Reference no: EM13376422
(1) The Model Classes:
Define and compile the following two classes that will represent seats in a stadium:
public class Seat {
public static int[] PRICING = {74, 47, 32, 19};
private byte section;
private char row;
private byte number;
public Seat(byte s, char r, byte n) {
section = s;
row = r;
number = n;
}
public byte getSection() { return section; }
public char getRow() { return row; }
public byte getNumber() { return number; }
public int getPrice() { return PRICING[section-1]; }
}
public class Stadium {
public static int ROWS = 27;
public static int COLUMNS = 35;
private static String[] SEAT_NUMBERS = {
};
private static String[] SEAT_ROWS = {
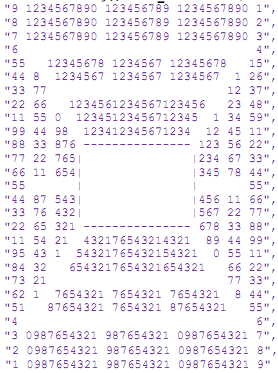
};
private static String[] SEAT_SECTIONS = {
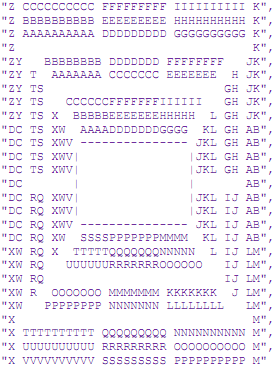
};
private Seat[][] seats;
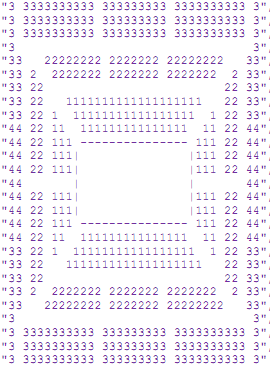
public Stadium() {
seats = new Seat[ROWS][COLUMNS];
for (int r=0; r< ROWS; r++) {
String secString = SEAT_SECTIONS[r];
String rowString = SEAT_ROWS[r];
String numString = SEAT_NUMBERS[r];
for (int c=0; c< COLUMNS; c++) {
byte section = (byte)Character.digit(secString.charAt(c),10);
char row = (char)rowString.charAt(c);
byte number = (byte)Character.digit(numString.charAt(c),10);
if (!Character.isLetter(row))
seats[r][c] = null;
else
seats[r][c] = new Seat(section, row, number);
}
}
}
public Seat[][] getSeats() { return seats; }
public Seat getSeat(int row, int col) { return seats[row][col]; }
}
When creating a new Stadium, the constructor above will fill a 27x35 two-dimensional array of Seat objects, where each Seat has a number, row and section. There are locations in the stadium where no seat resides ... therefore in the 2D array, there will be some null locations.
(2) The StadiumPanel Class:
Create a subclass of JPanel called StadiumPanel which will display a 2D array of JButtons as follows:
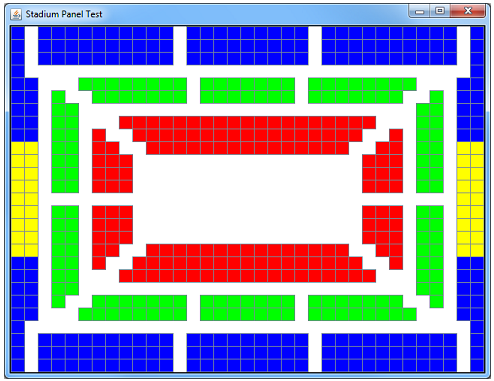
The constructor for this class should take a single Stadium parameter and then, using a GridLayout, arrange a 2D array of JButtons as shown above. If there is a Seat at the given row and column of the Stadium, add a JButton at that location in the grid. If there is no Seat (i.e., there is a null in the array) at that location, then add a blank JLabel instead of a JButton at that location in the grid. The background of the StadiumPanel should be white and all JButtons should be colored according to the Seat section that they represent. Section 1 should be red, section 2 green, section 3 blue and section 4 yellow (as shown above). Add the following to the end of your StadiumPanel class to make sure that it displays properly as the window is resized:
Save and compile the following BoardPanel class which represents a fixed-size JPanel:
import java.awt.*;
import javax.swing.*;
public class BoardPanel extends JPanel {
static int WIDTH = 633, HEIGHT = 462;
public int getWidth() { return WIDTH; }
public int getHeight() { return HEIGHT; }
public Dimension getSize() { return new Dimension(WIDTH, HEIGHT); }
public Dimension getSize(Dimension rv) {
rv.width = WIDTH; rv.height = HEIGHT; return rv;}
public void setBounds(Rectangle r) {
super.setBounds(new Rectangle(r.x, r.y, WIDTH, HEIGHT));
}
public void setBounds(int x, int y, int w, int h) {
super.setBounds(x,y,WIDTH,HEIGHT);
}
public Dimension getMaximumSize() { return new Dimension(WIDTH, HEIGHT); }
public Dimension getMinimumSize() { return new Dimension(WIDTH, HEIGHT); }
public Dimension getPreferredSize() { return new Dimension(WIDTH, HEIGHT); }
public void setSize(Dimension d) { }
public void setSize(int x, int y) { }
}
Change StadiumPanel to be a subclass of BoardPanel. Re-run the code to make sure that it no longer re-sizes as the window re-sizes.
(3) The StadiumApp Class:
Now you will create a StadiumApp class which represents the window shown on the next page. The window must use a GridBagLayout manager. The window has exactly 5 components:
1. a StadiumPanel
2. a JPanel that must use a GridLayout to display the section, row, number and price of a seat.
3. a JPanel that must use a GridLayout to display the price, HST and Total Cost for all selected seats.
4. a Purchase button
5. an Administrator button
Note that you MUST use the layout managers described above, otherwise you WILL NOT receive any marks for this part of the assignment. The window shown has a size of 840 x 505. The window may look weird when re-sized, but that is ok. Once you get it arranged nicely, use setResizable(false) to disable window re-sizing behavior. You may need to then adjust the window size to 840 x 494 or something like that to adjust for the margins which changes when you made it non-resizable.
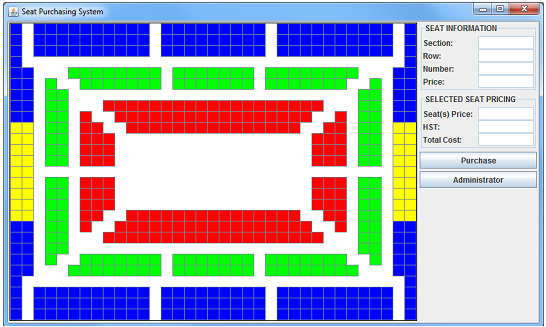
(4) Finishing Touches:
Add a MouseListener to each of the JButtons that represent a seat. Write the mouseEntered(MouseEvent e) and mouseExited(MouseEvent e) methods so that when the mouse hovers over a seat, the section, row, number and price of that seat is displayed in the Seat Information panel on the window. When the mouse leaves a seat, then those text fields should become blank again.
Add a private selected attribute to the Seat class along with public isSelected() and setSelected(boolean s) methods.
Add an ActionListener to each seat's JButton so that when pressed, the Seat clicked on becomes selected. Selected seats should be shown as Color.GRAY. You may want to write an update() method that simply goes through all seats and sets their color to either GRAY (if selected) or their default color as before. Then just call the update() from the ActionListener after you set the seat to be selected or unselected.
In the update() method that you wrote, go through all seats and total up the price for all currently selected seats and show the selected seats combined price in the SELECTED SEAT PRICING panel along with the HST and total Cost. These fields should ALWAYS reflect the totals for currently selected seats (see picture on next page).
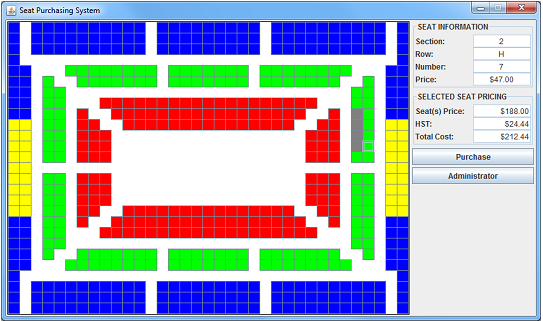