Reference no: EM132286003
Assignment - TCP/IP Communications via Socket Programming
1. Introduction
This assignment of TCP/IP communications is a group-of-two or group-of-three assignment worth 15%. Working individually without a group for this assignment is only acceptable with an pre-approval from the unit coordinator. The assignment will require Socket Programming, which is the topic of Week02 Lecture and Week03 Tutorial.
Sections 1 through 3 introduce some background information relevant to the assignment. The assignment tasks are described in Section 4. Section 5 highlights what and where you need to hand in your project report. In Section 6, the marking scheme of the assignment is enclosed for your reference.
This assignment relates to the following unit outcomes described in the unit outline:
2. Skills to undertake planning and design of computer networks to satisfy a set of requirements specifications with particular emphases on connectivity, scalability, reliability, security and QoS; and
4. Advanced collaborative and communication skills through a group project and formal technical report.
The criteria and performance standards used in this assessment are described in a table at the end of this document. Use the table as a marking guide.
You are asked to self-assess your assignment (in the enclosed marking guide table) and reflect on what you have achieved before handing in your assignment report. This enables you to check the completeness of your assignment tasks, and also gives you the opportunity to reflect on your achievements, e.g., what you have learned from this assignment and what you need to improve.
• For the self-assessment, submit the self-assessed sheet together with your assignment. The self-assessment sheet is the table at the end of this document, and is used as a marking guide.
• For reflection, compose a Reflection Section, in which each of the group members writes a separate paragraph of individual reflection.
Key technical aspects that this assignment is addressing include: TCP/IP communications, socket programming. No-technical aspects of the assignment include: team work, communication and report, and reflection.
2. Background: Dealing with Time
There are basically three types of methods to deal with time in C programming:
(1) Use some well-developed timing control APIs. For example, in Windows, a few functions are implemented in windows library (header file: windows.h); and QueryPerformanceCounter() can be used for high-resolution timing control. This is the method we used in our examples, and is recommended to you for this assignment.
(2) Using the standard time library. The standard time library provides a number of functions for time operations, e.g., time(), localtime(), etc. Find a book or search the Internet to learn how to use this time library.
(3) Using hardware timer interrupt, which is the highest hardware interrupt. In this assignment, you do not have to use this method.
For Linux users, sys/time.h declares a few time functions for high-resolution timing control, e.g., gettimeofday().
3. Background: Socket Programming
What is a "network socket"? A socket is a way to talk to other programs using the standard (Unix) file descriptors (Everything is treated as a file in Unix). A file descriptor is simply an integer associated with an open file; and the file can be a network connection!
Where to get this file descriptor for network communication? Call the socket() system routine. Then, send() and recv() and other socket calls can be used to talk to other computers. In this assignment, we deal with TCP socket only although other sockets are also available, e.g., UDP.
4. Assignment Tasks
You are asked to develop a Server program running on one computer, and a Client program running on another computer. (When you test your server and client programs on one computer, you may use Loopback IP Address 127.0.0.1, with which any packets sent out from this machine will immediately loop back to itself.)
The Server will accept input from keyboard for system initialization, selection of menu items, human command and instructions, etc. It will also display information on the monitor, periodically send commands to the Client, and receive feedback from the Client. After receiving a command from the Server, the Client will send feedback to the Server, and displays some information of your interest.
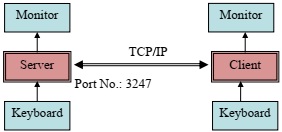
Assignment tasks are described below:
(1) When the Server is started, it initializes the settings of the server's IP address, port number, and the client's IP address, etc., through command window arguments, e.g., header file, arguments to main(), keyboard input, or input from a configuration file which is a pure text file.
(2) Every 3 seconds, the Server sends the Client a command to ask for data, e.g., through a single letter "R" or "r" (request). (Timing control is required here. Using our examples in the lecture materials if you like.)
(3) After receiving the command from the Server, the Client sends back to the Server an ACK consisting of the client's time hh:mm:ss:xxx ("xxx" means three digits, e.g., 251, indicating 251 ms) and also a random integer number between 0 and 100 with a uniform distribution. The Client may also display some useful information on its monitor. It is up to your design on what information the Client displays. (You may use a random generator to generate random numbers. For example, rand()%101; srand(time(NULL)) can give a seed for rand(), where time() is defined in header file time.h).
(4) The Server gets the feedback from the Client, and display the result.
(5) The Server reads keyboard input of various command and instructions. An obvious command is to terminate the Server program, e.g., using a single letter "E" or "e" (exit). When the Sever is to be terminated, the Server should also notify the Client of the Server's termination so that the Client also terminates properly.
How to test your programs:
(1) At early stages of your program development, you may test your programs on a single computer. Execute the Server program in one command window, and execute the Client program in another command window. Both the Server and Client share the same IP address, e.g., the Loopback IP address 127.0.0.1.
(2) If you test your programs in computer labs, you may execute the Server and Client programs on two different computers, which have different IP addresses.