Write a program to calculate the total resistance of a series or parallel circuit. The maximum number of resistors is two.
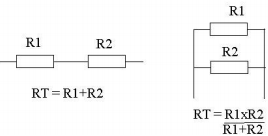
We need to decide whether the user wants the total resistance of a parallel or series circuit. Let us use scanf:
printf("Parallel (P) or Series (S) \n\r");
scanf("\n%c",&type);
Note: "\n%c" is used to remove the carriage return, when we enter in P we follow it by a carriage return i.e. 2 characters so "\n" removes the second character from the awaiting buffer. Let us Input the resistor values
printf(" Resistor Value R1\n\r");
scanf("%f",&r1);
printf(" Resistor Value R2\n\r");
scanf("%f",&r2);
The If statement allows us to test type
if (type == 'p' )
{
rt = (r1*r2)/(r1+r2);
}
else
{
rt = r1 + r2;
}
We can print out the total resistance
printf("Total resistance = %5.2f\n\r",rt);
The whole program is
include
include
include
include
include
void main()
{
char prompt;
char type ;
float r1,r2,rt;
printf("Parallel (P) or Series (S) \n\r");
scanf("\n%c",&type);
printf(" Resistor Value R1\n\r");
scanf("%f",&r1);
printf(" Resistor Value R2\n\r");
scanf("%f",&r2);
if (type == 'p')
{
rt = (r1*r2)/(r1+r2);
}
else
{
rt = r1 + r2;
}
printf("Total resistance = %5.2f\n\r",rt);
printf("Press any key to exit \n\r");
scanf("\n%c",&prompt);
}
It is worth noting that the If statement is case sensitive i.e. try 'p' instead of 'P'. Care must be taken and code should cater for upper and lower type. Considering the above program what if we want to calculate ten sets of resistor combinations, we would have to repeat this section of code ten times. Within C we have the ability to repeat sections of code using three different types of code namely: while, do while and For statements.