Reference no: EM131304755
Introduction to Signal Analysis Project: Music Synthesis
Project Definition: The objective of this project is to synthesize musical signals. You will generate harmonic tones and use them to perform well-known melodies. Moreover, you will use the concept of FIR filtering to add reverb, a popular sound effect, to musical signals.
A. Computing the fundamental frequency
The relationship between a Piano key number and the fundamental frequency of the played note is given by:
f0 = 440 · 2(K-49)/12,
where f0 denotes the fundamental frequency and K the Piano key number.
Write a function named keynum2freq, which receives a Piano key number, K (a non-negative integer number), and returns the corresponding fundamental frequency, f0. Note that the Piano has 88 keys in total (key numbers range from 1 to 88); we will also include 0 in the range of K values to represent silence in a melody line.
B. Generating Harmonic Tones
A harmonic signal is basically the sum of a number of sinusoids, whose frequencies are multiples of a fundamental frequency, i.e.
X(t) = h=1∑H Ahcos(2πfht),
where Ah is the amplitude, and fh = h · f0 the frequency of the hth harmonic.
Write a function named harmonic_signal, which receives the fundamental frequency, f0 (a real and positive number), the number of harmonics, Nh (a positive integer number), harmonic amplitudes, Ah (a vector of length Nh with elements greater than zero), the total duration of the harmonic signal, dur (a real and positive number), and the sampling rate, fs (a positive and integer number). The function should return a vector containing samples of the harmonic signal, X(t), over the specified duration. The output vector should be normalized so that its maximum value is equal to one.
C. Generating Time-Domain Envelopes
To improve the quality of the sound, we can multiply the harmonic signal by an envelope, E(t), so that it would fade in and out. A standard way to define the envelope function is to divide E(t) into four sections: attack (A), decay (D), sustain (S), and release (R), abbreviated as ADSR. The following figure shows a linear approximation to the ADSR envelope:
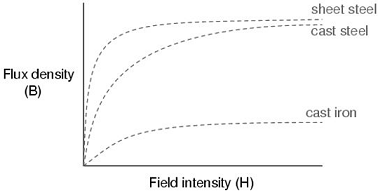
Write a function named adsr, which receives the following input variables:
- Adur: ratio of attack time to the total envelope duration (real, between zero and one)
- Ddur: ratio of decay time to the total envelope duration (real, between zero and one)
- Sdur: ratio of sustain time to the total envelope duration (real, between zero and one)
- Rdur: ratio of release time to the total envelope duration (real, between zero and one)
- Damp: envelope amplitude at the end of decay time (real, between zero and one)
- Samp: envelope amplitude at the end of sustain time (real, between zero and one)
- dur: total duration of the envelope (real and positive)
- fs: sampling rate (integer and positive)
Note that the sum of Adur, Ddur, Sdur, and Rdur, should be equal to one. Also, note that we are generating a normalized envelope (the maximum value of the envelope is equal to one, i.e. Aamp = 1). The function should return a vector containing samples of the envelope, E(t), over the specified duration.
D. Generating Musical Notes
Write a function named note which receives the Piano key number, K (integer, between 0 and 88), the intensity of tone or sound volume, vol (real, positive), the duration of the note, dur (real and positive), and the sampling rate, fs (integer and positive) and returns a vector containing samples of the synthesized tone. Functions keynum2freq, harmonic_signal, and adsr should be called to calculate the fundamental frequency of the note, harmonics, and the time-domain envelope respectively. The musical tone can be computed from the following relationship:
X(t) = vol ·E(t)h=1∑HAh cos(2πfht).
Note that if K = 0, the function should return a vector of zeros (silence) over the specified duration.
E. Synthesis of Melody Lines
Write a function named melody, which receives a vector of Piano key numbers, Keynums, a vector of note/silence durations, Durs, a vector of tone intensities (sound volumes), Vols, and the sampling rate, fs. The function should call the note function for each key number and corresponding tone properties and put the outputs back to back to generate a longer signal (Hint: you can use a for loop that calls the note function for one key number in each iteration). This longer signal is composed of a series of notes and silences, in other words it forms a melody.
F. Playing Tunes You Know Well!
Download the file Tune.mat from Blackboard and place it in the same folder as your functions. Open a new MFile. Load the content of the file to the workspace. You should be able to see six variables named, Gk, Gdur, Gvol, Fk, Fdur, and Fvol in the workspace. The first three are parameters of one melody line and the rest parameters of another. Call the function melody in each case and save the outputs in variables named G and F (set the sampling rate to at least 20000 Hz). Generate another variable named GF which is the sum of G and F. Call the MATLAB function sound with inputs GF and fs to play the music signal. Does it sound familiar? Feel free to test your code with your favorite melodies.
G. Adding Sound Effects to the Musical Signal
In this part we will add reverb to our musical signal, which is equivalent to FIR filtering of the signal. The reverb effect can be created by adding delayed versions of the sound signal to the original version. Each one of the delayed versions represents a reflection of the signal from some surface. Altogether, it gives a feeling of space, e.g. the contrast between being in a small room or in a concert hall.
Write a function named reverb, which receives the musical signal, X, the number of reverbs, Nr (integer and positive), a vector containing delay times for different reflections, Dr, a vector containing attenuation rate for different reflections, Ar, and the sampling rate, fs. Note that the input delays (elements of Dr) are measured in seconds, therefore the function should first convert them to number of delayed samples. The output is basically the sum of delayed versions of the input. This function is quite similar to the diff_ eq function you worked with in Lab 8.
Call the reverb function in the script you created in the previous part for the GF sound signal. Again convert the signal to audio and listen to it. Do you notice any difference in the sound quality?
H. Parameter Setting
Here is a list of suggested parameter values for testing your code:
Harmonics: Nh = 5, Ah = [ 1 0.8 0.6 0.4 0.2 ]
Envelope: Adur = 0.05, Ddur = 0.1, Sdur = 0.6, Rdur = 0.25, Damp = 0.6, Samp = 0.4
Reverb: Nr = 3, Dr = [ 0.2 0.3 0.4 ], Ar = [ 0.4 0.1 0.05 ]
Feel free to tweak parameter values and synthesize a variety of sound colors.
Show the execution of your code to the instructor during the session designated for project submission. Prepare a report of your work and submit it on Blackboard. Your report should contain all the code you develop as well as a brief explanation of how it works (no more than one page). Also, include a conclusion section in your report explaining what you have learnt from this project (no more than one paragraph).