Reference no: EM13541171
Question 1:
Use a one-dimensional array to solve the following problem: A company pays its salespeople on a commission basis. The salespeople receive $200 per week, plus 9% of their gross sales for that week. For example, a salesperson who grosses $5,000 in sales in a week receives $200 plus 9% of $5,000, a total of $ 650. Write an app (using an array of counters) that determines how many of the salespeople earned salaries in each of the following ranges (assuming that each salesperson's salary is truncated to an integer amount): $200-299,
$300-399, $400-499, $500-599, $600-699, $700-799, $800-899, $900-999 and over $999.
Write an application that prompts the user to enter the sales for each employee, then it calculates the salesperson's salary. The process repeats until the user finishes entering all employees' information. When the user is done entering this information, it displays how many of the salespeople earned salaries in each of the above ranges.
Question 2:
Standard telephone keypads contain the digits zero through nine. The numbers two through nine each have three letters associated with them. Many people find it difficult to memorize phone numbers, so they use the correspondence between digits and letters to develop seven-letter words that correspond to their phone numbers. For example, a person whose telephone number is 686-2377 might use the correspondence indicated in Fig. 7.29 to develop the seven-letter word "NUMBERS." Every seven-letter word corresponds to exactly one seven-digit telephone number. A restaurant wishing to increase itstakeout business could surely do so with the number 825-3688 (that is, "TAKEOUT").
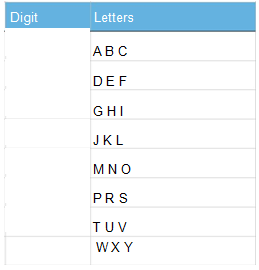
Every seven-letter phone number corresponds to many different seven-letter combinations. Unfortunately, most of these represent unrecognizable juxtapositions of letters. It's possible, however, that the owner of a barbershop would be pleased to know that the shop's telephone number, 424-7288, corresponds to "HAIRCUT." A veterinarian with the phone number 738-2273 would be pleased to know that the number corresponds to the letters "PETCARE." An automotive dealership would be pleased to know that the dealership number, 639-2277, corresponds to "NEW-CARS."
Write an app that allows the user to enter a seven-digit number, and displays every possible seven-letter word combination corresponding to that number. Avoid phone numbers with the digits 0 and 1.
Question 3:
A bank wants you to create a console app that will allow bank employees to view the clients' information. The app should prompt the user to enter first name, last name, account number and account balance. It should provide options to allow the bank manager to search through each client's information backward and forward. Create a Customer class to represent the client with first name, last name, account number and account balance. The application should use an array of Customer objects to store customer information. DO NOT use any collection classes such as ArrayList, LinkedList, HashSet etc. You can only use the following statement that assumes the maximum number of customers is 100
Customer [] customerList = new Customer[100];
Question 4:
This assignment is based on the principles of clock consistency and drifts in a distributed system. You have to create a simulation, running on a single machine, of a simple distributed system involving a master object (MO) and four process objects (PO). The MO and each PO will contain a logical clock, a concept rst proposed by Lamport.1 The concept of the logical clocks along with the following technique, which is based on the Berkley Algorithm,2 will attempt to resolve the clock consistency in this system:
1. Each event (send or receive) in the system is associated with a time-stamp, based on logical clocks.
2. Each PO, Pi, will have an associated logical clock, LCi. This logical clock is implemented as a simple counter that is incremented between any successive events executed within that PO. Since a logical clock has a monotonically increasing value, it assigns a unique number to every event. The time stamp of an event is the value of the logical clock for that event. Hence, if an event A occurs before an event B in Pi, then LCi(A) < LCi(B).
3. The MO will also contain a logical clock, that will be incremented periodically and as indicated in the next point.
4. After every t units of logical time (a parameter that can be varied), each PO will send its current local time (i.e., value of its logical clock) to the MO. Once a MO receives such a message from any PO, it will average all ve values (i.e., logical clock values of four POs and its logical clock value) and will consider it to be the correct logical clock value. It will then calculate an o set (either positive or negative) for each PO's logical clock and send it to each PO. It will also adjust its logical clock to that correct clock value.
5. A PO will advance its logical clock when it receives a message from the MO containing an o set. If Pi receives a message from MO with an o set ti (could be positive or negative) then Pi should adjust its clock such that LCi = LCi + ti + 1.
6. A Pi will randomly decide, in addition to sending a message to the MO, if it wants to send a message to one or more other Pj s. Upon receipt of such a message, each Pj will also advance its logical clock if the time stamp associated with the incoming message is greater than the current value of its logical clock, i.e., if Pj , receives a message (event B) from Pi with a time stamp t and LCi(B) t then Pj should advance its logical clock such that LCi(B) = t + 1.
7. Any Pi, in the system, may exhibit Byzantine or arbitrary failures.
Your task is:
1. Propose the interaction and failure models for this system. Discuss the pros and cons of your design.
2. To simulate this entire environment in Java using threads. Allow the simulation to reach a steady-state, i.e., run the program for a large number of iterations. During each iteration and at the end of the simulation compare the values of the logical clocks of POs with that of the MO. Print out the clock drifts (i.e., di erence between the logical clocks of the MO and each PO) for all POs. Repeat the simulation with di erent probabilities and access their e ects on the clock drifts.