Reference no: EM13317779
Part A: Multiple Choice
Circle the *most appropriate answer (i.e. only select one answer):
Part A Question 1. Which one of the following is true about a class in java?
a) It can extend multiple classes, and implement many interfaces.
b) It can extend only one class, but implement many interfaces.
c) It can extend multiple classes, but only implement one interface.
d) It can extend only one class and implement only one interface.
Part A Question 2. Assume that there is an abstract class called Vehicle, which has two concrete subclasses, Car and Truck. There is also an interface Loadable, which only Truck implements. Which statement below will cause a compilation error?
a) Loadable item = new Truck();
b) Vehicle item = new Car();
c) Vehicle item = new Truck();
d) Vehicle item = new Vehicle();
Part A Question 3. If two algorithms A and B perform the same function and algorithm A has performance O(log n) and algorithm B has performance O(n), which one of the following is true?
a) Algorithm A has better performance scalability than algorithm B
b) Algorithm B has better performance scalability than algorithm A
c) Algorithm A uses less memory than algorithm B
d) Algorithm B uses less memory than algorithm A
e) None of the above
Part A Question 4. Which one statement is true about the code fragment listed below?
public class Mylistener extends WindowAdapter
{
public void windowClosed (WindowEvent e)
{
System.exit (0);
}
}
a) This code compiles without error but could not be used as a window listener because it does not implement all WindowAdaptor methods.
b) The code will not compile correctly, because the class does not provide all the methods of the WindowListener interface.
c) The code compiles without error and the words extends WindowAdapter could be changed to implements WindowListener without changing the behaviour.
d) The code compiles without error and will do nothing if a windowClosing event is generated.
e) The code compiles without error and will throw an exception if a windowClosing event is generated.
Part A Question 5. Which one of the following is true about an abstract class in Java?
a) It cannot implement interfaces.
b) It cannot be instantiated.
c) It is not allowed to have constructors.
d) All of the above are true.
Part A Question 6. Which of the following statements about interfaces in Java is false?
a) You can extend an interface.
b) You can implement multiple interfaces.
c) Interfaces can have abstract methods.
d) Interface can have member variables.
Part A Question 7. Consider the following class hierarchy and code fragment:
Java.lang.Exception
|
Java.io.exception
/ \
Java.io.StreamCorruptedException java.net.MalformedURLException
1. try {
2. URL u = new URL(https://www.rmit.edu.au);
3. int ch = System.in.read();
4. System.out.print("char read");
5. }
6. catch (MalformedURLException e) {
7. System.out.print("MalformedURLException,");
8. }
9. catch (ObjectStreamException e) {
10. System.out.print("ObjectStreamException,");
11. }
12. catch (Exception e) {
13. System.out.print("general exception,");
14. }
15. finally {
16. System.out.print("executing finally block,");
17. }
18. System.out.print("continuing");
What will be output if the code at line 3 throws an IOException?
a) char read
b) general exception, continuing
c) MalformedURLException, OjectStreamException,general exception, executing finally block, continuing
d) char read, general exception, executing finally block, continuing
e) general exception, executing finally block, continuing
Part A Question 8. Consider again the class hierarchy and code fragments in Question 7. What will be output if the code at line 2 throws a MalformedURLException?
a) general exception, executing finally block, continuing
b) MalformedURLException, ObjectStreamException, general exception, executing finally block, continuing
c) MalformedURLException, general exception, executing finally block, continuing
d) MalformedURLException, executing finally block, continuing
e) MalformedURLException, general exception, continuing
Part A Question 9. Consider the hierarchy (note that the abstract classes are indicated by italic font):
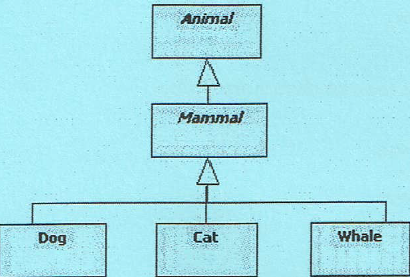
Consider the following code:
1. Dog rover, fido;
2. Animal animal;
3.
4. rover = new Dog();
5. animal = rover;
6. fido = (Dog)animal;
Which one of the statement below is true?
a) Line 5 will not compile.
b) Line 6 will not compile.
c) The code will compile but throw an exception at line 6
d) The code will compile and run
e) The code will compile and run but the cast in line 6 is not required and can be eliminated.
Part A Question 10. The following code relates to the hierarchy in question 9.
1. Dog rover, fido;
2. Animal animal;
3.
4. animal = new Animal();
5. rover = (Dog)animal;
Which one of the statements below is true?
a) Line 4 will not compile.
b) The code will compile but throw an exception at line 5
c) The code will compile and run
d) The code will compile and run but the cast in line 5 is not required and can be eliminated.
Part B: Java Programming
Part B Question 1. Consider the class diagram below. Presently, neither a class hierarchy nor polymorphism is used.
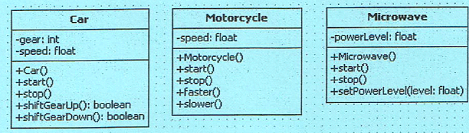
a) Identify common functionality (e.g. methods and variables) and place it in an abstract superclass called AbstractRunnable which has an additional instance variable boolean isRunning which is set appropriately.
b) Modify the Microwave class to use the superclass (from part a) making sure to include all necessary variables/methods. The constructor should set the powerLevel to 100.0 by default and the start() and stop() methods should be overridden to call the appropriate superclass method, with the remainder of the method body left empty with //TODO comment.
NOTE: The answer must be written using the actual Java code.
Part B Question 2. Consider the following interface, which is designed to calculate a rental fee for different types of borrowable media (e.g. books, videos, dvds)
Public interface Rentable
{
Public double getRentalFee();
}
a) Write an abstract class called AbstractMedia that implements Rentable to provide a predefined fee based on a constant called BASE_RENTAL_FEE which is set to $5.
b) Write a concrete subclass DVD that provides a concrete representation of the AbstractMedia class but does not provide any additional functionality.
c) Write another subclass DVDSeries that extends DVD and takes an int constructor parameter numDiscs (number of dvds in the series). This class should override getRentalFee() based on the default fee plus $1 for each dis in the series. For example, a dvd series with 5 discs would incur a rental fee of $10.
d) Write a declaration for a variable starwars of type DVDSeries which has 3 discs. You should use the most abstract static type.
Part B Question 3. Show the steps to insert the following numbers (one at a time) into a binary search tree. Show the complete tree at each stage (after each number is inserted):
Data: 32,12,21,1,47,37,24,9,66
Part B Question 4. Show the result of performing a pre-order traversal on the final tree above (you only need to show the final order of visited elements).
Part B Question 5. Draw the layout produced by the following code i.e Using the JFrame below, draw all of its contained components paying attention to size and position
Part C: GUI Programming
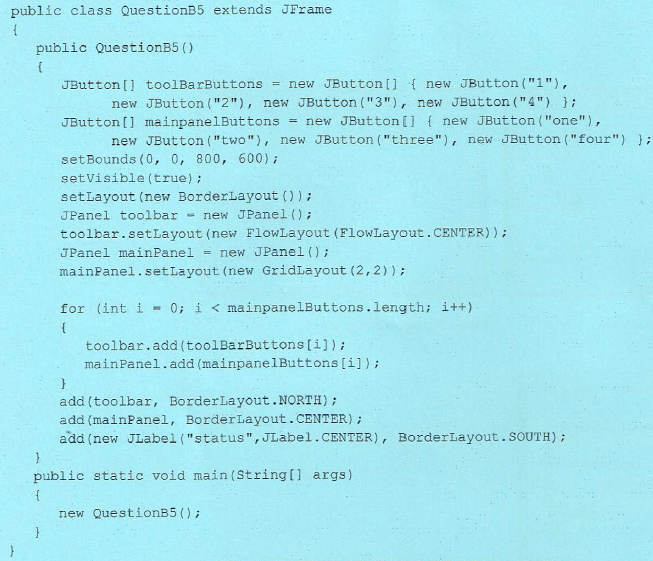
Implement a GUI as shown below:
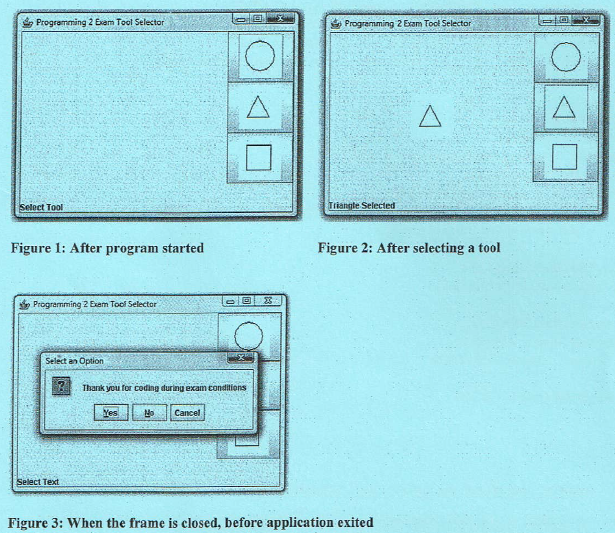
Note the following requirements:
• The title of the frame is "Programming 2 Exam Tool Selector".
• The frame has size of 320x200 pixels.
• There is a status message at the bottom that shows "Select Tool" (see figure 1).
• There are three buttons, equally sized.
• The buttons have an image but no text. The filenames of the images are "circle.png", "rect.png", "triangle.png". You may assume these files are found in the source directory.
• When a tool is selected the tool icon is displayed in the center of the main panel and the status bar message is updated to reflect the selected tool (see figure 2).
• When the frame is closed, a window pops up with the text "Thank you for coding during exam conditions" (see figure 3).
• When the dialog box is dismissed, the application closes.
Implementation:
• Your program must use WindowListener to handle the exit event.
• You may use anonymous Inner classes to implement your controllers.
• Since there is no real model functionality, MVC need not be used. However, you should use appropriate classes and packages to separate the various UI components but can use package scope fields to simplify object referencing.
• You should show all code but do not need to show imports. Where you are not sure of exact Java API syntax, you should provide comments describing which method you are trying to invoke.