Reference no: EM131299522
1) Very basic circuits 0.5 Amps pass through a 100-Ohm resistor. What is the voltage across the resistor? What is the law that you are using to solve this problem?
2) Given the circuit in the below figure. Ignore B1 and B2, they are included for completeness only. Current directions should be as they are here and you should not assume anything regarding the directions.
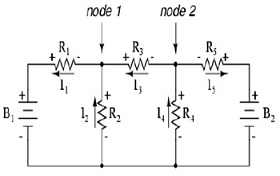
a. Use Kirchoffs Current Law to find I3, if I1 = 4 Amps and I2 =1 Amps, at node 1.
b. At node 2, If I4 is 5 Amps, what is I5?
3) Short answer questions:
a. Mention three different methods to program an Arduino processing board? 1.
b. Provide the C (not Ardiuno) program statement to set ONLY PORTB pins 1 and 7 to logic one. Use bit-manipulation techniques.
c. Provide the C (not Ardiuno) program statement to reset ONLY PORTB pins 1 and 7 to logic zero. Use bit-manipulation techniques.
d. Given a sinusoid with 500 Hz frequency, what should be the minimum sampling frequency for an analog-to-digital converter, if we want to faithfully reconstruct the analog signal after the conversion? What is the name of the theorem that solves this problem? This was discussed in the Lecture several times.
e. If an analog signal is converted by an analog-to-digital converter to a binary representation and then back to an analog voltage using a DAC, will the original analog input voltage be the same as the resulting analog output voltage?
Circle your answer: YES NO. Explain your answer very briefly.
f. Describe the flow of events when an interrupt occurs.
g. There are two methods to doing GPIO that we discussed in Lecture. Name them. In the lecture, we had a funny unscientific name to one of them, mention it.
4) Given the code below:
a. Comment on each line of code as to what does that line of code do.
Use the comment symbols
b. Convert the Ardiuno Library functions except delay() to C code that manipulates the registers of the PORTs.
int led = 13;
voidsetup() {
pinMode(led, OUTPUT);
} void loop() {
digitalWrite(led, HIGH);
delay(1000);
digitalWrite(led, LOW);
delay(1000);
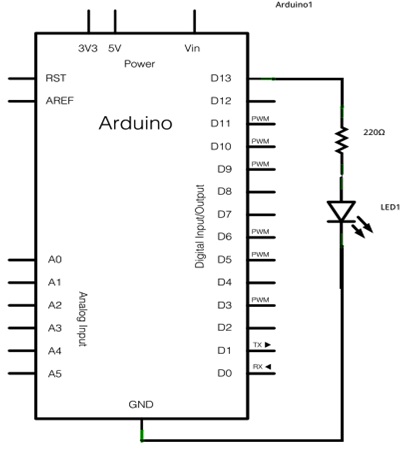
5) A Temperature sensor circuit is described in the code below.
a. Construct the circuit similar to the figure above i.e. draw the circuit schematic.
b. Modify the behavior of the circuit you have drawn in part a above and change the code below as necessary to light up a single LED for 100 milliseconds if the temperature goes above 75 degrees F.
c. Which of these two variables degreesC or degreesFis declared in the code below? Which one can you compare against?
d. Answer the questions in the comment next to each statement in the code.
e. Add the necessary modifications to the circuit that would cope with your changes to light up the LED when the temperature is between 75 degrees F and 85 degrees.
/*TEMPERATURE SENSOR
Use the "serial monitor" window to read a temperature sensor.
The TMP36 is an easy-to-use temperature sensor that outputs a voltage that's proportional to the ambient temperature.
Hardware connections:
The temperature sensor has a triangle logo and "TMP" in very tiny letters.
When looking at the flat side of the temperature sensor with the pins down, from left to right the pins are: 5V, SIGNAL, and GND.
Connect the 5V pin to 5 Volts (5V).
Connect the SIGNAL pin to ANALOG pin 0.
Connect the GND pin to ground (GND).
We'll use analog input 0 to measure the temperature sensor's signal pin. It analog input pin reads a value between 0 and 1024.
*/
constinttemperaturePin= 0;
void setup()
{
// What does this function do?
Serial.begin(9600);
}
// Why does this function return void?
void loop()
{
floatvoltage, degreesC, degreesF;
// What is the purpose of each of the statements below?
voltage= getVoltage(temperaturePin); //
// Now we'll convert the voltage to degrees Celsius.
degreesC= (voltage - 0.5) * 100.0; //
degreesF= degreesC* (9.0/5.0) + 32.0; //
Serial.print("voltage: ");
Serial.print(voltage);
Serial.print(" deg C: ");
Serial.print(degreesC);
Serial.print(" deg F: ");
Serial.println(degreesF);
delay(1000);
}
floatgetVoltage(intpin)
{
return(analogRead(pin) * 0.004882814);
}