Reference no: EM131421780
Part A -
1. Create the following class hierarchy, with Option at the top. Option then has derived classes EuropeanOption, AmericanOption, etc. We are going to define a number of methods (some of them virtual functions) within Option that will allow us to value options.
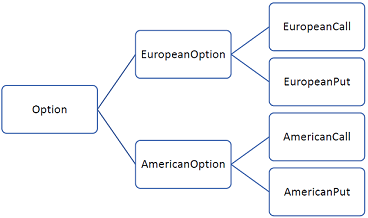
2. Create a constructor in Option that takes double K, double T, double sigma and double r as strike, time-to-maturity, stock volatility and risk-free rate parameters respectively. This constructor should be used by each of the derived classes in their constructors. Additionally, the Option class should keep protected properties for Strike, TimeToMaturity, Sigma and r.
3. To Option, add a virtual function double getExerciseValue (double s , double t) that returns the exercise value of the option for spot price s at time t.
Note: for American options, this just returns the intrinsic value of the options. For European options, if t ! = TimeToMaturity, then the exercise value should be zero (that is, European options can only be exercised at maturity).
4. To Option, add a virtual function:
double getBlackScholesValue (double s) that returns the Black Scholes value of the option.
5. To Option, add a method:
double getBinomialTreeValue (double s , int N) that returns the value of the option using a binomial tree of depth N. As with getBlackScholesValue(), the value of the option should be taken for a spot of s
a. You should use a single vector<double> to hold your tree node values.
b. The binomial tree should handle early exercise by comparing the current node's "binomial" value to the exercise value at that time and spot.
c. The getBinomialTreeValue() method should not be virtual. That is, the tree can be implemented using the getExerciseValue() method and does not need to know specifically what type of option is being valued. Below is some pseudocode that should be helpful. Source: https://en.wikipedia.org/wiki/Bihomial options pricing model
6. To Option: add a virtual function:
double getValue (double s) that returns the option value for spot value s.
European options should return the Black-Scholes value. American options should return the binomial tree value of the option with a tree depth of 250.
7. Make sure to implement the virtual functions, at the appropriate level, for all the option classes. For example, if a class can implement the function for all its derived classes (like getValue()), then implement the function within that class. If not, then implement the function within derived classes. Remember that calls and puts have different forms of the Black Scholes formula.
Part B -
1. Create a Bond class with a constructor that takes principal, coupon, recovery rate and time to maturity as parameters. Store the parameters as properties. For the purposes of the homework, we will assume that coupons (if any) are paid annually.
2. Add a public method called double getPrice (double YTM) which returns the bond's price given its yield to maturity. The formula for price given yield to maturity is:
price(T, YTM) = principal/(1+YTM)T + coupon * i=0∑T-i>0 1/(1+YTM)T-i
Notice that we count "backwards" from time to maturity to discount coupons. So, if T=5, we discount coupons at 5 years, 4 years, 3 years, 2 years and 1 year. If T=4.75, we discount coupons at 4.75 years, 3.75 years, 2.75 years, 1.75 years and 0.75 years.
3. Add a public method called double getYTM (double price) which returns the bond's yield to maturity given its price. Note: you will need to use a root-finding method like the secant method to search for the appropriate YTM. Use a function like:
f(ytm) = price - getPrice(ytm) to find the ytm that produces the expected price
4. Add a public method called double getHazandRate (double price, double r) which returns the bond's hazard rate for a given price and risk-free rate.
You can estimate the hazard rate using the following formula:
YTM - r/1 - RecoveryRate
5. Add a public method called double getDefaultProbability (double t, double price, double r) which returns the bond's default-probability for a given price and risk-free rate.
Remember, to calculate default probability (dp) we first calculate survival probability (sp).
sp(t) = e-ht, dp(t) = 1 - sp(t)
6. Add a public method called double getModifiedDuration (double YTM) which estimates the modified duration of the bond using the following formula:
modified duration = 100 * (price (ytm) - price(ytm+0.01)/price(ytm))
That is, it estimates the modified duration by adding 1% to the yield-to-maturity and calculating the price difference.
Attachment:- Assignment Files.rar