Reference no: EM13890560
The second project builds on the first project by adding the ability to specify transformations of some of the graphic objects. The specification for those objects now will require less information about their position and size because both will be able to be modified using the transformations. As before, you must complete the skeleton project provided by defining some of the remaining classes. The UML diagram for the whole project is shown below:
The code for all the classes shown in black is provided in the attached .zip file. You must complete the project by writing those classes shown in red. The skeleton project contains a parser that will parse a scene definition file that defines the scene to be displayed. The new grammar for the scene definition file is shown below:
scene -> SCENE IDENTIFIER number_list graphics END '.'
graphics -> graphic graphics | graphic
graphic -> text | transformable_graphic transformations END
transformable_graphic -> isosceles | parallelogram | regular_polygon
isosceles -> ISOSCELES COLOR number_list ANGLE NUMBER ';'
parallelogram -> PARALLELOGRAM COLOR number_list ANGLE NUMBER ';'
regular_polygon -> REGULAR_POLYGON COLOR number_list SIDES NUMBER RADIUS NUMBER ';'
text -> TEXT COLOR number_list AT number_list STRING ';'
transformations -> transformation transformations | transformation
transformation -> rotation | scaling | translation
rotation -> ROTATE ANGLE NUMBER ';'
scaling -> SCALE number_list ';'
translation -> TRANSLATE number_list ';'
number_list -> '(' numbers ')'
numbers -> NUMBER | NUMBER ',' numbers
Below is a description of the modifications required to each of the five classes from the first project:
1.The Text class is unchanged from the first project.
2.The ConvexPolygon class must now have an additional data element, which is a vector of pointers to Transformations. The constructor must now be passed this vector in addition to what was previously required. The draw function must now perform each of the transformations before drawing the object.
3.The constructor for the IsoscelesTriangle class will now require the list of transformation and the color, but the only information specifying the shape will the angle of the top vertex. The base of the triangle should be centered at the origin and the height of the triangle should be 1. It should perform the transformations using the built in functions of OpenGL, glTranslated, glRotated, and glScaled for translating, rotating and scaling respectively.
4.The constructor for the Parallelogram class will now require the list of transformation and the color, but the only information specifying the shape will be the angle of the lower left vertex. The lower left vertex should be placed at the origin, the top and bottom sides should be parallel to the x-axis and all sizes should have a length of 1. It should perform the transformations using the built in matrix multiplication function of OpenGL, glMultMatrixd.
5.The constructor of the RegularPolygon class will now require the list of transformation and the color, but the only information specifying the shape will be the number of sides. The center of the polygon should be placed at the origin and the radius should be 1. It should perform the transformations using the built in functions of OpenGL, glTranslated, glRotated, and glScaled for translating, rotating and scaling respectively.
In addition to modifying the above classes, you must write the following new classes:
1.The Rotation class must have a member variable containing the angle of rotation, which is passed to and saved by the constructor, so that it can be used to call the rotate method defined in the Transformable interface, when the transform method that it is required to implement, is called.
2.The Translation class must have member variables containing the x and y distances of translation, which are passed to and saved by the constructor, so that they can be used to call the translate method defined in the Transformable interface, when the transform method that it is required to implement, is called.
3.The Scaling class must have member variables containing the x and y scale factors, which are passed to and saved by the constructor, so that they can be used to call the scale method defined in the Transformable interface, when the transform method that it is required to implement, is called.
Sample Input and Output
Below is a sample of a scene definition file that would provide input to the program:
Scene Polygons (500, 500)
Isosceles Color (0.0, 0.0, 1.0) Angle 90;
Rotate Angle 45;
Translate (100.0, 0.0);
Scale (50.0, 50.0);
End
RegularPolygon Color (1.0, 0.0, 0.0) Sides 7;
Translate (-100.0, 0.0);
Scale (75.0, 75.0);
End
Parallelogram Color (0.0, 1.0, 0.0) Angle 45;
Rotate Angle 45;
Translate (100.0, -100.0);
Scale (90.0, 90.0);
End
Text Color(0.0, 0.0, 0.0) at (-200., 200.) "Hello World";
End.
Shown below is the scene that should be produced when the program is provided with the above scene definition.
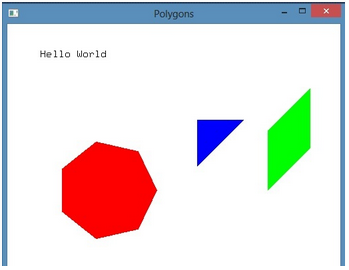
Project Questions
If you have any questions about the this project, post them in the "Ask the Professor" discussion.
Attachment:- Project.zip