Reference no: EM1379691
Linked Lists
Along with vectors, linked lists are one of the fundamental data structures in computer science. Unlike vectors, which store information in a contiguous block of computer memory, linked lists have the potential to store information in non-adjacent memory blocks. In this lab, you will write a very basic doubly-linked list. To complete this lab, you will need to have created the appropriate .h and .c files that
implement the following struct and functions:
typedef struct IntList_t
{
int value;
struct IntList_t *previous;
struct IntList_t *next;
} IntList_t;
IntList_t* intList_init(int starting_value)
Like our vector's init function, the LL version must dynamically create a new IntList_t and set its starting value as well as setting the previous and next pointers to NULL.
IntList_t * intList_rewind(IntList_t *some_list)
This function returns a pointer to the "beginning" of the linked list. For our purposes, we will consider the beginning of a linked list to be represented by the IntList_t whose previous pointer is equal to NULL.
void intList_add(IntList_t *some_list, int value)
This function will add a new IntList_t to the end of the current linked list. Note that for our purposes, the last element in the list is represented by the individual IntList_t whose next pointer is equal to NULL.
void intList_remove(IntList_t *some_list)
This function removes the supplied IntList_t from the current chain of list items.
void intList_free(IntList_t *some_list)
Freeing up dynamic memory allocated for a linked list is a little more work than freeing up dynamic memory for a vector. As such, this function should call free on every node in the linked list.
Sample Output
To test these methods, you must write a simple test driver in your main function. Here is an example of my test driver:
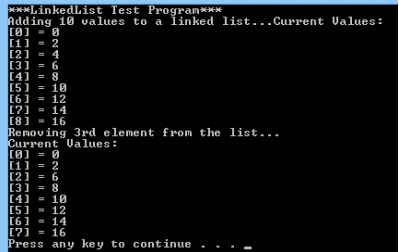
Determine a source code control system
: Determine a source code control system and discuss why is such a system necessary when multiple programmers build a program or system?
|
Describe the concept of a signal
: Assume when a child process is forked, a parent may wait for the successful completion of the child via the wait service so that return result of that application can be read from the procedure descriptor block.
|
Discuss mitigation strategies
: It is determined that 3-out of 5-businesses that experience downtime of forty-eight hours or more will be out of business within three years.
|
Overview of multiprogramming mode
: Member of coordinating a computer's activities is handling failure. This is called fault tolerance. Briefly explain about how a computer handles loss of power to limit the loss of all work
|
Write a very basic doubly-linked list
: CptS 122 Lab #2: Linked Lists , Along with vectors, linked lists are one of the fundamental data structures in computer science. Unlike vectors, which store information in a contiguous block of computer memory, linked lists have the potential to sto..
|
Compute the net expected value for the project
: You are considering to make modifications to an existing application. Compute the net expected value for the project risks and opportunities
|
Enable multimedia on a website
: Few multimedia can be hard to watch on a mobile device due to screen size or bandwidth limitations. Determine two articles that discuss considerations and new developments that will enable multimedia on a site
|
Circuit switch and packet switch environment
: Provide two examples of a circuit switch and packet switch environment. Base one example on a circuit switch and the other on a packet switch.
|
Determine the largest value
: A soft real time system has 4-periodic events with periods of 50, 100, 200 and 250 msec each. Assume 4-events need 35, 20, 10 and X msec of CPU time, respectively.
|