draw a tree with TREE1 texture(15 points)
Draw a tree using the preloaded TREE1 texture. Note that this is still a square image file; it looks like a tree because we have transparency (alpha) set up! Draw the tree facing the user so that your instructor can check your texturing orientations. Your tree should be oriented like this:
2- Create a Virtual World (65 points)
In this exercise, you will create a simple worldwith the preloaded textures. You must add at least five (5) different objects. You must use at least three (3) different textures for your objects. (These requirements are in addition to the ground, which was drawn for you, and the tree, which was drawn in the previous exercise). Feel free to add more objects and/or more textures. Have fun!!!
3- Revisit OpenGL transformation order (20 points)
Find the lines of code that allow the user to translate and rotate inside the world. Hint: The lines are at the beginning of the display function. Copy the lines of code here, and then reverse the order that the transformations happen. Answer the following questions:
1. Copy Code here:
2. Please fully describe how the user was INITIALLY moving about the world. In other words, describe what happened when you pressed the "up" arrow? What happened when you pressed the left arrow? And so on. Did you always move on a certain axis, or did that change after rotating?
3. Please fully describe how the user moves about the world AFTER you switched the order of the transformation. What changed? Why did it change?
Submit this document with your answers to Exercise #3 andyour code for your virtual world to Scholar(the whole folder in a zip file or the .cpp file you edited, whichever your instructor asks for). This is anINDIVIDUAL assignment, though you can ask your teammates for assistance, you must submit individually.
Rename the project folder as LastName_FirstName_TextureLab before you zip.