Reference no: EM13498623
Assignment 1
Define the class bankAccount to implement the basic properties of a bank account. An object of this class should store the following data:
Account holder's name (string), account number (int), account type (string, checking/saving), balance (double), and interest rate (double).(Store interest rate as a decimal number.) Add appropriate member functions to manipulate an object. Use a static member in the class to automatically assign account numbers. Also declare an array of 10 components of type bankAccount to process up to 10 customers and write a program to illustrate how to use your class.
Assignment 2
Amanda and Tyler opened a business that specializes in shipping liquids, such as milk, juice, and water, in cylinderical containers. The shipping charges depend on the amount of the liquid in the container. (For simplicity, you may assume that the container is filled to the top.) They also provide the option to paint the outside of the container for a reasonable amount. Write a program that does the following:
a. Prompts the user to input the dimensions (in feet) of the container (radius of the base and the height).
b. Prompts the user to input the shipping cost per liter.
c. Prompts the user to input the paint cost per square foot. (Assume that the entire container including the top and bottom needs to be painted.)
d. Separately outputs the shipping cost and the cost of painting. Your program must use the class cylinderType (designed in Programming Exercise 3) to store the radius of the base and the height of the container. (Note that 1 cubic feet ¼ 28.32 liters or 1 liter ¼0.353146667 cubic feet.)
Assignment 3
In this exercise, you will design various classes and write a program to computerize the billing system of a hospital.
a. Design the class doctorType, inherited from the class personType, defined in Chapter 10, with an additional data member to store a doctor's speciality. Add appropriate constructors and member functions to initialize, access, and manipulate the data members.
b. Design the class billType with data members to store a patient's ID and a patient's hospital charges, such as pharmacy charges for medicine, doctor's fee, and room charges. Add appropriate constructors and member functions to initialize, access, and manipulate the data members.
c. Design the class patientType, inherited from the class personType, defined in Chapter 10, with additional data members to store a patient's ID, age, date of birth, attending physician's name, the date when the patient was admitted in the hospital, and the date when the patient was discharged from the hospital. (Use the class dateType to store the date of birth, admit date, discharge date, and the class doctorType to store the attending physician's name.) Add appropriate constructors and member functions to initialize, access, and manipulate the data members.
Write a program to test your classes.
Assignment 4
Programming Exercise 11 in Chapter 8 explains how to add large integers using arrays. However, in that exercise, the program could add only integers of, at most, 20 digits. This chapter explains how to work with dynamic integers. Design a class named largeIntegers such that an object of this class can store an integer of any number of digits. Add operations to add, subtract, multiply, and compare integers stored in two objects. Also add constructors to properly initialize objects and functions to set, retrieve, and print the values of objects.
Assignment 5
Banks offer various types of accounts, such as savings, checking, certificate of deposits, and money market, to attract customers as well as meet their specific needs. Two of the most commonly used accounts are savings and checking. Each of these accounts has various options. For example, you may have a savings account that requires no minimum balance but has a lower interest rate. Similarly, you may have a checking account that limits the number of checks you may write. Another type of account that is used to save money for the long term is certificate of deposit (CD).
In this programming exercise, you use abstract classes and pure virtual functions to design classes to manipulate various types of accounts. For simplicity, assume that the bank offers three types of accounts: savings, checking, and certificate of deposit, as described next.
Savings accounts: Suppose that the bank offers two types of savings accounts: one that has no minimum balance and a lower interest rate and another that requires a minimum balance and has a higher interest rate. Checking accounts: Suppose that the bank offers three types of checking accounts: one with a monthly service charge, limited check writing, no minimum balance, and no interest; another with no monthly service charge, a minimum balance requirement, unlimited check writing and lower interest; and a third with no monthly service charge, a higher minimum requirement, a higher interest rate, and unlimited check writing. Certificate of deposit (CD): In an account of this type, money is left for some time, and these accounts draw higher interest rates than savings or checking accounts. Suppose that you purchase a CD for six months. Then we say that the CD will mature in six months. The penalty for early withdrawal is stiff.
This figure shows the inheritance hierarchy of these bank accounts.
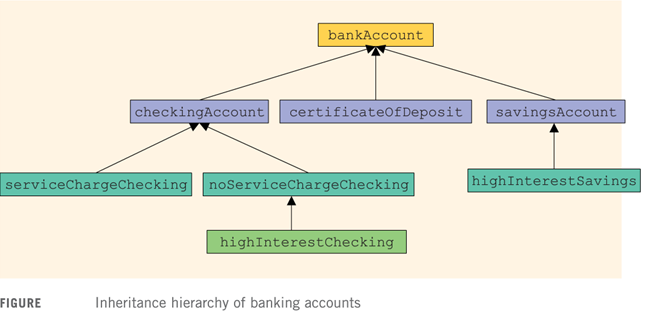
Note that the classes bankAccount and checkingAccount are abstract. That is, we cannot instantiate objects of these classes. The other classes in Figure 12-22 are not abstract.
bankAccount: Every bank account has an account number, the name of the owner, and a balance. Therefore, instance variables such as name, accountNumber, and balance should be declared in the abstract class bankAccount. Some operations common to all types of accounts are retrieve account owner's name, account number, and account balance; make deposits; withdraw money; and create monthly statement. So include functions to implement these operations. Some of these functions will be pure virtual.
checkingAccount: A checking account is a bank account. Therefore, it inherits all the properties of a bank account. Because one of the objectives of a checking account is to be able to write checks, include the pure virtual function writeCheck to write a check.
serviceChargeChecking: A service charge checking account is a checking account. Therefore, it inherits all the properties of a checking account. For simplicity, assume that this type of account does not pay any interest, allows the account holder to write a limited number of checks each month, and does not require any minimum balance. Include appropriate named constants, instance variables, and functions in this class.
noServiceChargeChecking: A checking account with no monthly service charge is a checking account. Therefore, it inherits all the properties of a checking account. Furthermore, this type of account pays interest, allows the account holder to write checks, and requires a minimum balance.
highInterestChecking: A checking account with high interest is a checking account with no monthly service charge. Therefore, it inherits all the properties of a no service charge checking account. Furthermore, this type of account pays higher interest and requires a higher minimum balance than the no service charge checking account.
savingsAccount: A savings account is a bank account. Therefore, it inherits all the properties of a bank account. Furthermore, a savings account also pays interest.
highInterestSavings: A high-interest savings account is a savings account. Therefore, it inherits all the properties of a savings account. It also requires a minimum balance.
certificateOfDeposit: A certificate of deposit account is a bank account. Therefore, it inherits all the properties of a bank account. In addition, it has instance variables to store the number of CD maturity months, interest rate, and the current CD month. Write the definitions of the classes described in this programming exercise and a program to test your classes.