Reference no: EM131061972
Task 1 - Square Tile Generator
Define a function createSquareTile(numTiles, prefix) which generates a specified number of solid colour square tile pictures with the size and colour of each picture specified by the user. It accepts two input parameters:
- numTiles - int, the number of square tiles to be created.
- prefix - str, the prefix of the file names for the square tiles to be generated.
The function MUST follow the program flow on page 4. For each square tile being generated, the function should create a JPEG file using the specified prefix. For example, createSquareTile(3, "tile") will create three files named tile1.jpg, tile2.jpg and tile3.jpg. Depending on the size and colour specified by the user for each tile, the generated pictures may look like:
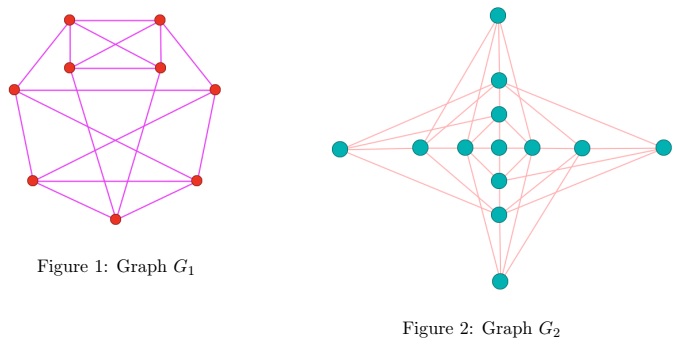
Note: In a solid colour picture, every pixel has the exactly same colour.
Task 2 - Tint a Picture in a Circular Region Using a Colour Gradient
Define a function gradTintPicInCircle(bgPic, solidPic1, solidPic2, intensity, centreX, centreY, radius) that applies a tint, based on a colour gradient, to a specified circular region over a background picture. This function will return the tinted background picture as a Picture object, and accepts the following 8 input parameters:
- bgPic - Picture, the background picture to be tinted.
- solidPic1 - Picture, the 1st solid colour picture that provides the starting colour of the colour gradient. In this assignment, you should always use a solid colour picture generated by Task 1.
- solidPic2 - Picture, the 2nd solid colour picture that provides the ending colour of the colour gradient. In this assignment, you should always use a solid colour picture generated by Task 1.
- intensity - int, the strength of the tint in percentage. For example, a value of 30 means 30%.
- centreX - int, the centre position of the circular region on the X axis of the background picture.
- centreY - int, the centre position of the circular region on the Y axis of the background picture.
- radius - int, the radius of the circular region.
To tint a background picture using one colour (rtint, gtint, btint), the colour of each pixel in the tinted background picture is calculated by:
rbgtint = rbg + (rtint - rbg) x intensity/100.0
gbgtint = gbg + (gtint - rbg) x intensity/100.0
bbgtint = bbg + (btint - bbg) x intensity/100.0
To tint a background picture using a colour gradient, the tint colour to be applied to a pixel in the background picture depends on the coordinate of that pixel in the background picture and should be calculated accordingly.
You may think of this process as firstly creating a colour gradient picture of the same size to the background picture and then using the colour of each pixel in the created colour gradient picture to tint the corresponding pixel in the background picture.
This function requires the colour gradient to be defined from right to left with respect to the background picture, which means the starting and ending colours of a colour gradient should be applied to the rightmost and leftmost columns of the background picture, respectively. Furthermore, the tint should ONLY be applied to the pixels within the specified circular region.
Below are three sample pictures created by invoking function gradTintPicInCircle using different inputs. The pictures on the left and in the middle used the provided RMIT building picture as the background picture. The picture on the right used an empty canvas as the background picture to better visualize the tint effect. Note: The specified circular region may exceed the border of the background picture as illustrated by the middle picture.
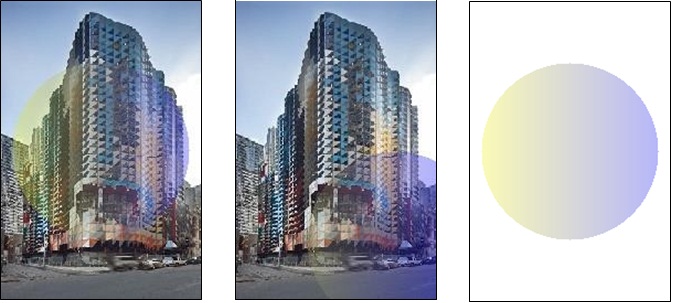
Skeleton Code
You MUST use the provided skeleton code and follow its instructions to complete this assignment. In the skeleton code, there is a predefined main function and two incomplete function definitions. You should complete these two incomplete function dentitions so that when function main is executed, it generates three square tile pictures using createSquareTile and three tinted pictures using gradTintPicInCircle.
Demonstration (Week 11 or 12)
The whole assignment group must demonstrate the assignment in your allocated tute/lab class prior to the final submission. If two group members come from different classes, please choose one of the two classes to make the demonstration. Subject to the on-time final submission and the plagiarism detection, you will receive preliminary marks for your demonstration, and each member must be able to clearly explain the entire program.
You may choose to demonstrate your code in Week 11 or Week 12 and you must make your final code submission by 11:59pm May 27 (Friday) 2016.
Program Flow for Task 1
You should follow the following program flow to define function createSquareTile:
Based on the specified numTiles, loop numTiles times. In each iteration,
1. Check if it is the very first iteration (i.e. the first tile to be created):
a. For the first iteration, ask the user for an integer using a dialog popup with the following message:
Please enter the size of Tile 1:
If the entered size is non-positive, display an error message via a dialog popup and then repeat Step 1-a.
b. For subsequent iterations, ask the user the following question using a dialog popup:
Same size as the previous tile? (Y/N):
i. If the answer is "Y", use the size of the previous tile and go to Step 2.
ii. If the answer is "N", ask the user to enter the size of the tile to be created:
Please enter the size of Tile #: (Replace # with the tile number)
If the entered size is non-positive, display an error message via a dialog popup and then repeat Step 1-b-ii.
iii. If the answer is neither "Y" or "N", display an appropriate message via a dialog popup and then repeat Step 1-b.
2. Ask the user to pick a colour using a colour picker popup.
3. Create a new Picture object based on the specified size and colour.
4. Save the created Picture object as a JPEG file based on the specified prefix, for example, when the prefix is "image", the 3rd square tile being created will be named "image3.jpg".
There will be no program flow provided for Task 2. Should you need any help, please discuss with your assignment partner (if applicable) or seek assistance from your tutor in the practical class.