Reference no: EM131225711
Assignment - Repetition and Decomposition/Functions
Problem Statement:
Write a program, my_math.cpp, that defines 5 mathematical function and calculates the summation or integration of that function. You are creating your own library of commonly used mathematical functions and analyzing the different methods, rectangle vs. trapezoid methods, for solving integration problems to gain experience writing a short program, which uses selection, repetition, and functions/procedures.
Your program should define these 5 functions:
f1(x) = 2x5 + x3 - 10x + 2 f2(x) = 6x2 - x + 10
f3(x) = 5x + 3 f4(x) = 2x3 + 120
f5(x) = 2x2
Summation Function Explanation:
We will use the functions specified above, where x = a to b.
bΣx=af5(x)
- The summation needs a and b values for the beginning and ending values for x.
- Calculate the summation of the function, f5(x)=2x2, with a user specified a and b value
- Return the sum.
Integration Functions Explanation:
Your program should determine the area under the functions specified above. You will calculate the area under a curve using the rectangle and trapezoid method. Therefore, you need integrate_rectangle() and integrate_trapezoid() functions. Don't freak out!!! Programming integration into the computer doesn't consist of calculus. It is simple math, and these two methods only require summing the area of a certain number of rectangles or trapezoids under a specific curve, f(x).
For Example, if your f(x) = 2x:
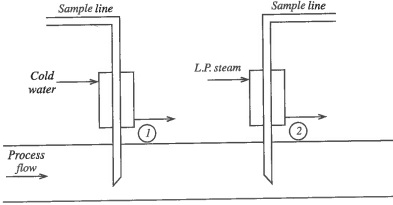
Basic Numerical Methods (Rectangle vs. Trapezoid):

n = number of rectangles and/or trapezoids
a = beginning x value
b = boundary for rectangles
width = width of each rectangle/trapezoid, (b-a)/n
f(x) = height of rectangle
(f(x1)+f(x2))/2 = height of trapezoid Area = width * height
The functions are bounded by any interval on the x-axis, including both positive and negative values!!!.
You can check your answers to these functions using
https://www.wolframalpha.com/widgets/view.jsp?id=8ab70731b1553f17c11a3bbc87e0b605
Here is an example run of your my_math.cpp program:
Choose a function (1, 2, 3, 4, 5, other(quit)): 5
Which operation do you want (summation(1) or integration(2)): 2
Would you like to calculate the area using the rectangle, trapezoid, or both (1, 2, 3): 2
How many trapezoids do you want? 1000 Please select a starting point, a = 1 Please select an ending point, b = 2
The area under 2x2 between 1 and 2 is 4.667
Program Input:
- Mathematical operation to perform
- Starting and ending points for the area or summation
- Function to calculate the area or summation, i.e. f1, f2, f3, f4, f5
- Function/Procedure(s) for calculating the area, i.e. rect, trap, both
- Number of rectangles and/or trapezoids to use
Program Output:
- The function being evaluated
- Starting and ending points for the integral or summation
- For integration: Number of rectangles and/or trapezoids used
- The area or summation calculated by the method(s)
Program Description in more detail:
Your program needs to adhere to the following guidelines:
- If the user chooses to see the area calculated by both methods, each method should receive their own number of rectangles or trapezoids as input and return the value from the calculation.
- Your program should continue running until the user no longer wants to perform more calculations.
- You should use procedural decomposition and have functions for f1(x), f2(x), f3(x), f4(x), and f5(x), as well as functions for calculating the summation and area using the rectangle vs. trapezoid method.
Program Style/Comments
In your implementation, make sure that you include a program header in your program, in addition to proper indentation/spacing and other comments! Below is an example header to include. Make sure you review the style guidelines for this class, and begin trying to follow them, i.e. don't align everything on the left or put everything on one line! https://classes.engr.oregonstate.edu/eecs/fall2015/cs161-001/161_style_guideline.pdf
/******************************************************
** Program: my_math.cpp
** Author: Your Name
** Date: 10/20/2015
** Description:
** Input:
** Output:
******************************************************/
Testing
Report any checking/self-reflection you did while solving the problem. For instance, how did you make sense of the output from the implementation? This includes things such as using a calculator to make sure the output is correct, testing to make sure your code executes correctly and behaves the way you expect under specific circumstances, using external sources of information such as the internet to make sense of the results, etc. In addition, you will provide us a test plan!
You will be graded on how thorough your test plan is. Make sure you think about input you hope works and input that won't work. Your program doesn't have to handle input that doesn't work!!!