Problem Definition
A new Met Office web application will allow users of their web site to view rainfall statistics for months and years in the UK. The application allows the mean rainfall for a given year to be calculated, as well as the mean rainfall for a given month over all available years.
The Met Office data for rainfall is kept in a text file with each line in the file containing a year number, followed by 12 values representing the mean rainfall for each month in that year.
For example, the following extract from the file shows the data for the years 1914 through to 1916:
1914, 50.9, 87, 115.8, 32.3, 47.1, 56.6, 97.1, 63.9, 48.1, 62.4, 110.3, 190.8
1915, 105.3, 129, 36.2, 37.3, 64.3, 33.3, 123, 76.2, 39.4, 71.4, 73.5, 182.7
1916, 58.6, 129.4, 97.9, 47.9, 70.7, 63.7, 51.3, 89.2, 54.8, 142.1, 110.1, 90.4
The file contains mean monthly rainfall data for the years 1914 to 2008 inclusive (Met Office 2009).
You have been asked to define two classes and an enumeration to support the reading in and manipulation of this data, as shown in the following class diagram:
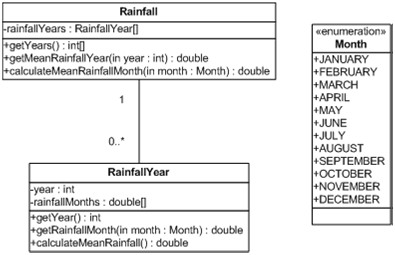
Figure: Assignment 3 Class Diagram
The Month enumeration provides an easy way for a given month to be specified using an appropriate label. This is used in retrieving rainfall data for different months.
The RainfallYear class is used to store the mean monthly rainfall data for just one year (using an array of doubles). As well as having a getter for the year, this class also has a method which will allow the mean rainfall for a month to be returned when a month enumeration value is specified. A method for calculating the mean rainfall over the whole year is also provided.
The Rainfall class is used to store the rainfall data for each available year (using an array of RainfallYear objects). As well as a method which will return an array of the available years, this class also provides methods to return the mean rainfall for a given year, as well as a method which will calculate the mean rainfall for just a particular month over all available years.
When a Rainfall object is constructed, it will automatically read in the available rainfall data from a specified file ("rain.txt"). As the file is read, each value should be checked to ensure that it is a valid integer (for the year) or a valid double (for the monthly rainfall values), with the year value incrementally increasing.
Note that the class diagram does not specify the private methods you might like to include to parse the rainfall file.
Your task is therefore to:
Define the two required classes and one enumeration, including the private methods necessary to parse the rainfall file.
Write a set of RainfallYearTest JUnit tests.
Write a set of RainfallTest JUnit tests.
3. Create a New Java Project in Eclipse
Open up a workspace in Eclipse: you can download a workspace from ULearn with the code formatting styles already defined (from the Labs folder).
Create a project with the name com1017_username_cw3, where username is replaced with your own username.
Add a "test" source folder to your project (in addition to "src" folder).
Create a package in your "src" folder called org.com1017.cw3.username where username is replaced with your own username.
Create a package in your "test" folder called org.com1017.cw3.username where username is replaced with your own username.
If you need further details on how to do this, refer to the Assignment 1 sheet.
4. Defining the Month Enumeration (10%)
Look at the class diagram in Figure 1.
Define a new Java enumeration (within the package you have created) called "Month".
Within the enumeration, specify the required range of values for the months.
1. Defining the RainfallYear Class (30%)
Look at the class diagram in Figure.
Define a new Java class (within the package you have created) called "RainfallYear".
Add the fields to the class definition.
Add the getter to the class definition.
Add the "getRainfallMonth" method to the class. Note that this is not a normal getter as it must be able to take the Month enumeration type and convert this to return the mean rainfall for the correct month.
Add the "calculateMeanRainfall" method to the class. This should calculate the mean rainfall over all 12 months of the year.
Define a parameterised constructor for the class that allows the year to be specified as an integer, and the 12 values for the monthly rainfall as a double array.
Defining the Rainfall Class (30%)
Look at the class diagram in Figure.
Define a new Java class (within the package you have created) called "Rainfall".
Add the field to the class definition.
Add the "getYears" method to the class. Note that this is not a normal getter as it must be able to extract all the available years that are stored and return these as an integer array.
Add the "getMeanRainfallYear" method to the class. Note that this is not a normal getter as it must be able to extract the mean yearly rainfall for the specified year.
Add the "calculateMeanRainfallMonth" method to the class. This needs to extract out the mean monthly rainfall for the given month for each available year to calculate the mean.
Define any private methods you might use to parse the rainfall file. These methods should parse each line of the file to create the associated "RainfallYear" objects. As the file is read, you should check that each value read in is a valid value of the type required (integer for year and double for rainfall), and that the years increase sequentially
Define a parameterised constructor for the class which:
o Takes as a parameter the file name to be parsed.
o Parses the file to create the array of "RainfallYear" objects.
Hints: you might like to create a method that counts the number of lines in a file so that you can tell in advance of parsing the rainfall file how many entries there are. Useful Java classes for reading files are FileReader and BufferedReader. Our lab classes will also focus on file reading to help you understand how to achieve this.
Testing Your Code (30%)
You need to write your own unit tests for your classes which you should put in your "test" source folder within the package you have created.
Create a JUnit test for RainfallYear which has two tests that:
o Tests the construction of a RainfallYear object by creating an object with appropriate values for the year and monthly rainfall data.
o Tests the calculation of the mean rainfall.
Create a JUnit test for Rainfall which has three tests that:
o Tests the construction by checking that all the years have been loaded correctly from the file.
o Tests the calculation of the mean rainfall for a particular year. For reference, the mean rainfall for 1914 is 80.19.
o Tests the calculation of the mean rainfall for a particular month over all the available years of data. For reference, the mean rainfall for January over all years is 90.75.
1 Define an AllTests test suite which will run both the RainfallYear and Rainfall tests.
Hint: a help sheet is available on ULearn which shows you have to create an empty JUnit 4 test.