Reference no: EM131099779
Assignment - Implementation of Algorithms and Data Structures
Question 1.
a. Implement a generic Stack<T> class using an array T[] for storing elements. Your class should include a constructor method, which takes as argument the capacity of the stack, push, pop and peek methods, and asize method which returns the number of elements stored. Implement dynamic resizing for this stack.
b. Write a program to test your implementation of this Stack class. You also need to provide at least 4 test cases for this program to test all the methods including constructor, push, pop, peek and size, and to test dynamic resizing.
Question 2.
a. Implement a genericQueue<T> class that has enqueue, dequeue and peek methods and all of these methods must be O(1) time. This class also has a min methodwhich returns the minimum value stored in the queue, and throws an exception if the queue is empty. Assume elements are comparable. If the queue contains n elements, you can use O(n) space, in addition to what is required for the elements themselves.
b. Write a program to test your implementation of this Queue<T> class. You also need to provide at least 4 test cases for this program to test all the methods including enqueue, dequeue, peek and min.
Question 3.
a. Implement a generic queueclass namedInefficientQueue<T> in terms of twointernal stacks. The methods required in this class are enqueue, dequeue, andpeek. The goal of this problem is to get you to use the stack abstraction while implementing aqueue abstraction. This method for implementing a queue is quite inefficient (thus the name of the class). Why is this so?
b. Write a program to test your implementation of this queue class. You also need to provide at least 4 test cases for this program to test all the methods including enqueue, dequeue and peek.
Question 4.
Implement the RemoveBefore(int nodeValue)[1 mark] and RemoveAfter(int nodeValue)[1 mark] methods for a LinkedListclass.
The RemoveBefore (RemoveAfter) method removes the node before (after) the node having the given node value. The internal class Node[1 mark] to build the LinkedList class has only an integer value, nodeValue, and a single link, next, that links to the node just behind the given node.You also need to implement the Add(int nodeValue) method [1 mark] for the LinkedList class using the internal Node class.
Question 5.
a. Write a method that takes a sorted integer array A and a key k and returns the index of the first occurrence of k in A. Return -1 if k does not appear in A. For example, when applied to the array in Figure 12.1 below, your algorithm should return 3 if k = 108; if k = 285, your algorithm should return 6.
b. Write a Java program to test this method and provide at least 4 test cases for this program.
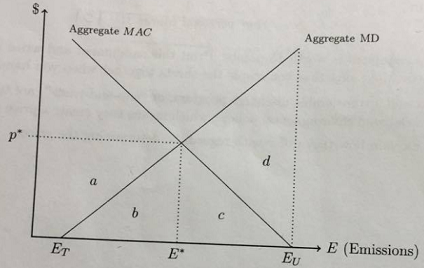
References:
[1] Gayle Laakmann. Cracking the Coding Interview.
[2] Adnan Aziz, Tsung-Hsien Lee and Amit Prakash. Elements of Program Interviews.
What is the total revenue joe can earn in a year
: What are the total opportunity costs of producing ten boats (explicit and implicit)?
|
Excessively suddenly stops the alcohol use
: Alcohol withdrawal occurs when a person who uses alcohol excessively suddenly stops the alcohol use. Studies have shown that the onset of withdrawal is experienced a mean of 39.5 hours after the last drink, with a standard deviation of 18 hours.
|
Create a table named faculty to store facultyid
: Create a table named Faculty to store FacultyID( Primary key), FirstName, LastName, Email, Date of birth and number of courses taught to date. You should select the appropriate data types and constraints for the table.
|
Find the amount credited and the outstanding balance
: 1. How much would have to be paid on an invoice for $328 with terms of 2/10 ROG if the merchandise invoice is dated January 3, the merchandise arrives January 8, and the invoice is paid (a) January 11; (b) January 25?
|
Write a program to test your implementation of stack class
: Write a program to test your implementation of this Stack class. You also need to provide at least 4 test cases for this program to test all the methods including constructor, push, pop, peek and size, and to test dynamic resizing
|
What has happened to your accounting profit
: What has happened to your accounting profit?
|
Traditional and continuing features of the japanese economy
: Discuss in details four traditional and continuing features of the Japanese economy and what should the Japanese government do about Abenomics?
|
The shift from personal to personalized computing
: This is 4 pages article, i need a 1 or 1 1/2 pages summary , The power of many, The shift from personal to personalized computing
|
Credited and the outstanding balance on an invoice
: Find the amount credited and the outstanding balance on an invoice dated August 19 if a partial payment of $500 is paid on August 25 and has terms of 3/10, 1/15, n/30. The amount of the invoice is $826
|