Reference no: EM13544946
(Date and String Builder)
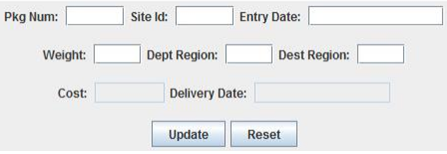
Source code for above Panel is provided.
(a) When the Update button is clicked, the Cost and Delivery Date text fields should be filled in based on data in the other fields. These two textfields should not be editable by the user (note that JTextFields have a setEditable() method).
• Modify PackageCostNDatePanel.java to set the fields non-editable.
(b) Cost for a package is computed as $2 per pound plus 3 times the difference between destination region and departure region (thus the further a package is going, and the more a package weighs, the more the cost). For example, if the package weighs 4 pounds and is going from region 5 to region 3, the cost is computed as: 2*4 + 3*abs(5-3) which evaluates to 8+6 or $14.
• Modify getCost() of Package.java to calculate cost.
(c) The delivery date should be 3 business days after the entry date. For example, if the entry date were 4/22/2014, then the delivery date should be computed as 4/25/2014 (package is dropped off on a Tuesday and delivered 3 business days later on a Friday). If the entry date were 4/25/2014, then the delivery date should be computed as 4/30/2014 (package is dropped off on a Friday and three business days later is a Wednesday). For the purposes of this lab, you may ignore holidays. Any weekday (Monday thru Friday) should be considered a business day. You should have used the GregorianCalendar class in previous labs.
You'll want to parse the Entry Date and create a GregorianCalendar object to represent it. Note that the GregorianCalendar class has an add() function that allows you to add a certain number of days to the current date. You can also find out what day of the week, a date falls on (by using the get() method). Thus, for the Delivery Date, you can make a copy of the Entry Date (what function would you use to do that?) and then adjust the date so it is three business days later. For more information on GregorianCalendar, read the lecture notes, the course textbook, and refer to the Java Sun API website at:
https://java.sun.com/javase/6/docs/api
• Modify setDeliveryDate() of Package.java
(d) When the Reset button is clicked, the UI components should be cleared (reset the UI).
• Modify reset() of PackageInfoPanel.java
• Modify reset() of PackageCostNDatePanel.java
(e) Modify toString() of Package.java to print the Package information.
Section A (Exception Handling):
• Create a new class named BadPackageDataException following the exception chaining approach taught in class by professor (refer Exception Handling.pdf) and use this class for throwing exceptions:
Data validation in PackageInfoPanel.java
• Check all the data entered are valid integers, if nothing is entered or non-integer value is entered then throw BadPackageDataException with appropriate message.
• Check if package number entered is greater than zero, if not, throw BadPackageDataException with appropriate message.
• Check if weight entered is greater than zero, if not, throw BadPackageDataException with appropriate message.
Entry Date validation in Package.java
• Check if the data entered is in the format of MM/DD/YYYY, if not, throw BadPackageDataException with appropriate message.
• Check if the year in entry data should not be less than 2014, if so, throw BadPackageDataException with appropriate message.
Section B (Threads):
• Modify PackagePanel.java to make it a thread.
• Modify ImplementPackage.java to start a new thread of PackagePanel.java.
Section C (Flow of execution):
• Write the flow of execution of this application step by step and briefly explain the functionality of each function being called (like the first step would be to instantiate Frame and then instantiate Panel and place it into the Frame).