Reference no: EM13201569
Part 1: Product Maintenance
You'll create a series of pages that allow you to add, update, or delete a product that's available to the application.
The Index page
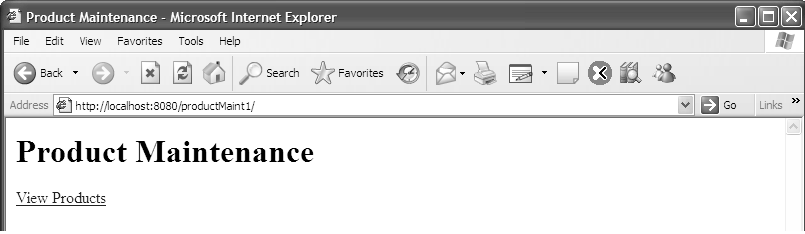
The Products page
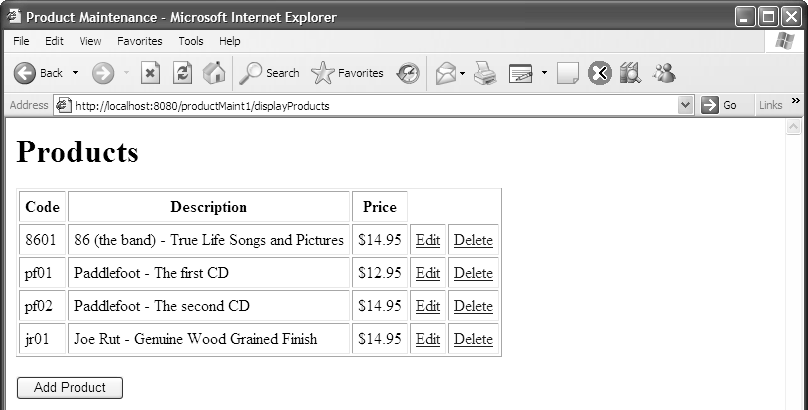
The Product page
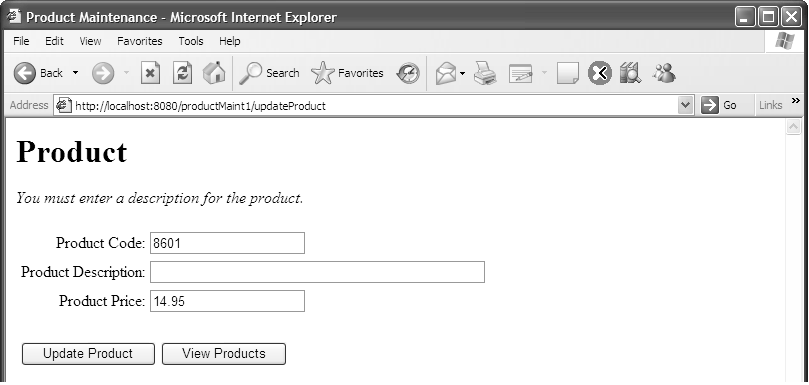
The Confirm Delete page
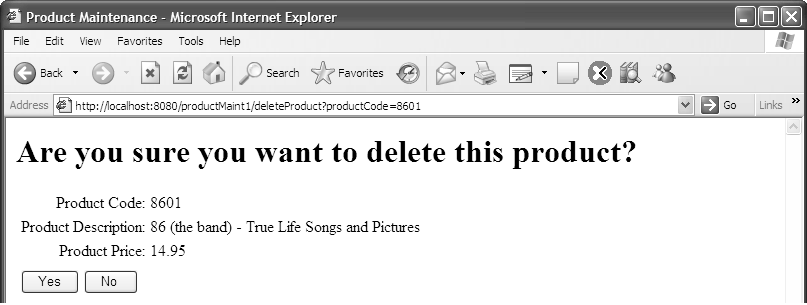
Operation
• When the application starts, it displays the Index page. This page contains a link that leads to the Products page that can be used to add, update, or delete products.
• To add a new product, the user selects the Add Product button. This displays the Product page with all text fields empty. Then, the user can fill in the text fields and click on the Update Product button to add the product.
• To edit an existing product, the user selects the Edit link for the product. This displays the Product page with all existing data for the product displayed. Then, the user can edit any entries and click on the Update Product button to update the data for the existing product.
• To delete a product, the user selects the Delete link for the product. This displays the Confirm Delete page. Then, if the user confirms the deletion by selecting the Yes button, the product is deleted and the Products page is displayed to reflect the new data. If the user selects the No button, the Products page is displayed.
Specifications
• Use a Product class like the one shown later in this document to store the product data.
• Use a ProductIO class like the one shown later in this document to read and write the product data to a text file named products.txt in the WEB-INF directory.
• Use a text file like the products.txt file shown later in this document as a starting point for the products that are available to the application.
• Use server-side validation to validate all user entries. In particular, make sure the user enters a code, description, and price for each product. In addition, make sure the product's price is a valid double value.
• If possible, get the Product.java, ProductIO.java, and product.txt files from your instructor or trainer. Otherwise, you can import starting versions of these files from the book applications.
The Product class
package music.business;
import java.text.NumberFormat;
import java.io.Serializable;
public class Product implements Serializable
{
private String code;
private String description;
private double price;
public Product()
{
code = "";
description = "";
price = 0;
}
public void setCode(String code)
{
this.code = code;
}
public String getCode()
{
return code;
}
public void setDescription(String description)
{
this.description = description;
}
public String getDescription()
{
return description;
}
public void setPrice(double price)
{
this.price = price;
}
public double getPrice()
{
return price;
}
public String getPriceNumberFormat()
{
NumberFormat number = NumberFormat.getNumberInstance();
number.setMinimumFractionDigits(2);
if (price == 0)
return "";
else
return number.format(price);
}
public String getPriceCurrencyFormat()
{
NumberFormat currency = NumberFormat.getCurrencyInstance();
return currency.format(price);
}
}
The ProductIO class
package music.data;
import java.io.*;
import java.util.*;
import music.business.*;
public class ProductIO
{
private static ArrayList<Product> products = null;
public static ArrayList<Product> getProducts(String path)
{
products = new ArrayList<Product>();
File file = new File(path);
try
{
BufferedReader in =
new BufferedReader(
new FileReader(file));
String line = in.readLine();
while (line != null)
{
StringTokenizer t = new StringTokenizer(line, "|");
if (t.countTokens() >= 3)
{
String code = t.nextToken();
String description = t.nextToken();
String priceAsString = t.nextToken();
double price = Double.parseDouble(priceAsString);
Product p = new Product();
p.setCode(code);
p.setDescription(description);
p.setPrice(price);
products.add(p);
}
line = in.readLine();
}
in.close();
return products;
}
catch(IOException e)
{
e.printStackTrace();
return null;
}
}
public static Product getProduct(String productCode, String path)
{
products = getProducts(path);
for (Product p : products)
{
if (productCode != null &&
productCode.equalsIgnoreCase(p.getCode()))
{
return p;
}
}
return null;
}
public static boolean exists(String productCode, String path)
{
products = getProducts(path);
for (Product p : products)
{
if (productCode != null &&
productCode.equalsIgnoreCase(p.getCode()))
{
return true;
}
}
return false;
}
private static void saveProducts(ArrayList<Product> products,
String path)
{
try
{
File file = new File(path);
PrintWriter out =
new PrintWriter(
new FileWriter(file));
for (Product p : products)
{
out.println(p.getCode() + "|"
+ p.getDescription() + "|"
+ p.getPrice());
}
out.close();
}
catch(IOException e)
{
e.printStackTrace();
}
}
public static void insert(Product product, String path)
{
products = getProducts(path);
products.add(product);
saveProducts(products, path);
}
public static void update(Product product, String path)
{
products = getProducts(path);
for (int i = 0; i < products.size(); i++)
{
Product p = products.get(i);
if (product.getCode() != null &&
product.getCode().equalsIgnoreCase(p.getCode()))
{
products.set(i, product);
}
}
saveProducts(products, path);
}
public static void delete(Product product, String path)
{
products = getProducts(path);
for (int i = 0; i < products.size(); i++)
{
Product p = products.get(i);
if (product != null &&
product.getCode().equalsIgnoreCase(p.getCode()))
{
products.remove(i);
}
}
saveProducts(products, path);
}
}
A product.txt file that contains four products
8601|86 (the band) - True Life Songs and Pictures|14.95
pf01|Paddlefoot - The first CD|12.95
pf02|Paddlefoot - The second CD|14.95
jr01|Joe Rut - Genuine Wood Grained Finish|14.95
Part 2: Product Maintenance without scripting
For this part, you'll enhance the application described in part 1 by removing the scripting from the JSPs and by adding a custom tag to validate user entries. (Prerequisites: chapters 1-12)
The Product page with custom tags for validation
Specifications
• Use EL and JSTL to remove all scripting from the JSPs.
• Use a custom tag to mark empty fields that are required on the Product page with an asterisk.
Part 3: Product Maintenance with a database
For this part, you'll enhance the application described in the previous part by modifying it so it uses a database instead of a text file to store the product data.
The Product page
Specifications
• Use a class named ProductDB that's in the music.data package to add, update, and delete the products in the Product Maintenance application.
• Use a connection pool as described in chapter 14.
• Use the music database that's created when you do the procedure for creating the databases that's described in appendix A.