Your assignment for the semester will involve the development of a system for drawing trees using the Python programming language and the turtle graphics module (turtle.py). Completion of the assignment will involve the use of many of the programming constructs that you have seen previously in the labs. Your assignment mark constitutes 10% of your overall final module mark. If you get stuck, remember to ask for help - that's what the lab sessions are for!
Note: A model answer for the last question in lab 17+18 has been posted to the assignment directory. It is recommended that you use this file as a starting point for your program.
TASK
Your project will be to construct a system that allows you to draw trees using a fractal pattern similar to the Sierpinksi Triangle seen in the last two labs (Labs 17 and 18).
The remainder of this script guides you through the development of the system on a step-by-step basis and provides instructions for submission of your assignment.
STAGE 1 - DRAWING TREES
Modify the code you used in Labs 17 and 18 for drawing the Sierpinski Triangle. Instead of drawing three lines at 120 degrees to each other, change your method so that it allows you to specify as parameters the number of lines to draw and their angle of separation. For example, if you draw 6 lines at an angle of 64 degrees, then the first step of the drawing should look like this:
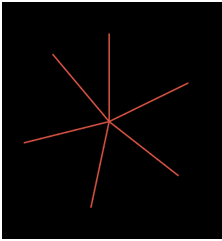
As with the code for Labs 17 and 18, there should be a parameter that defines how much each line is reduced each step. If the reduction is by 0.5, then for the next step of the drawing, 6 further lines are recursively drawn from the end points of the previous 6 lines each in the same pattern as above but reduced in size (in a manner similar to the way the Sierpinski's Triangle was drawn). This ends up looking like this:
Repeating this for the next step, the drawing will end up looking like this:
After 5 steps, the drawing looks like this:
As with the code for Labs 17 and 18, the code should contain parameters that allow you to modify the following:
• The initial heading of the first line drawn;
• The initial x and y co-ordinates of where the drawing should begin.
Add yet further parameters so that you can also specify the following:
• The initial thickness of the lines drawn;
• The ratio at which the thickness of the lines gets reduced each step (if this is set to 1.0, then the thickness remains the same for all lines drawn).
STAGE 2 - LET THE USER SPECIFY THE PARAMETERS
Allow the user to enter the parameters prior to the drawing of the fractal pattern. For example, ask the user what angle of separation they would like, the number of lines to draw, the length of the initial lines, how much each line is reduced each step, and the value of the four parameters listed above. This will allow you to easily try out many different configurations when testing your program. (Note: In the report you submit for your assignment, make sure you include a section on how you tested your program, along with screenshots that show it working).
STAGE 3 - DRAWING FORESTS
Change your program so that multiple trees can be drawn. Add a further two parameters that define the number of trees that will be drawn, as well as the width between them (along the x axis). Then draw the forest of trees once the user has finished inputting the values of all the parameters.
For example, if the user wanted to draw 3 trees in their forest, separated by 10 units when the length of the initial lines is also set at 10 units, then the output would look something like this:
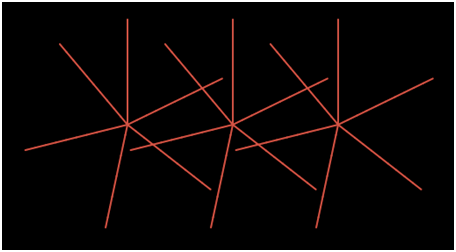
Repeating this five times, the output would look like this:
STAGE 4 - ADDING SOME RANDOMNESS
So far the trees you have drawn are very regular. Add some randomness to your drawings so that the colour is randomly chosen for each line drawn rather than the same at each recursive step (as in the above images). Add further randomness by randomly choosing to stop drawing a percentage of some of the lines (and therefore all the lines that would have been recursively drawn latter on for those lines). This should result in lop-sided trees that look a bit more like trees do in nature. For example, in the following image, the leftmost tree has all its "branches", but the middle tree has randomly lost two of its branches, and the rightmost tree has lost three:
Repeating this random growth pattern for the next four steps might end up with a forest that looks like the following:
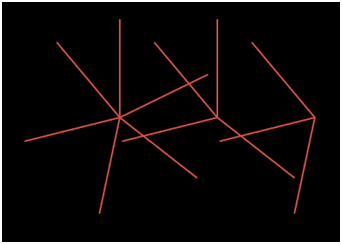
STAGE 5 - TESTING OUT YOUR CODE
Thoroughly test your code out by getting it to draw different forests using different values for the user input. Post screenshots of the output of your program. Marks will be awarded for the variety of the screenshots.
STAGE 6 - PRESENTATION OF YOUR CODE
Remember to structure your code neatly by indenting correctly and including blank lines occasionally such that it is easier to follow. Include comments using the ‘#' symbol to explain how your code works. If your code looks like spaghetti to you, imagine how it will look to another programmer who has never seen your code before! You will gain marks for following correct coding conventions here.
STAGE 7 - USER MANUAL
You've commented your code and structured it neatly so that other programmers will be able to follow your code but what about those people who are less-technically minded who will actually be using your program? Write a user guide explaining how your program works. Include screenshots guiding a user through the process of operating your software. Bear in mind that these users are likely not programmers themselves.
You will gain marks for the presentation & clarity of your work here.