What is aspect oriented programming?
The Aspect-oriented software development is the latest technology for the separation of the concerns (SOC) in the software development. These techniques of AOSD make it possible to modularize crosscutting aspects of the system.
When we look at the times of COBOL where we used to break the modules in small functionalities and use reusability to its maximum.
Then came the time interval when we talked in terms of Objects where things were clearer as software was modeled in the terms of real life examples. It worked fine and till date is the most accepted way of implementing and organizing the project. So the question is why AOP?
The Aspect oriented programming does not oppose the OOP's but rather supports it and make it more maintainable. So to remove the logic from head the AOP is the replacement of the OOP.
When we describe in terms of objects it is an entity which maps to the real world domain. The Object has attributes which represent the state of the object and also define its behavior. By the rule of object oriented programming object must be stand alone and communicate with each other objects using messages or defined interface.
One object must not communicate with the other object directly rather communicate through the defined interfaces. Each object satisfies some "Concern" in relation to the system.
There are primarily 2 types of concern from an object perspective:-
1) The Main /Core concerns which it must satisfy and is his work.
2) The System concerns that are not related to the business functionalities but software related concerns e.g. Error handling, audit trail, Security etc.
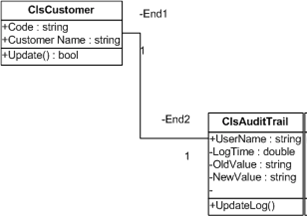
Figure :-Customer and Audit trail relationships
The class diagram above shows the relationship between the two classes "ClsCustomer" and "ClsAuditTrail". The "ClsCustomer" class does inserting of the new customers into the database and the "ClsAuditTrail" does the auditing of what is modified in the customer class.
There are two main concerns in this project :-
1)The Customer code should not more than 10 lengths (Business level concern) greater
2)All the customer data which is updated must be audited. (System level concern)
Below are s the class codes. If you view the ClsCustomer implementation in the update method I have called the Audit trail implementation. If you really look from the object oriented point of view we are doing something in the customer class which is supposed to be not his implementation: - Audit Trail logging. Thus we have also split down the rule of the encapsulation. In brief the class not only handles his work but also some another work which is not his concern.
When one or many than one concerns span across module it is known as cross cutting. For e.g. in the audit trail we will probably require to audit trail for customer as well as the supplier. Hence the Audit trail can span across other objects also that is known as cross cutting.
Both the classes actually implemented as per class diagram is shown below . If you see the "Update" method of the customer class, its doing both of the concerns that is checking for the customer code length and also maintaining the audit trail by using the audit trail class.
Public Class ClsCustomer
Private pstrCustcode As String Private pstrCustName As String Public Property Code() As String
Get
Return pstrCustcode
End Get
Set(ByVal Value As String)
pstrCustcode = Value
End Set
End Property
Public Property CustomerName() As String
Get
Return pstrCustName
End Get
Set(ByVal Value As String)
pstrCustName = Value
End Set
End Property
Public Function Update() As Boolean
' first / core concern
If pstrCustcode.Length() > 10 Then
Throw New Exception("Value can not be greater than 10") End If
' usingthe customer audit trail to do auditing
' second concern / system concern
Dim pobjClsAuditTrail As New ClsAuditTrail
With pobjClsAuditTrail
.NewValue = "1001"
.OldValue = "1003"
.UserName = "shiv"
.Update() End With
' then inserting the customer in database
End Function
End Class
Public Class ClsAuditTrail
Private pstrUserName As String Private pstrOldValue As String Private pstrNewValue As String Private pdblLogTime As Double
Public Property UserName() As String
Get
Return pstrUserName
End Get
Set(ByVal Value As String)
pstrUserName = Value
End Set
End Property
Public Property OldValue() As String
Get
Return pstrOldValue
End Get
Set(ByVal Value As String)
pstrOldValue = Value
End Set
End Property
Public Property NewValue() As String
Get
Return pstrNewValue
End Get
Set(ByVal Value As String)
pstrNewValue = Value
End Set
End Property
Public Property LogTime() As Double
Get
Return pdblLogTime
End Get
Set(ByVal Value As Double)
pdblLogTime = Value
End Set
End Property
Public Sub Update()
' do the logging activity here
End Sub
End Class
In brief the customer class is doing much activity. There is a lot of tangling of code. So how do we get over this problem...? Simple, split the System level concern (Audit Trail) from the core level concern (Customer code check). This is achieved at this moment in the .NET using the attribute programming.
Here are some of the changes to the customer class
Imports System.Reflection
Public Class ClsCustomer
Private pstrCustcode As String Private pstrCustName As String Public Property Code() As String
Get
Return pstrCustcode
End Get
Set(ByVal Value As String)
pstrCustcode = Value
End Set
End Property
Public Property CustomerName() As String
Get
Return pstrCustName
End Get
Set(ByVal Value As String)
pstrCustName = Value
End Set
End Property
_ Public Function Update() As Boolean
If pstrCustcode.Length() > 10 Then
Throw New Exception("Value can not be greater than 10") End If
' usingthe customer audit trail to do auditing
' then inserting the customer in database
End Function
End Class
And here is the change to the audit trail class
Imports System.Reflection
Targets.All)> _ Public Class ClsAuditTrail
Inherits Attribute
Private pstrUserName As String Private pstrOldValue As String Private pstrNewValue As String Private pdblLogTime As Double
Public Property UserName() As String
Get
Return pstrUserName
End Get
Set(ByVal Value As String)
pstrUserName = Value
End Set
End Property
Public Property OldValue() As String
Get
Return pstrOldValue
End Get
Set(ByVal Value As String)
pstrOldValue = Value
End Set
End Property
Public Property NewValue() As String
Get
Return pstrNewValue
End Get
Set(ByVal Value As String)
pstrNewValue = Value
End Set
End Property
Public Property LogTime() As Double
Get
Return pdblLogTime
End Get
Set(ByVal Value As Double)
pdblLogTime = Value
End Set
End Property
Public Sub New(ByVal pstrUserName As String, _ ByVal pstrOldValue As String, _
ByVal pstrnewValue As String, _ ByVal plng As Long)
Update() End Sub
Public Sub Update()
' do the logging activity here
End sub
End class