Reference no: EM131110985
1 Goals
To write code to
- parse and load data files and deal with missing data,
- implement a more complex program involving multiple function calls.
- output nicely formatted results and figures summarizing your analyses.
2 Background - the theory governing the electrical condutivity of natural waters
Certain basic properties of lakes and oceans are important to understanding how they "work". These include tem- perature (i.e., the heat content), and the amount of dissolved matter (the salt content). One measure of the dissolved matter is the VOLUMETRIC SALINITY S, which is the total amount of dissolved stuff measured in units of grams per liter of solution. Seawater has salinities of 2-35 g/l, but even fresh water in lakes (and our drinking water) has small amounts of dissolved material (typically .1-1 g/l in most lakes, but sometimes up to 70 g/l or more in so-called
saline lakes, which usually are in hot dry places with a lot of evaporation)∗.
Temperature is pretty easy to measure, but the salinity is not. In general, the dissolved matter consists of a large number of different ions, each of which may require specialized chemical analysis techniques to measure properly. However, we can get a rough idea of the concentration of charged ions in solution by measuring the electrical
conductivity of the water at a fixed reference temperature (usually chosen to be 25?C). This is a relatively easy thing
to measure. The salinity in g/l and conductivity κ in mS/cm ("milli-Siemens per cm") are related by a factor α such that
S = ακ (1)
where this factor α is specific to a particular lake (i.e., a particular ionic composition), but is usually around 0.5-1. In this lab we are going to write to code to try and predict the α ratio a bit more accurately for lakes with a known chemical composition.
Conductivity is related to the speed at which electrical charges (attached to ions) move through the fluid (the "elec- trical current") when a voltage is applied. The total current is the combined effect of the current that arises from the effects of each ion. Let us assign each type of ion to an index i = 1 . . . N . Since different ions have differ- ent sizes, masses, and electrical charges they each have their own INFINITE DILUTION IONIC EQUIVALENT† CONDUCTIVITY λo. Then the total measured conductivity κ would be the sum of these:
where zi is the charge on the ion and ci is its concentration in MOLES PER LITER SOLUTION. For example,zi = 1 for Na+ (sodium), and zi = -2 for SO2- (sulfate). Note that the volumetric salinity can also be calculated
where Mi is the MOLAR MASS of the different ions (see Table 1), and ci is again the concentration.However, when there are lots of other ions around, their electric fields can "screen" each other, so that the actual ∗Since volume changes with temperature, assume that we are dealing with volume at a reference temperature of 25?C in this assignment. † In this context, the word EQUIVALENT means that the value is per charge, rather than per
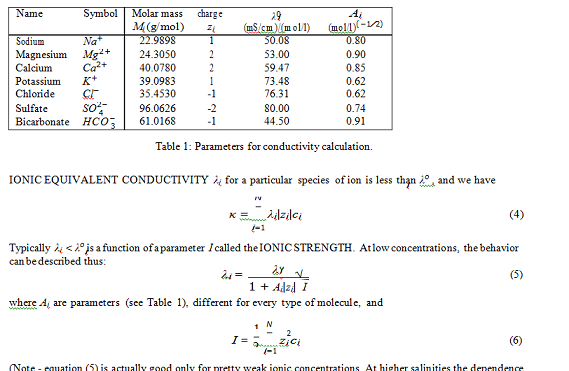
(Note - equation (5) is actually good only for pretty weak ionic concentrations. At higher salinities the dependence on I becomes more complicated, and other effects in addition to this 'screening' also become important in many natural waters, but we are going to ignore that here).
Although natural waters (i.e. lake, river, and seawaters) contain almost everything at some concentration, most of the dissolved material is composed of 7 different ions, the so-called MAJOR IONS. These are listed in Table 1, along with their molar masses, charges, and the parameters for calculating their equivalent conductivities.
One final issue is that ions are not present in arbitrary amounts. Since you don't get electrocuted when you touch water (!), the number of positive charges must EXACTLY equal the number of negative charges. That is, the CHARGE BALANCMUST equal zero (exactly) for any water containing ions. One advantage of this is that if you have a measurement that doesn't include one of the major ions (typically bicarbonate, or HCO-), you can estimate this by adding up the charges of the other ions and then setting the concentration of HCO- to balance the excess of positive charge.
Another advantage is that you can calculate the charge balance as a check value on the data, to see if it makes sense (or whether an error has crept into the values).
3 The assignment - an overview
The assignment is basically concerned with the writing of a number of functions which can implement the conduc- tivity theory described above. These functions will then be called by a program which will load data files containing the composition of a number of natural waters and make some plots comparing them. The organization of the functions is up to you, but one way of doing this is to have
• assign2.m containing the "driver" code which reads water composition data and makes plots
• cond.m containing the function cond, and subfunction istrength. This will compute the conductivity for a given chemical composition, with the subfunctions computing the ionic strength of the solution.
• chargebal.m containing the function chargebal which will compute the charge balance for a given composition.
• salinity.m containing the function salinity which will compute the salinity of a given composition.
• loadcomp.m containing the function loadcomp which will load data in a given filename, and return a row vector containing the composition.
Some other hints are:
• Since there are 7 different ions, you could use a different variable name for each in your code. However, it is more efficient to store their data in row vectors, where each column represents data for a particular ion.
• Avoid "hard-wiring" numbers into your code. Keep the (fixed) data for molar masses, ionic charges, and so on in row vectors specified ONCE. This makes it much easier to modify your program to take into account changes in the molar masses, number of ions in the compositions, or other factors.
• The simplest starting point is to write code that can load in a composition, and compute its salinity and charge balance. Missing data in composition files is specified by a -999 value. Salinities of compositions with missing data should return an error. Charge balance calculations should ignore missing data (i.e. make the calculation assuming a value of zero for the missing data).
• Above all, resist the tendency to start writing code BEFORE you REALLY understand WHAT you have to code. IN PARTICULAR, use pencil and paper to specify the INPUTS and OUTPUTs of all functions, and specify WHERE (in what functions) the different kinds of information in Table 1 is required.
4 The assignment itself
1. Read ALL the background, and ALL parts of the asignment (I wasn't kidding in the hints).
2. Begin with seawater. Load the seawater composition seawater.txt, and compute its salinity (check value 35.12978962193 g/l, perhaps varying in the last decimal place depending on details of the computation) and conductivity (check value 46.86864157996 mS/cm). The composition of seawater at other salinities can be found by scaling the ionic concentrations by a constant factor. For example, IF the salinity was 40 (it is not), then you could find concentrations for a salinity of 10 by scaling with a factor of 10/40.
• PLOT: the conductivity (on y axis) for salinities of 0.1 to 35 g/l (on x axis).Take enough steps between
0.1 and 25 so that the curve LOOKS SMOOTH when plotted.
• PLOT: the factor α (on y axis) for salinities of 0.1 to 35 g/l (on x axis). Make both of these plots as subplots, one above the other, with the x axes aligned.
3. Seawater is mostly made up of sodium and chloride (i.e. table salt). If you take a solution of ONLY Na+ and
Cl- (i.e. with the amounts of all other ions set to zero), what is α for salinities of 0.1 to 35?
• PLOT: Add the conductivity and α curves to the two from the previous section.
• TEXT: How much of a difference does approximating seawater with a sodium chloride solution make in the conductivity at a salinity of 10 g/l (i.e. what is the percent error)?
4. Read in the compositions of world average freshwater (worldriver.txt), Mahoney Lake in the interior of British Columbia (mahoneylake.txt), and Mono Lake in California (monolake.txt). Mono and Mahoney Lake are SALINE lakes. In all cases the bicarbonate concentrations are not known. Using the charge balance, estimate what these concentrations might be (i.e. so that the overall charge balance is zero) and then compute the conductivities of these waters.
• PLOT: A figure that will allow you to compare the relative composition of seawater and all 3 other water types. Be creative!
• TEXT: The dominant positive ion for seawater is Na+, and the dominant negative ion is Cl- (that is, these have the greatest concentrations in mol/l). Using your figure, determine (and state) the dominant positive and negative ion for the other water types.
• OUTPUT: A table containing the name of the lake, the input or calculated bicarbonate concentration, the conductivity of the water, and the α ratio. Use fprintf!
5 HAND IN AS HARDCOPY
• all items requested as PLOT in the form of a plot. Pay attention to the quality of the plots as part of your mark will involve plot quality as well the correctness of the results.
• all items requested as TEXT in the form of written text
• all items requested as OUTPUT in the form of a printed file
• a printout of your assign2.m script and the other functions you write.
• Electronically submit the partner.m file described next. Not handing this in will result in marks being deducted!
ASSIGNMENTS CAN BE DONE WITH OTHERS, AS PER THE LABS. Each person must hand in their own as- signment, but you MUST document the degree of collaboration. This will be done by submitting a file partner.m via VISTA. The file will contain the following lines:
partner.name='YYYYYY'; partner.stu='ZZZZ'; partner.collab='AAAA'; Time_spent= XX;
where the .collab string is a phrase like 'checked answers only', 'worked whole assignment together', or some other brief statement that describes your collaboration.
If you worked alone, you must STILL submit the file (we would like to know how many people worked alone!) but you can write the word NONE for all three partner information strings, or something like 'various people about minor issues', or...