Reference no: EM131071018
Assignment
Objectives
This assignment will provide practice and experience in:
• Designing an application - Topic 02
• Developing applications - Topic 02
• Variables and constants - Topic 03
• Decisions and conditions - Topic 04
• Modularisation - Topic 05
• Arrays and structures - Topic 08
• File handling - Topic 10
NB Depending on when you start this assignment you may need to read ahead especially on how to use files.
Suggestions:
Read the assignment specifications carefully first. In Week 2 start making notes on what is required. By Week 3 you should be ready to make the first version of the program based on your screen design. Week 5 consider what parts of your code should be modularised. By Week 8 you should be able to implement the arrays. By Week 10 implement file handling. Finalise the program in Week 11.
Background
Data provided by third parties is often not in an immediately useful format. Sometimes due to the quantity and/or complexity of the data it is better to write a program to process it rather than using a spreadsheet or word processor. For this exercise we will be using weather data from the Australian Bureau of Meteorology but note that the skills that you develop in this exercise can be applied to any similar problem, e.g. currency fluctuations, ocean temperatures over time, university admission data and so on.
You are required to write a program that will calculate the average rainfall and wind speed for a single day using weather data downloaded from the Australian Bureau of Meteorology. The data file can be downloaded from https://www.bom.gov.au/nsw/observations/nswall.shtml or alternatively you can use the one uploaded with this document. Select any single town, e.g. Ballina and scroll to the bottom of the page and click the link which will look similar to that below:
25/01:00pm 31.4 34.2 24.3 66 5.0
Other formats:
Comma delimited format used in spreadsheet applications
https://www.bom.gov.au/fwo/IDN60801/IDN60801.94596.axf
This file should be saved to your bin/debug folder and submitted along with the rest of your assignment. You may choose your own location and date to download, e.g. I chose Ballina and 23rd January.
Specifications
You are required to design and write a VB Windows application using Visual Studio 2013 to display data and to extract average wind speed and rainfall from a downloaded data file. Your program must:
• use a common dialog control for loading and saving data;
• have at least one general procedure;
• must demonstrate the use of an array;
• must use at least one try catch;
• You must use the data file as downloaded from the Internet. You are not allowed to modify the data in the data file before using it in your application;
• Your program must display the data in the file;
• Your program must display the average wind speed and the average rainfall;
• Your program must save the modified data (see step 4 below) to a new file.
The program does not have to be fully completed to earn marks but your submission must be satisfactory and a reasonable attempt. See the general instructions in mySCU/Assignments/Marking Criteria for clarification of satisfactory and reasonable. If you have any queries about the assignment please use the discussion board.
The marks will be allocated as follows:
• Design 30% (Sketch, Plan of objects and properties, Plan of event procedures)
• Style 30% (See style guidelines)
• Functionality 40% (as per specifications)
Advice and hints
I suggest that you develop your application using the following steps:
1) Write the code to open the file and copy the ‘raw' data from the file into a ListBox.
2) Write the code to save the data in the ListBox to a new file.
3) Write the code to remove unwanted data so that you just have the lines of data we are interested in. Keep using the ListBox so that you can know you have got it right. The first item in your ListBox should be something like: 0,94596,"Ballina","IDN60801","23/02:00pm","20150123140000","20150123030000"... You will need to study the raw data file carefully to decide how to remove the unwanted
data. I suggest loading it into Word so that you can see how the file is formatted, i.e. where the line breaks are. Click this icon in Word to see the line break character:
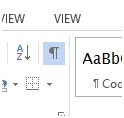
Show or hide formatting symbols
4) Process each line of data so that each data item is stored in a separate memory location, i.e. you could use variables, an array or an array of structs. Note that in the original data file the first line following [DATA] identifies what each data item is, i.e.
sort_order
|
wmo
|
name[80]
|
history_product[80]
|
local_date_time[80]
|
local_date_time_full[80]
|
aifstime_utc[80]
|
0
|
94596
|
"Ballina"
|
"IDN60801"
|
"23/02:00pm"
|
"20150123140000"
|
"20150123030000"
|
You will also need to remove double quotes. One way to do this is to process the string one character at a time skipping over the double quotes and building a new string. At this stage you should be able to display something like 0,94596,Ballina,IDN60801,23/02:00pm,20150123140000,20150123030000...
5) Now you need to assign the individual data items to variables or to an array. One way is to use the split method.
6) The rainfall data is rain_trace[80] and the wind speed is wind_spd_kmh. Once you have isolated these values it should be reasonably straightforward to calculate the average rainfall and windspeed.
7) Finally make use of the discussion board and internal workshops to clarify any aspect of this assignment.