Reference no: EM13139206
AIMS:
1. To understand actual communication with an SMTP server can be achieved using TCP Sockets.
2. To understand the development of a graphical user interface (GUI) and use of event handling.
3. To send an actual email using your GUI client
Stage 1
- To build a simple Pretend Mail Client (PMC) that prompts the user for typical email fields and then outputs SMTP protocol messages to the screen.
- To understand and construct SMTP protocol messages.
- To understand OOP design.
Write a program (e.g. called PMC.java). This program will perform the following tasks:
1. The following command is used to run your program and accept console input (i.e. input from the keyboard) for email fields and message body,
java MyPMC
For example, if the user provides information for the fields of MAIL FROM, TO, SUBJECT, EMAIL-TEXT, the output format should be as follows:
HELO xxxx.edu.au
MAIL FROM: [email protected]
RCPT TO: [email protected]
DATA
Subject: Hello
This is message .
This output should be displayed to the screen.
2. Accept console input of more complicated emails that contain various optional combinations of extra fields, in particular Carbon Copy ("CC:"), Blind Carbon copy ("BCC:"). For this task, assume that there is at most one email address in each field. That is, if the field of CC and BCC are also provided by the user (eg. CC to [email protected] BCC to [email protected]), the output format should be:
HELO xxxx.edu.au
MAIL FROM: [email protected]
RCPT TO: [email protected]
RCPT TO: [email protected]
RCPT TO: [email protected]
DATA
Subject: Hello
CC: [email protected]
BCC: [email protected]
This is message .
You need to check if the field of CC/BCC is empty (i.e. check if the user enters an email address of CC/BCC). If the fields of CC and BCC are not provided by the user, the output should be that one shown in Task 1.
3. Allow multiple comma separated addresses for each relevant field. For example, the user is allowed to enter more than one email address for the field of TO (e.g., [email protected],[email protected]). The output for this field should be:
RCPT TO: [email protected]
RCPT TO: [email protected]
4. Allow the user to send more than one email without having to restart the PMC.
5. Create a message class that includes fields of MAIL FROM, TO, CC, BCC, SUBJECT, EMAIL-TEXT. It should also include the methods of set and get for each field, e.g. for the field of From (i.e. for MAIL FROM), it should have the methods: setFrom and getFrom.
Use this class to declare 10 objects, e.g. Message [] m= new Message[10]; m[0] is used to store the first email you sent, m[1] is used to store the second email you sent, and so on. Just before exiting, your program should output the emails that you have sent. The format for each email should be the same as described in Tasks 1-3.
6. Your PMC should consist of at least 2 classes.
a. main class to get input and to control the program
b. message class
Stage 2 15 marks (Due: 5:00pm Friday 23 May 2014)
You are to develop a Java program that can communicate with a real SMTP email server for sending emails. You program should provide a GUI (see a sample GUI below) and can successfully send the SMTP commands to the mail server
The requirements are as follows:
1. The Java source files created must compile.
2. The compiled program should be able to send an actual email using correct SMTP commands
3. The GUI program must have:
a. text input fields for each required field,
b. a send button to send the email
c. a clear (or restart) button to clear fields (to, from, subject, message etc)
d. a sent button to output those emails you have sent
e. two text areas,
i. one to compose the email message and
ii. A second to display server responses
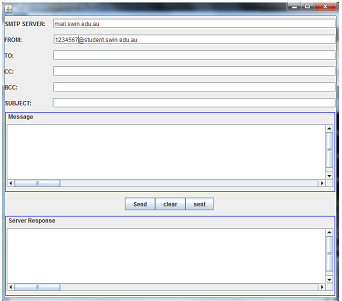
Your program must perform the following tasks:
1. Accept minimal email parts for any email recipient [These parts MUST include fields to send messages using SMTP.]
2. Make sure you validate the email fields [This means anywhere you would need an email address, the string should be a valid email address.]
3. Have default values for the Mail From field as well as which SMTP server to send the emails to. The program should allow these values to be changed if desired by user
4. Your program should receive an acknowledgement from the SMTP server and present an appropriate message to the User as to the status of the sent message
5. Make sure your CC: and BCC: functions work as well.
6. The program responds appropriately to events like clicking of a send button
7. Allow users to send more than one email without having to restart the program.
8. Handling multiple comma separated email addresses for each relevant field such as to, cc and bcc.
9. Use the message class you created in stage 1 for the output of those emails sent. You program should support the output up to 10 emails you have sent. (they can be displayed in the message area or on the console window)
You are also required to provide a technical report for your stage 2 program. The report should comprise of the following sections:
1. Title: Your name, Student ID, Team number (even if done individually)
2. A description for your GUI,
3. A description for the classes your created.
For your GUI description, you should describe the GUI components with their layout arrangement used in your program with a hierarchical structure, e.g. using a tree diagram. Here is an example of GUI design for UI1.java.
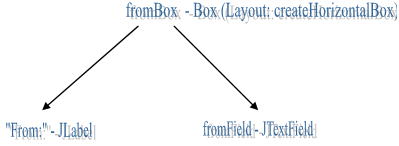
Or: fromBox (Box, layout: createHorizontalBox) includes:
"From:" ( JLabel )
fromField: ( JTextField )
Note that: you should indicate the Class name (e.g. Box) and Layout (e.g. Horizontal) you used for a
GUI component.
To describe the classes you created, you should provide the purpose of a class you created, and describe how to use each method (and each constructor if it has) in this class.
Your code should be easy to read and must be well commented.
For detailed marks distribution of this assignment refer to attached marking scheme.