Reference no: EM13909386
Part I- Description:
In this project, you will implement a program that will do three things:
1. Allows the user to draw a wireframe polygon (i.e., series of lines) using Bresenham's line drawing algorithm.
2. Allows the user to change the current drawing color (for items already drawn and items to be drawn).
3. Draws circles (using the midpoint circle algorithm) automatically at each vertex of the polygon.
For the line-drawing part, here Is what your program should be able to do:
1. When the user first presses and holds down the left mouse button, a line should be drawn from the initial point where the mouse button was pressed to the current location of the mouse. This should be updated dynamically as the user continues to move the mouse and hold down the left mouse button.
2. When the user releases the left mouse button, the line should be drawn on the screen, and the endpoint of the line should stop following the mouse position.
3. If the user presses and holds the left mouse button again, a new line should be drawn from the endpoint of the last line to the current mouse position.
4. If the user right-clicks, a line should be drawn connecting the LAST vertex drawn with the FIRST vertex. Note that NO NEW VERTEX should be created on a right-click.
5. If the user clicks the left mouse button again to start drawing AFTER a right-click, the current polygon should be cleared (as well as any circles drawn), and a new polygon started.
For the color-changing part, the user should be able to hit a key on the keyboard to change to one of 4 color chokes: white, red, green, and blue. (So, "1" = white, "2" = red, etc.)
This should update ALL colors in your color buffer to be the same color; so, if the user hits "red", all lines drawn and all lines to be drawn will draw in red.
For the circle-drawing part, a circle with a radius of 50 pixels should be drawn on each vertex of the polygon (including the one being dragged around by the user.
You must use Bresenham's algorithm to draw lines. This means you will use OpenGL to draw points only (GL_POINTS). Do NOT draw the lines with GL_LINES!
You must deal with all 8 octants for line drawing.
You may use the following code in this project:
-Any of the sample code for this class
-The Bresenham's line drawing code in the Hearn-Baker book (pg. 98-99 in the 3rd edition) as a starting point (you will need to adapt it for all 8 octants)
-The circle midpoint code found in the Hearn-Raker book (pg. 108-109 in the 3rd edition)
However, you may NOT use code from elsewhere (online or offline) for ANY part of this assignment. You also CANNOT share code amongst yourselves. These are INDIVIDUAL projects.
NO CREDIT will be given for the Bresenham code if it only handles 2 octants (i.e., what the Hearn-Baker code handles).
The current line must be updated dynamically as the user moves the mouse while pressing the left mouse button (and again, a circle must be drawn as well).
The program MUST redraw the image while Idle, so you must pass In your drawing function to glutldleFunc as well as glutDisplayFunc.
You must use OpenGL, GLUT, GLEW, and GLM for this project.
You must use OpenGL 3.0+ (so do NOT use the fixed-function pipeline, glElegin()/glEnd(), etc.). That said, just use the simple shaders from the sample code, since you should only have to modify the C++ code.
You will need vertex buffers for pixel positions as well as the color for each pixel (but the color can be set to the same value).
Bonus:
For bonus credit, when the user right-clicks and closes the polygon, instead of drawing full circles at each vertex, only draw the part of the circle segment that goes from one edge to another:
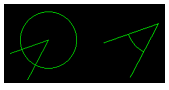
You want the Image on the right, NOT the left.
In some cases, the circle will loop around the "outside"; this is still acceptable:
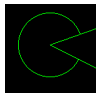
To do this, you will need to:
1. Loop through each set of three vertices → (i -1), i, (i + 1)
2. Check the angle for the two lines →(0) to (i -1), and (i) to (i + 1)
a. You will probably use the atan2() function, which Is in radians, and returns from -PI to Pl. To make all angles positive: if(angle <0) angle = 2.0*PI + angle.
3. Pick the smaller of the two angles as your "start angle" and the other as your "end angle"
4. When you go to plot each pixel in the midpoint circle algorithm, check whether the vector formed by the pixel coordinates has an angle between the start and end angles
5. If it is, draw that pixel. If not, don't.
Hints:
I would STRONGLY suggest creating a simple struct for holding 3D points:
struct Vec3 {
GLfloat x,y,z;
};
I would also add some constructors in there for ease of use.
I would suggest keeping track of the start and end points for the lines In a C++ vector (e.g., myLines); then, use your Bresenham function to fill ANOTHER vector (e.g., myPixels) with the actual pixels to draw. Finally, before you draw the lines, update the vertex buffer with the pixel data (e.g., use glilufferData() to copy the data from myPixels to the vertex buffer).
Ideally, you should create a struct for your current polygon that contains a vector with the vertices, a vector with the color for each vertex, and finally whether the polygon is "finished" or "not finished" (i.e., closed or not closed).
You should also consider making a struct for circles as well (i.e., center and radius).
Regular Version Screenshot:
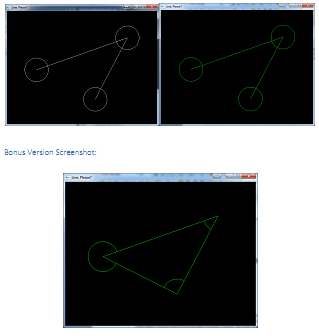
Part II- Description
In this project, you will allow the user to:
-To draw and close a polyline as in Part I; HOWEVER, there will be some key differences:
The line/fill color will be chosen based on which polygon is being drawn:
• First polygon → red
• Second polygon → green
• Third polygon → blue
• ...then repeat color pattern
After the first polygon, the alpha value should be set to 0.5
• You will use the following formula to write to the buffer:
• Dst = Src*Alpha + (1 - Alpha)*Dst
The screen should NOT be cleared after a polygon is closed; you will instead add a new polygon to draw on top of the previous polygons
-Once the polygon is closed (right-click), the polygon should be filled using the general scan-line filling algorithm.
-A new left click should start a new polygon BUT leave the previous polygons alone
You MUST use the "Render to Texture" sample as your starting point:
You will draw lines and fill polygons using "Case 2" from that sample (that is, you will write to your own color buffer and then copy that buffer to a texture).
You do not have to fill the polygon until it is closed.
You MUST meet the following requirements:
-You MUST use the "Render to Texture" sample as your starting point AND you must use "Case 2"
-You MUST use Bresenham's algorithm for lines (do NOT use GL LINES)
-You MUST use the general scan-line filling algorithm (do NOT use GL POLYGON, GL TRIANGLE, GL_TRIANGLE_STRIP,
GL_TRIANGLE_FAN, GL QUADS, or GL_QUAD_STRIP)
-You MUST use OpenGL 3.0+ (do NOT use (g1Begin()/glEnd(), gluPerspective(), glMatrixMode(), etc.); using legacy will give you a grade of ZERO
-You MUST use OpenGL, GLUT, GLEW, and GLM for this project
-You MUST deal with what to when crossing vertices (either shortening the edges or adding vertices)
-You MUST change the color with each new polygon using the pattern described above; color changes should ONLY affect new polygons (old polygons should keep their existing colors)
-You MUST do ALPHA BLENDING for all polygons after the first one! (Alpha of first polygon = 1.0, alpha of all other polygons = 0.5)
-Your code MUST compile and run! Code that does NOT compile will be given a ZERO
You must also include a README (in plain text). This should include:
-Title of project and class: so, "CS 548: Assignment 2"
- Your name and email
-OpenGL version: also include what version your shaders are using (the ttversion part)
-OS and Development environment: For example, Windows 7 and Visual Studio 2013
-Basic description of any controls: If you have any additional controls you have added, mention these.
The README will be graded on clarity, neatness, and content, and it's worth 10% of your grade.
Hints:
NOTE: You will NOT be storing the pixels in vertex and color buffers and then drawing with GL POINTS like last time. This time, you are changing pixel values directly on your own buffer, and then you're copying the buffer to a texture.
I would strongly recommend you have the following structs/classes:
-Vertex3 (points)
- Color4 (color)
- Line (stores two Vertex3)
-Edge (used in sorted edge list and active edge list)
-Polygon (contains list of lines AND the sorted edge list (which will be a list of lists)
If you use C++ stdlib vectors for your lists (which I would recommend), remember you can insert the contents of one vector onto the end of another vector using:
A.insert(A.end(), B.begin(), B.end());
Also, you will have to sort edges by x values. You can use functions from the stdlib to do this. You will have to create a function to do the sort. Let's assume you have an Edge struct with x in it:
int compareEdges(const Edge a, const Edge b){
return (int)(a.x < b.x);
}
...
// To do the sort
sort(A.beginQ, A.end(), compareEdges);
This will sort from the smallest to largest values of x.
Each edge's current x value can be a float (so feel free to just add the inverse slope and use floor() to get the new pixels in the FILLING algorithm).
You can build the sorted edge list for each polygon ONCE and save it in each polygon after it's complete. The active edge list will still need to be built every time the polygon is drawn.
REMEMBER: You should only add/shorten edges if the vertices around it are MONOTONICALLY increasing/decreasing in y!
NOTE: Do NOT add edges to the sorted edge list that are horizontal!
ALSO REMEMBER: You do NOT need a destination alpha channel for this to work! (You only need the source alpha.)
Screenshot:
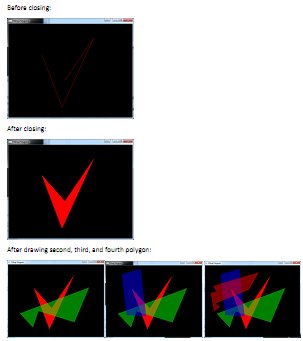
Attachment:- RenderToTexture.zip