Reference no: EM131280682
Part I: Theoretical Questions
1. What is Type Casting in C#? Name the two forms of Type Castings in C#. Why is it needed?
2. What are the access modifiers in C#?
3. State the different ways of passing parameters to a method in C#.
4. Does C# support multiple inheritance? Explain how multiple inheritance can be implemented in C# with the help of an example.
5. Consider the following code. Convert the if-else statement to switch-case.
if (opt == 1)
{
result = num1 + num2;
Console.WriteLine("\n{0} + {1} = {2}", num1, num2, result); }
else if (opt == 2)
{
result = num1 - num2;
Console.WriteLine("\n{0} - {1} = {2}", num1, num2, result); }
else if (opt == 3)
{
result = num1 * num2;
Console.WriteLine("\n{0} * {1} = {2}", num1, num2, result);
}
else if (opt == 4)
{
result = (float)num1 / num2;
Console.WriteLine("\n{0} / {1} = {2}", num1, num2, result);
}
else { Console.WriteLine("\nInvalid option.Please try again.");
}
Part II: Output and Debugging Questions
Note: Provide a copy of the code and screen shot for the output in the solutions
1. Consider the program below. Will the program generate an output? If not, why? Write the corrected program.
class Program
{
static void Main(string [] args)
{
const int a = 5;
const int b = 6;
for (int i = 1; i <= 5; i++)
{
a = a * i;
b = b * i;
}
Console.WriteLine(a);
Console.WriteLine(b);
Console.ReadLine();
}
}
2. What is the output of the following program?
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
int p, q;
int[,] M = new int[5, 5];
for (p = 0; p < 5; ++p)
{
for (q = 0; q < 5; ++q)
{
M[p, q] = p * 1 + q * 1;
Console.Write(M[p, q] + " ");
} Console.Write("\n");
} Console.ReadLine();
}
}
}
3. Identify the errors in the following C# program. Write the correct program. [4 marks]
class Program
{
static void Main(string[] args)
{
/ This is a comment
integer m=9,
Console.WriteLine("value = ', m);
}
}
Part III- Problem Solving Questions
Note: Provide a copy of the code and screen shot for the output in the solutions
1. Using loops, write a C# program which accepts the number of rows and then prints a pattern according to the number of rows as shown below.
Enter number of rows :6
$
$$
$$$
$$$$
$$$$$
2. Write a C# program to:-
a. Define a two dimensional array of three rows and three columns.
b. Read array elements from the keyboard.
c. Print the array elements.
d. Calculate the sum of diagonal elements and print the sum.
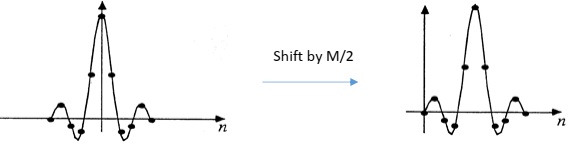
3. Write a C# program to
a. Input a string from the keyboard
b. Print the given string
c. Reverse the string and print it.
d. Print the reversed string in capital letters.
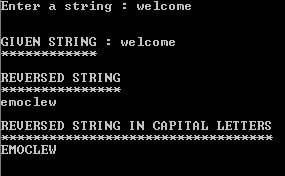
How much power is being supplied to the pump
: Water at 40 °C is pumped from an open tank through 200 m of 50-mm-diameter smooth horizontal pipe as shown in Fig. P12.22 and discharges into the atmosphere with a velocity of 3 m/s. Minor losses are negligible.
|
Explain how conflicts can be constructive
: Explain how conflicts can be constructive. What are superordinate goals and how do they help resolve conflicts between antagonistic groups? What are superordinate goals and how do they help resolve conflicts between antagonistic groups? How does dive..
|
Different kinds of involvement
: Is it always good? What key elements are necessary to keep it going? Are there different kinds of involvement?
|
Predict the future of healthcare delivery in the us
: For our final discussion of the class, I would like for you to predict the future of healthcare delivery in the U.S. Review the resources for this week and critique how well you really think they'll work.
|
What is type casting in c#
: M107 - What is Type Casting in C#? Name the two forms of Type Castings in C#. Why is it needed and what are the access modifiers in C# - state the different ways of passing parameters to a method in C#.
|
How receiver of delta sigma modulation system is simplified
: 1-bit modulation merely restates that the quantizer consists of a hard limiter with only two representation levels. Explain how the receiver of the delta-sigma modulation system is simplified, compared with conventional DM.
|
Chinese government owns the land
: Chinese government owns the land). If you are in Dubai, you can purchase alcohol, but you will need a special license to purchase alcohol. You will also learn that school tuition cost more than an Ivy League education, but fortunately, your comp..
|
Explain how conflicts can be constructive
: How would you analyze supply and demand for health care services? Do you ever think that health care moves too fast in initiating or adopting change? Why or why not? How would you handle a client that is constantly in with the project team and direct..
|
The importance of the project leaders attitude
: The importance of the project leader’s attitude and leadership in reporting progress to stakeholders and senior management.
|