Reference no: EM131231
Lab-1
The goal of this lab is to better familiarize you with polymorphism and the factory design pattern, two key components in Assignment. To that end, you will construct a simple "Device Maker." The device will be made from a list of widgets in a text file that will be user-specified. You will need to create a widget factory to convert the name of the widget into the actual widget.
UML Diagrams
Let's start with the Widget and its derived classes:


Note that Widget is declared as an interface. This means that all of Widget's class methods are pure virtual. Pure virtual methods do not have method bodies. Instead, we place the keyword "virtual" before the return type and set them to equal zero (yes, this makes little sense, but that's how C++ does it). For example, the getPrice method would look like:
virtual double getPrice() const = 0;
Defining methods as pure virtual tells C++ that the class' children will be responsible for actually implementing any real functionality. Note that Widget's children (Gear, Cog, Actuator, and Spring) add no additional functionality to Widget. Instead, they simply define the functionality as required by Widget. To that end, each getName() should just return the Widget's name (e.g. "Gear"). Prices for each widget are as follows:
- Gear: $1.25
- Cog: $1.35
- Actuator: $3.50
- Spring: $0.99
Device Class
Let's next look at the Device class:
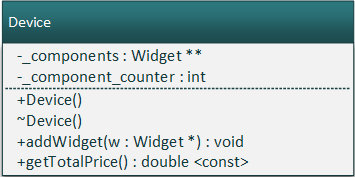
I'm using _component_counter to track the number of valid Widgets inside _components. Also, Device revisits some advanced concepts that you might've forgotten about. Note that _components is a Widget **. This means that _components is an array of pointers to Widgets (hence the double *). You will need to initialize this variable inside your constructor using the following snippet:
_components = new Widget*[100];
This tells C++ to dynamically create _components as an array of 100 Widget pointers. Because we've dynamically created an array of pointers, we finally get to create a useful destructor! In your destructor, you will need to use the delete keyword to delete your array of pointers:
delete[] _components;
Note that we use array syntax "[]" to tell C++ that we want to delete an array of dynamic pointers rather than just a single dynamic pointer.
Widget Factory
Finally, let's examine our Widget Factory:
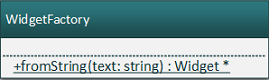
Our factory has a single static method that returns a pointer to a dynamically created widget. To do so, you will need to examine the supplied text (it should contain the widget's name) and convert to the appropriate widget. In the case that an unrecognized name was supplied, simply return a NULL pointer.