Reference no: EM131372219
Part 1: Solving a quadratic equation
This exercise is similar to what you should have already completed for one of the exercises in Chapter 3 of the MATLAB course notes. Note however, that it is not exactly the same as the format of inputting and outputting values differs from the example in the notes.
Write a MATLAB function that solves a quadratic equation of the form
ax2 + bx + c = 0
The syntax of your function should take the form
>> [quadRoots] = Q1 STNO(coeff);
where STNO should be replaced by your student number.
The function should accept one input argument, coeff, a three element vector of the quadratic coefficients in the order a, b, c.
Your function should return one output argument, quadRoots. For the case where two roots are present, this should be a two-element vector of them. For the case where one root is present, the output should be a scalar of that value. For the case where no roots are present, the output should be a scalar set to NaN (not a number, see Section 1.3.3 of the course notes).
It is permissible for coeff and quadRoots to take the form of either row or column vectors.
Code format and error-free execution
Output when two roots are present
Output when one root is present
Output when no roots are present
Part 2: Factorials
The factorial of a positive integer is defined as:
n! = n × (n - 1) × (n - 2) × (n - 3) × . . . × 1
where n! = 1 when n = 0. For example, 5! = 5 × 4 × 3 × 2 × 1 = 120.
The double factorial of a positive odd integer is defined as:
n!! = n × (n - 2) × (n - 4) × . . . × 1
where n!! = 1 when n = 0. For example, 9!! = 9 × 7 × 5 × 3 × 1 = 945.
Write a MATLAB function that computes both the factorial and double factorial for a positive odd integer.
The syntax of your function should take the form
>> [fact,DblFact] = Q2 STNO(n);
where n is the number your wish to find the factorials of, and fact and DblFact are the factorial and double factorial respectively.
Add a few error checks to the input to check that is sensible. (You might need to do a small amount of background research to find a way to verify the input is a odd integer).
Code format and error-free execution
Factorial
Double factorial
Error checking
Part 3: A vertically falling ball
The drag force D(t) as a function of time t acting on an object falling at velocity v(t) is given by
D(t) = cd1/2ρv(t)2 A
where cd is a dimensionless constant known as the drag coefficient that depends on the geometry of the object (use cd = 0.47 for a sphere), ρ is the density of air (use ρ = 1.2 kg/m3) and A is the cross-sectional area, which is circular for a ball.
The total force on a falling ball is thus the difference between drag and gravity:
F(t) = cd1/2ρv(t)2A - mg
where g is the acceleration due to gravity = 9.81 m/s2.
The acceleration a(t) is therefore (using Newton's Second Law):
a(t) = (cdρA/2m)v(t)2 - g (1)
Note that all the terms here are constant except for a(t) and v(t).
Because the acceleration is not constant, we cannot use "suvat" equations but can ap- proximate velocities sequentially using:
vn = vn-1 + anδt (2)
where vn and an approximate the velocity and acceleration at time tn (= nδt) respectively, and δt is the time step between values (see Annex 1 for derivation). Please use δt = 0.1s for this assignment.
Write a MATLAB function to calculate the time taken tThresh (in seconds) for a vertically falling ball of mass m (in kg) and diameter d (in m) to reach a velocity of vThresh in the presence of drag assuming the initial velocity is zero. Your function should also return the terminal velocity vTerm and the fraction of the terminal velocity reached at this time (e.g. if vTerm = 50 m/s and vThresh = 25 m/s, then the fraction is 0.5).
The syntax for your code should take the form
>> [tThresh,vTerm,vFrac] = Q3.STNO(m,d,vThresh)
where m and d are the mass and diameter of the ball respectively and vThresh is the velocity at the required time.
[Hint: You may download Q3 TEMPLATE.m to use as a starting point. Assume that the mass of the ball falls in the range 5 kg to 20 kg and that the diameter is in the range 5 cm to 50 cm.]
Error free execution
Calculated time
Terminal velocity
Fraction of terminal velocity
Part 4: Bouncing ball
N.B. This part of the assignment is designed to be challenging with very limited help available!
In an ultra-exciting physics experiment, some students plan to launch a bouncy ball from a toy cannon. Assuming that the ball is launched at velocity v (in m/s) and elevation angle θ (in radians measured from the horizontal ground to direction of cannon), write a MATLAB function that plots the trajectory of the ball for the first two bounces on a well-labelled graph. Unlike for Part 3, assume no drag.
Your code should take the form:
>> [d] = Q4 STNO(v,theta,c)
where d is a two-element vector indicating the distances of the first two points of contact with the ground from the cannon, v and theta are the launch velocity and angle as stated above, and c is defined below. An example output is shown in Figure 1 for clarity.
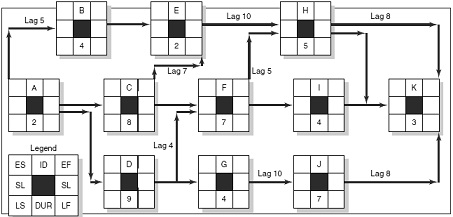
Figure 1: Sample result. In this case, d would be approximately [10.2 17.8].
Assumptions:
- No drag/wind.
- The ball is launched from the ground.
- The ground is perfectly flat and level with no obstacles.
- There is no spin on the ball and no friction between the ball and the surface: hence no change in horizontal velocity after each bounce.
- Vertical velocity changes by a multiplicative factor of -c at the instant of each bounce.
Distance to bounces
Graph (trajectory)
Graph (style, clarity, labelling)
For a final challenge, add two additional inputs to specify the distance from the origin and the height of a thin wall. Your function should then plot the new trajectory including a rebound from the wall. Assume that the bounce between the ball and wall does not affect the magnitude of the velocity (unlike the bounce with the ground).
If you attempt this final part then please submit two functions for Part 4: one without this final calculation as previously ([d] = Q4 STNO(v,theta,c)) and one of the form:
>> [d] = Q4e STNO(v,theta,c,D,H)
where D and H refer to the distance and height of the wall respectively. You should plot the wall on the graph using the MATLAB line function (type help line into the MATLAB command window for more details).