You will be creating a World that consists of ants and doodlebugs. Each time you click the board each bug will do some of the following: move, bread, eat, and starve.
Ants will function in a certain way, and doodlebugs in another.
This assignment is based on Absolute Java.
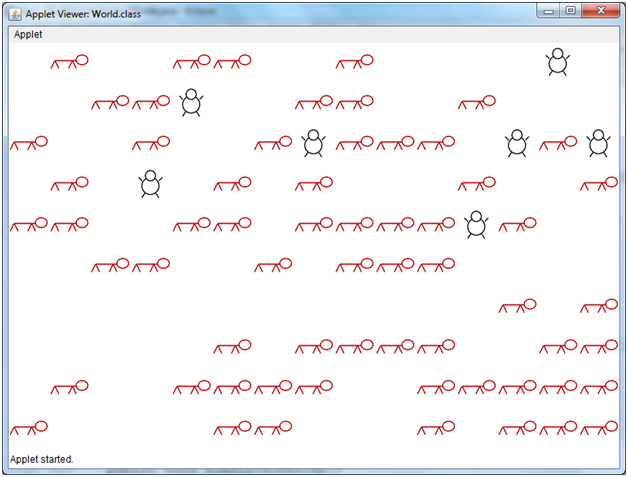
Bug Rules
Ants
Move
- Every time step the ant will attempt to move.
- Pick a random direction using a random generator (up, right, down, or left).
- If the space is on the grid, and not occupied then the ant will move there.
Breed
- If an ant survives 3 time steps he will attempt to breed.
- He breeds by creating a new ant in a space adjacent to himself.
- If there is no empty space adjacent to himself to breed then he will not do so.
- He will then wait 3 more time steps until tries to breed again.
Doodlebugs
Move
- Every time step the doodlebug will move.
- First he will check out each direction. If there is an ant in an adjacent space he will move there (consequently taking up the ants space and eating him!)
- If there was no ant in an adjacent space he will move just like ants do.
- Note: doodlebugs can't eat other doodlebugs
Breed
- If a doodlebug survives 8 time steps he will attempt to breed.
- He breeds in the same manner as an ant.
Starve
- If an ant has not eaten an ant within the last 3 time steps, then at the end of the last time step he will die of starvation. You will remove him from the grid.
UML
Ants and Doodlebugs will extend from a generic Organism.
Create a UML diagram for the Organism, Ant and Doodlebug. This diagram may change as you develop your design, but having a basic flow will greatly help in the implementation.
Provided Files
- World.java
- Organism.java
- Ant.java
- Doodlebug.java
- ant.png
- doodlebug.png
Word
Methods you will use in your classes. Don't modify this class.
public Organism getAt(int x, int y)
- Returns the Organism at the x, y position in the grid.
- If there is no organism it returns null
public void setAt(int x, int y, Organism bug)
- Sets the entry at the x, y position in the grid to the specified organism
public boolean pointInGrid(int x, int y)
- Returns true if the point x, y is in the grid and false if it goes beyond the grid space (e.g. if x = -1 that is not in the grid)
Images
- Put the two images in an images folder within your src
What you need to edit
- Organism.java
- Ant.java
- Doodlebug.java
Organims
Here are the methods that the World calls on the Organisms. I assume you will want to make more methods, such as move...
Organism(World world, int x, int y)
- Creates a new Organism
- The coordinates in the grid (X and Y) are required so you can pick a new location relative to its current to move to, and breed at.
- The World is required so you can call methods like getAt(x, y), setAt(x, y), and pointInGrid(x, y) on it.
public abstract String toString()
- This method is written for you
- Returns the string representation of the organism ("ant", "doodlebug"). Used by the World to determine which type of organism it is.
public void resetSimulation()
- This method has been written for you
- You will need to keep track of weather the organism has simulated this time step or not in an attribute of the class. This is important as it stops an organism from moving to a new place in the grid and then simulating again.
public boolean simulate()
- This will set the attribute that keeps track of weather it has simulated to true. This method returns true if it simulates, and false if it doesn't (has already simulated)
- If the organism was created this time step don't do the rest
- Call move, then breed, then starve (only for doodlebugs).
- You will then increment a time step counter
Ant and DoodleBug
- I want you to figure out what goes here