Reference no: EM13558
Many bank ATM machines have recently added the ability to dispense cash with- drawals in multiple bill denominations chosen by the customer. For example, the new touchscreen Chase ATM machines allow the customer to withdraw bills in denominations of $100, $20, $5, and $1. Below is a schematic diagram of what a screen might look like for a customer who is requesting a with-drawal of $179.
Although I am not privy to exactly how the programming is done for this kind of machine, I suspect that it is implemented using some variation of the Model-View-Controller (MVC) paradigm.
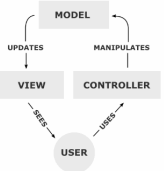
The model object(s) represents all the data and business logic that is driving the entire withdrawal workflow. The view object pulls the data it displays from the model(s). The controller object responds to user interaction events (such as pushing a button) by calling methods on the appropriate model objects.
Define a single class called Withdrawal that could serve as a model for the ATM machine. No view or controller classes need to be defined. The program's main function could serve as a text-based user interface substitute for a view and controller. Or it can simply instantiate (construct) a Withdrawal object, and call various methods on it to adequately test the program.
Withdrawal Class Data Members
An instance of the Withdrawal class should have three data members (corresponding to the light blue shaded areas in the UI illustration above): amount_ an integer representing the withdrawal amount bill_denominations_ a vector of integers such as [100, 20, 5, 1] bill_quantities_ a vector of integers such as [1, 3, 2, 4]
Doxygen Comments
Students should annotate each public member function with Doxygen comments using the following tags:
@brief one line summary describing what the function does
@param describe the meaning of each parameter
@return describe the meaning the return value
For example the C library function pow(double x, double y) might be documented as:
/**
* @brief exponentiation function
* @param x the base of the exponentiation
* @param y the power the base is raised to
* @return x raised to the power y
*/
Withdrawal Class Implementation
The most difficult member function to implement is the constructor itself. Note that it must initialize all three data members, but the constructor is only supplied with two parameters. The "extra" data member (bill_quantities_) must be calculated from the two parameters using logic. The simplest way to do this is to repeated use integer division (/) and remainder (%) operations.
The getter member functions are routine. The calculator member functions are fairly straightforward. The controller will call the Nudge member function when a ⊕ or ? button is pushed and will modify the element of the bill_quantities_ data member with the supplied index by the given delta.
The main Function
As mentioned previously, the main function can either be:
- an interactive program; or
- a non-interactive program that constructs one or more instances of the Withdrawal class and calls various member functions on them.