Reference no: EM131373548
General Instructions
- Your HTML files must be validated by the W3C validator at https://validator.w3.org
- You must test your web pages in Google Chrome to make sure they work. You should test them in another browser, but this is not a requirement.
- Save all your files (HTML, CSS, images) to your /practicals/prac2 folder on your TWA website.
General Workflow
PHP does not work like HTML or Javascript. You have to upload your files to your TWA site or edit within your TWA site for testing. This means you edit -> upload -> test-> edit -> upload -> test ...
To speed up this process you may want to develop locally using a local installation of the software Apache/MySQL/PHP, such as UwAMP (https://www.uwamp.com/en/), however configuration and/or technical support on how to get it to work will not be provided by your tutor. If you choose to do this, do not substitute it for your actual TWA site for testing. If your site works locally, but does not work on your TWA site, that is not a reason for special consideration when marking. How your work works on your TWA site will be assessed.
PHP Form Processing
Task 1
Within your practical-2.zip after extraction under the task-1 folder you will have the file task1.php. This file contains a HTML form with various inputs. Your task is to echo out the values passed by the form to itself (task1.php) via a GET request. What you are doing is called a callback. You are to display values within the provided section of the task1.php page:
To get full marks in this task the password must be hidden with the use of ‘#'. There is to be the same amount of ‘#' as characters in the password. For example:
Password = ########
Task 2
Within your practical-2.zip under the task-2 folder you have been given a file called task2.php. This file contains a HTML form with various inputs. Your task is to echo out the values passed by the form to itself (task2.php) via a POST request. What you are doing is called a postback. You are to display values within the provided section of the taks2.php page:
Note: notice the name of the checkboxes? sports[] this is to tell PHP to build an array with the data sent within the request for the parameter sports
Primitive Booking System
You will be building a primitive car booking system. This booking system uses a database to store all its information. Once you've completed you will be able to login and add new bookings and list all the bookings for the various cars available.
When working on these tasks all files are to be within the folder task-3-10.
Simple styling is applied to the initial form in task 3, this styling is the level of styling that is required for all your forms. Although the styling may be basic, the structure of your HTML documents should still be well thought out and formed.
Database Operations
The database schema is as follows:
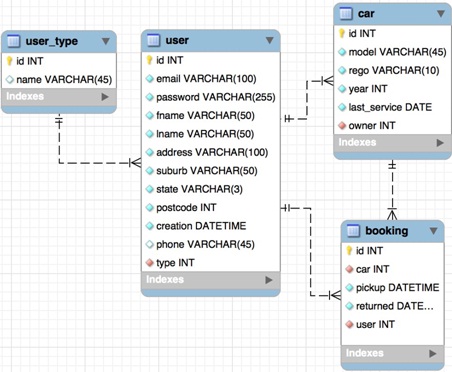
Connecting to the database
You have your own unique database. To log into your database you have to use a username and password. Your database name for Practical 2 is prac2_### with the ### being your TWA site number. For your username, it is your TWA username, however your password is twa###XX. The ### being your TWA site number and
the XX being the first two (2) characters of your TWA site password. For instance, your TWA site is twa999, and your password is abcd7890, this would mean your password would be twa999ab.
For these tasks you are to use the PHP mysqli extension - that is the MySQL Improved, do not confuse it with the general MySQL extension which is deprecated and should not be used! For reference to the mysqli extension and for all its capabilities please refer to https://php.net/manual/en/book.mysqli.php
Here is the sample code to connect to your database (using example user):
Listing data within your database
So you can see what is in the database there is a file in the task-3-10 folder called called listdata.php. This file, after you have placed your database username, password, and database name in the connection string, will list out all the data found within the database. This will allow you to see if you have successfully inserted data into the correct table and in the correct format.
Task 3
Within the task-3-10 you will have a file called adduser.php. This file already has a partial form created for one of the tables (user). Your task is to use this form to insert new users into the database table via PHP.
Be aware the type of user is a dropdown box and it is empty. It is your task to dynamically populate this field via PHP to insert the different types of users available from the user_type table. This drop down box cannot be hard coded as a foreign key to the user_type table is present.
As the page contains no validation initially, you are to provide validation through PHP. This means you will need to handle incorrect data (incorrect type, etc.). There are no specific rules on validation, for instance, the phone number needs to be 9 characters long and contain only numbers, but the form cannot fail if rogue data is entered. To ensure this, it would be best to refer to the database schema for the variable types and lengths needed for the available table fields. If the form is invalid you must present notifications to the user in line with the form element, similar to the error messages for practical set 1, but presented by PHP not Javascript.
You will notice when looking at the previous data that a user's password is very long. This is because it is hashed. Passwords should never be stored as plain text. Therefore, you will have to hash passwords. To do this you pass a string through a hash algorithm. This hashing is important, as it will set up other tasks you will complete. Here is a basic example on how to hash a string:
<?php
// A stored password
$password = 'password';
// PHP Hashing Method
$password = hash('sha256', $password);
echo $password;
// Prints out:
// 5e884898da28047151d0e56f8dc6292773603d0d6aabbdd62a11ef721d1542d8
?>
The following is an example of how to insert a record for the user_table into the database via PHP. Remember for this task you are not working with the user_table, you are working with the user table! Seeing how it can be done for the user_type table might help you understand how you would do it for the user table.
<?php
// We establish a connection to the database
$connection = new mysqli('localhost', 'root', 'password', 'prac2');
if($connection->connect_error) {
echo "Failed to connect to MySQL" . $connection->connect_error;
}
// Before inserting we should double check everything exists from the form
// The way you do this is up to you
if(isset($_POST['name'])) {
// We sanitise any input to prevent SQL injection
$name = $connection->real_escape_string($_POST['name']);
// We use an INSERT query to insert our data into the database
$result = $connection->query("INSERT INTO user_type (name) VALUES('$name')");
} else {
// Display error message maybe?
}
// Was the insertion successful?
if(!$result) {
echo $connection->error;
}
?>
Task 4
Create the forms for the remaining tables. You can use the format and style of the previous form. As you have created the adduser.php file, it means you have to create 3 more usertype.php, car.php, booking.php.
Hint: You can use a lot of the logic from task 3.
Task 5
As you now have multiple files, create a simple navigation menu somewhere on your pages so a user can navigate between each file.
Task 6
Create a new file called carbookings.php. You are to create a dropdown box of the available cars and when the dropdown box changes the page is updated showing a table of all the bookings for the selected car. It is to be when the dropbox changes not when a button is clicked, therefore there is no submit button on this page. The table is to have headers for each column which include the user's full name, car rego, pickup date time, expected return date time.
Task 7
Not everyone should have access to your site, therefore create a new file called index.php. This file will be your entry page to your booking system. It will welcome a user and have a login form that will consist of a username input, password input, and a login button. The username and password entered must match the username and password in the database. If a user fails to login they are to be presented with an error message regarding their incorrect login. Once matched they will be redirected to the carbookings.php file. For the existing users in the database their username and passwords are as follows:
[email protected] |password1 [email protected] | password2 [email protected] | password3 [email protected] | password4
Hint: This login needs to hash the entered password so that it can be compared to the hashed password in the database
Task 8
For each user listed when a car is selected within your carbookings.php page turn their name into a hyperlink so when clicked will go to the page userlisting.php showing the details of the user clicked. You can style the user details however you like, but they must be styled so they look user friendly.
Task 9
With the userlisting.php page you are to create a table giving a history of all the bookings a user has made. This table is to be presented below the user details and include the model, rego, year, booking pickup and return.
Task 10
A user cannot have access to any page, except index.php, without being logged in. If they arrive at a page and they have not logged in they will be redirected to the index.php page. For this you are to use a PHP session which will be created once they have successfully logged in. The session will be created in the index.php file and basic checking if the user is logged in should be present in all files to ensure the user is logged before they can access a page.
Attachment:- practical-2-files-rev1.zip