Reference no: EM13791
1. Object Oriented Programming: Class hierarchies, inheritance and virtual functions
In this part of the assignment you are asked to develop a class structure that can be used to represent closed curves in a two-dimensional plane. Examples of such curves are rectangles, ellipses, squares, triangles, general polygons, etc. To limit the scope of the assignment, we will focus on four special types of curves: ellipses, circles, rectangles and squares. Moreover, we will only work with curves with axes that are parallel to the axes of the coordinate system. In the figure below, you see examples of these objects, as well as example of an object that is not allowed, since it is not parallel to the coordinate axis.
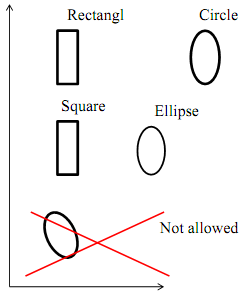
Each object has a position, which can be represented by a coordinate in the (x,y) plane. For example, the place of a circle can be represented by the coordinate of its center. The place of a square can, e.g., be represented by the coordinate of its lower left corner. Further, each object has a size. The size of a circle can be represented by its radius, whereas two numbers are needed to represent the size of a rectangle - its lengths in the x and y direction respectively.
Moreover, there are several common fundamental properties that any closed curve has, e.g., an area and a circumference (i.e., the length of the curve).
It also has a parametric representation, which can be defined as a (non-unique) function p, which takes a number between 0 and 1 and
returns a coordinate, such that the whole curve can be "drawn" by plotting all p(a) for all a between 0 and 1. For example, a circle with center at (x,y) and radius r has the parametric representation p(a) = (x + rx*(2Πa),y + ry*(2Πa).
a) Define classes for a coordinate and for generic closed curves. You may use the following declarations if you wish
class coordinate
{
public:
coordinate() {}
coordinate(double xin,double yin) {x=xin;y=yin;}
double x;
double y;
};
class closedcurve
{
public:
closedcurve();
~closedcurve();
double area();
double circumference();
coordinate place(double a);
};
b) Define child classes ellipse, circle, rectangle and square. The class hierarchy should
be as follows:
class ellipse: public closedcurve
{ ...
}
class circle: public ellipse
{...
}
class rectangle: public closedcurve
{...
}
class square:public rectangle
{...
}
These definitions capture the fact that all these objects are closed curves, that a circle is a special case of an ellipse and that a square is a special case of a rectangle. Implement constructors and destructors, as well as the methods for area and circumference for each of these four object types. The formulas for these methods are standard (e.g., the area of a circle is Πr2 ), except for the formula for the circumference of an ellipse, which is nontrivial.
There are many methods to approximate the circumference of a general ellipse, with major radius of length a and minor radius of length b. One such formula, that you can use, is the so-called Hölder approximation, 4(as+ bs)1/s, where s = ln(2)/ ln? (Π/2)(feel free to use other approximations). Although this formula is, in fact, exact for the case where the ellipse is a circle, the precision will be much lower than the standard formula for the circle's circumference, 2Πr. You are therefore recommended to use the latter formula for circle objects.
c) Implement the method, coordinate place(double a), for the parametric representation, which takes a number between 0 and 1, and returns the coordinate of the curve, for each of the four types of objects. The formulas for rectangles and squares are easy and the formula for the circle was described above. For the ellipse, one parameterization is:
p(a) = (x + rx*(2Πa),y + ry*(2Πa)), where rx and ry are the radiuses in the x and y direction respectively.
d) Add a method void printcurve(int N,char Name[]) to the closedcurve class, which takes a number of points, N, and a file name, Name, as arguments and outputs (x,y) coordinates for N points on the curve to a file with name "Name." For example, in the figures below, the resulting points from a square and a circle were printed to files, using N=200 points. The points were then imported to Excel, and shown using a scatter chart.
When implementing the method, use the ofstream class.
Remember to add #include <fstream> and using namespace std; in the header.
If you have implemented your class hierarchy correctly you should only have to write one method, which works for any object of type closedcurve.
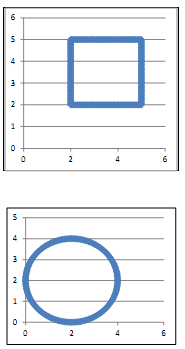
e) Implement a less-than (<) operator for the class that compares the area of one curve to another. As with printcurve above, you should only need to implement a single method.
f) Write a program that uses the curve class hierarchy. The program should define several different objects, output their area, circumference, etc. It should also use the printcurve function. Please provide charts of a couple of objects, similar to the ones above.
g) Incorporate virtual functions to get late binding of objects (if you haven't done so yet). Write a program that asks the user what type of object is needed, e.g., a circle or a square, and then calculates the area of that object. In the spirit of true polymorphism, only one
object should be defined in the program. You are allowed to use terminal input and output in this exercise.