Reference no: EM131491896
Assignment
Java RMI (Remote Method Invocation, reference Chapter 5 of the textbook and Week-3 lecture) enables the local invocation and remote invocation use the same syntax to implement generic remote servers like the Compute Engine example in Week-3 lecture slides. However, Java RMI needs 2 HTTP servers to transfer Java classes between a RMI client and a RMI server at runtime. In addition, Java RMI applications need a RMI Registry for registering or looking up the remote objects.
In this assignment (assignment-2), you are to implement a remote invocation framework that is similar to Java RMI but lightweight (note: for this assignment, you don't use any Java RMI APIs). To implement the framework, you will need to use Java Interface, Java Objection Serialization, Java Thread and client/server model. Recall you have created some Java examples about these Java components and models for assignment-1; now you will need to use these Java components as a reference to build this framework. Thus if necessary, you may need to review your assignment-1.\
This assignment specification is as follows.
Part 1: Java TCP Networking, Multi-threading and Object Serialization Programming
The framework consists of a compute-server, a compute-client and a codebase repository, which are depicted in the following diagram. The framework is a generic computing architecture because the compute-client and compute-server just need to know the Task and CS Message components in advance before the framework can start to run. The specification of the components is as follows.
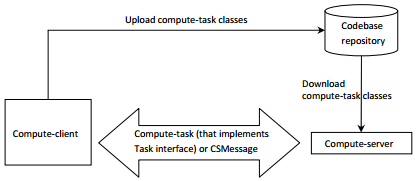
1. The interaction contract
The interaction contract between the client and server is defined by the Task interface:
//The Task interface (interaction contract) between clients and the server
public interface Task {
public void executeTask();
public Object getResult();}
Every compute-task must implement the Task interface. Executing the executeTask() method will perform the task and set the result. Calling the getResult() method will return the result.
2. The compute-client and compute-server
The compute-server is used as a generic compute-engine. While running, the server is continuously waiting for the compute-tasks. A compute-task is created by a compute-client and sent as a serialized object to the compute-server. Once the compute-server receives a task, it will cast (deserialize) it into the Task interface type and call its executeTask() method. After executing the task, the compute-server will send the same object back to the computeclient.
The compute-client is continuously accepting a user's requests. Every request specifies a compute-problem and its corresponding parameters. For a request, the compute-client creates a compute-task and sends it as a serialized object to the compute-server. Once receiving the compute-task object back from the server, the compute-client will call the getResult() method of the object to display the result.
3. The codebase repository
Such a framework makes the compute-server generic. That is, the compute-server just needs to know the Task interface, then it can be compiled and run. If a compute-client implements a new compute-task after the server is run up, the compute-client just needs in some way (in real world application it could be a FTP server, but in this assignment, you just need to copy the files into a directory) to upload the Java class of the compute-task into a pre-determined network location (e.g. the codebase directory), which the compute-server can access from its Java classpath. Then the compute-server can perform such a new compute-task. Therefore, the server never needs to be shut down, recompiled, and restarted.
4. The error message
However, when there is an exception occurred (e.g. a compute-client wants the compute-server to perform a compute-task, but forgets uploading the Java class of the compute-task) onto the codebase of compute-server, the compute-server will create a CSMessage object and sends it back to the compute-client. Note: the CSMessage follows the interaction contract by implementing the Task interface. By calling the getResult() method, the compute-client will know the problem and fix it later on.
To implement the framework, you need to implement the following Java classes:
1. A Java application to implement the compute-client;
2. A Java application to implement the compute-server; and
3. A Java class to implement the request processing thread.
4. A number of Java classes to implement Calculate Pi, Calculate Primes and Calculate the Greatest Common Divisor tasks.
Part 2: Program use and test instruction
After the implementation of the framework, prepare an end user instruction about how to use your software.
Attachment:- Assignment Files.rar