Reference no: EM131931245
Assessment details
1. Objectives
The purpose of this assessment item is to assess your skills attributable to the specified learning outcomes, and achieving the expected graduate attributes of advanced level knowledge, cognitive, technical, and creative skills, and professional level communication and self-management. The learning outcomes are:
- Design and implement appropriate data structures for application development.
- Analyse, develop and implement software solutions with the focus of data structures and algorithms.
- Apply classes, inheritance, polymorphism, and exception handling.
2. Assessment Task
In this assignment, you are required to analyse the given problem, model, and design the required data structures using UML class diagrams. You will be implementing the software solution applying efficient algorithms, inheritance, polymorphism, and exception handling. Your Java Application should have an interactive Graphical User Interface (GUI) based on the JFrame class using SWING GUI components. You should also write a report as specified demonstrating your conceptual knowledge and communication skills.
Problem
A restaurant manager wants you to develop a software application which can be used by customers seated at a table to choose their menu items for a selected meal of breakfast, lunch, or dinner. A table can be occupied by more than one customer and all the customers in one table should be able to choose their options and send it. The concerned staff members such as chef, waiter, and billing staff should be able to use the entered data for their job. To make your job easy, initially you develop a prototype. During the prototype development you have to develop a Java Application that lets the user to choose food and beverage items for a lunch menu and display the nutrition values for the individual items and total for the whole meal ordered.
Modelling and GUI Design
You can use the following guidelines in your modelling and GUI design.
1. First you will design the classes using UML class diagrams and include the attributes with correct data types and all the methods, clearly indicating public or private. This will ease your implementation.
2. A top panel that contains Text fields for text entry, and ComboBoxes (drop down list) for selecting food and beverage. Provide Labels for the Textfields and ComboBoxes.
3. A middle panel that contains a Text area to display the menu choices and nutrition values. Provide a Label to display the Text area.
4. A bottom panel that contains four buttons which are "Enter Data" button, "Display Choices" button, Display Order, "Clear Display" button and "Quit" button.
An example GUI design is shown in Figure 1. You can modify your design to make it more user friendly and add the reasoning for your changes in java doc comments inserted in the source code file.
The functions of the five buttons are described below.
1. Enter Data
The user enters the name and table number through the text fields on the GUI, and selects food and beverage for the lunch menu. The list of food and beverage items and associated nutrient values are given in the data file (.csv) available at the unit website. Your application should load the data when it launches. Once the user clicks the ‘Enter Data' your application should store the entered data in appropriate data structures.
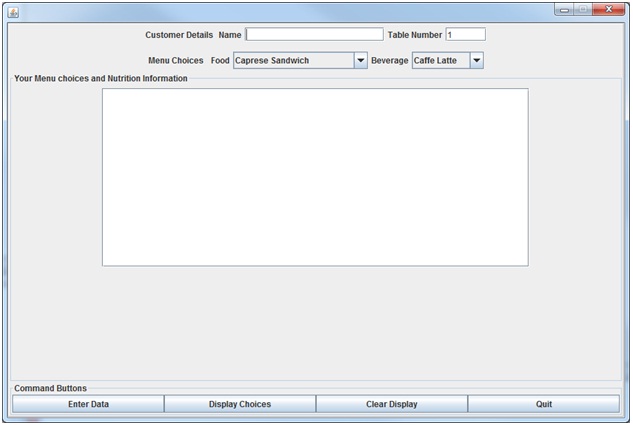
Figure 1. Example GUI Design
The user should enter required data and click on "Enter Data" button. If any of the entries are missing appropriate error message should be displayed.
Note that after "Enter Data" button is clicked, the Name Textfield must be cleared as blank, the TableNumber Textfield should be set to its initial value of ‘1'and the two ComboBoxes must be set back to their default statuses.
2. Display Choices
Clicking on the "Display Choices" button should display the selected menu choices, including nutrition values for each of the nutrition information, and the total nutrition information for the whole meal. As given in the data file, the nutrition values to be displayed are Energy, Protein, Carbohydrate, Total Fat, and Fibre. The total of each nutrition value is calculated by adding the corresponding nutrition value for each item.
3. Display Order
This is for displaying the Customer names and items ordered for a particular table. The user should enter the Table Number before clicking the ‘Display Order' button.
4. Clear Display
Clicking on the "Clear Display" button should clear all contents from the display area and set the Textfields and ComboBoxes to their default values.
5. Quit
The "Quit" button should allow the user to exit from the application.
Data Structures.
You can use the classes given below as a guideline for your design.
1. Customer class
a) Include appropriate fields to store:
Customer's first name: Maximum width 20 characters.
Selected table number: It should be an integer value between 1and 8 with a default value of 1. Raise an exception for wrong entry and allow the user to re- enter the value for 3 times.
b) A constructor and necessary accessor and mutator methods to get and set values.
c) A toString() method that displays the customer details.
2. Nutrient class
a) This class should have the fields to store the nutrient name and quantity of nutrient. Complete the class with necessary constructor, mutator, accessor methods and a toString() method.
3. MenuItem Class
a) This class should have the fields to store:
MenuItem name and an ArrayList of nutrient objects to store the nutrient details.
b) It should also include constructor, mutator, and accessor methods.
c) A toString() method for displaying item details.
d) This class also should have a copy constructor to allocate memory for the ArrayList when the assignment of method calls with parameter passing and assignment of one object to another using the assignment statement are required.
4. OrderedCustomer class
a) This class extends the Customer class. This class should have a member field to store the list of menu items chosen by the customer by clicking the "Enter Data" button.
This class also should have:
b) Constructor, accessor and mutator methods.
c) A toString() method for displaying the OrderedCustomer details.
d) A method to calculate the total of each of the nutrition values. Use polymorphism while using objects of this class.
5. RestaurantOrderGUI class
a) This class should have the GUI components listed above.
b) It should have the methods to set up the GUI components and the event handling methods.
c) This will also contain the main method.
Software Tools for Building the Application
You can build your application using the TextPad Editor or NetBeans. You should create the GUI components using code rather than 'designer' and 'click and drag' of the NetBeans. This creates code which is not maintainable. Remember this is a good prototyping tool, but not recommended for coding.
Note: Commence with one class at a time, test it and then incrementally add the next.
Report
You should write a report which includes a front page with your student details, and the UML class diagrams, and screen shots of execution of your program to demonstrate that you have tested the program. Provide any additional information you want to include, for example reasoning for your particular choice of screen design.