Reference no: EM131227715
Problem 1
Create a complete Java program in a class named Bday that declares four variables and assigns appropriate values to them.
your birthday month (1-12)
your birthday day (1-31)
the birthday month of another student sitting near you today (1-12)
the birthday day of that same student near you (1-31)
Ask your neighbor for their name and for the proper numbers to store in the variables for his/her birthday. Then produce output in this format using your four variables:
My birthday is 9/19, and Suzy's is 6/14.
Problem 2 : Syntax errors(10)
The following program contains 9 mistakes! What are they? Copy and paste the following code into Jgrasp/Eclipse and correct the various mistakes.
public class Oops {
public static void main(String[] args) {
int x;
System.out.println("x is" x);
int x = 15.2; // set x to 15.2
System.out.println("x is now + x");
int y; // set y to 1 more than x
y = int x + 1;
System.out.println("x and y are " + x + and + y);
}
}
Problem 3:
Write a for loop that produces the song Bottles of Beer on the Wall:
10 bottles of beer on the wall, 10 bottles of beer
Take one down, pass it around, 9 bottles of beer on the wall
9 bottles of beer on the wall, 9 bottles of beer
Take one down, pass it around, 8 bottles of beer on the wall
... (output continues in the same pattern) ...
1 bottles of beer on the wall, 1 bottles of beer
Take one down, pass it around, 0 bottles of beer on the wall
Problem 4:
Write a complete Java program that produces this sequence of numbers using a for loop:
8
11
14
17
20
23
Problem 5:
Write a program to produce the following output using nested for loops. Use a table to help you figure out the patterns of characters on each line.
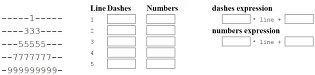
Test your loop expressions in Practice-It! using the checkmark icon above. Use your expressions in the loop tests of the inner loops of your code.
Problem 6:
Consider the following method for converting milliseconds into days:
// converts milliseconds to days
public static double toDays(double millis) {
return millis / 1000.0 / 60.0 / 60.0 / 24.0;
}
Write a similar method named area that takes as a parameter the radius of a circle and that returns the area of the circle. For example, the call area (2.0) should return 12.566370614359172. Recall that area can be computed as π times the radius squared and that Java has a constant called Math. PT.
Problem 7:
Write a method named pay that accepts two parameters: a real number for a TA's salary, and an integer for the number of hours the TA worked this week. The method should return how much money to pay the TA. For example, the call pay (5 . 50, 6 ) should return 33.0.
The TA should receive "overtime" pay of 1 V2. normal salary for any hours above 8. For example, the call pay (4.00, 11) should return (4.00 * 8) + (6.00 * 3) or 50.0.
What would happen it choynski set target revenues and
: Plot the monthly data and the regression lines for each of the following cost functions: Which cost driver for manufacturing overhead costs would you choose? Explain. Choynski anticipates 2,600 machine-hours and 300 batches for next month. Using the ..
|
What risk factors for teen substance abuse are present
: What risk factors for teen substance abuse are present in Thelma's life? What practical action would you recommend for Thelma to fight against the guilt and anger she feels about the situation with her father?
|
Compute the cost allocation rate for each activity
: Sawyer Pharmaceuticals manufactures an over-the-counter allergy medication called Breathe. Compute the cost allocation rate for each activity
|
What ethical issues may arise in this specific situation
: In a 2-3 page paper, describe the organization you selected and then discuss the value and advantages of an intake interview for the organization. What situation would it be used in? Why would an intake or counseling interview be a good choice for..
|
Write a complete java program that produces the sequence
: Write a complete Java program that produces this sequence of numbers using a for loop. Create a complete Java program in a class named Bday that declares four variables and assigns appropriate values to them.
|
What two legal issues associated with clinical psychology
: What are at least two cultural limitations associated with assessment and treatment? In your response, discuss the use or misuse of assessment instruments, therapy techniques, research results, or any other facet of clinical practice that could ha..
|
Ideal routing of a mobile network
: Why might a home agent be the ideal routing of a mobile network?
|
Friend in every countryin the world
: Let the domain for x be the set of all students in this class and the domain for y be theset of all countries in the world. Let P(x, y) denote student x has visited country y andQ(x, y) denote student x has a friend in country y. Express each of t..
|
Identify the price level
: In the diagram, sketch a line showing long-run aggregate supply at a potential output of $10 trillion. Sketch in an aggregate demand curve and identify the price level. Illustrate the long-run effect of an increase in the money supply.
|