Reference no: EM13908871
You are to write a simple menu-driven transaction system for a hospital patient record-keeping application. A hospital has a number of types of patients, such as outpatients, emergency room, and inpatients. Each patient may be either male or female.
Clearly, there are common data for all patients, such as name social security number, insurance name and number, and age. Outpatients have appointment times, while inpatients have room numbers. Female patients and male patients also have some different medical data.
You are to build an inheritance hierarchy of patients such that the base class is a generic patient. The base class should have four derived classes, Male, Female, Outpatient, and Inpatient. At the next level you will have four multiply-derived classes, i.e. MaleIn, FemaleIn, MaleOut, FemaleOut. Be sure to consider when the derivation should utilize a virtual base class so that class members are not needlessly duplicated in lower-level derivations.
To implement polymorphism, you will use the virtual class member functions to enable invoking the appropriate member function at run time. To hold a hybrid array of base- related classes, the array will hold pointers to the base class objects. This will enable a function receiving pointers to access the appropriate object functions.
Your program should offer a menu to add a new patient, display all patients, display all outpatients (Male or Female), display all inpatients (Male or Female), or quit. Therefore you will create an array of pointers to the base class (although no objects of the base class will ever exist). When an "add" is chosen, the user is asked for the patient type. For example, if the type is male outpatient, we dynamically allocate (using new) a male outpatient object, fill in the appropriate information from the user and assign the object pointer to the next available slot in the patient array. You do not have to do any patient updates or removals for this program.
Since we want to make this an OO system, the array itself along with the functionality of displaying should be an object of a class, say Hospital.
Your output should clearly demonstrate that the program implements and achieves all the OO requirements as outlined above.
Your main program should have similar code as shown below, but be creative in defining your own driver module. Remember to make it menu-driven console application (interactive).
Class diagram of the hospital patient record-keeping application
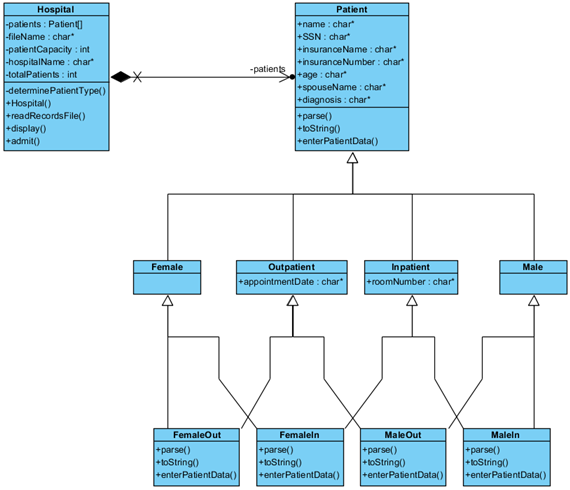
#include "Hospital.h"
#include "Patient.h"
void main (void)
{
Hospital general("Waterbury General Hospital", 500), memorial("Smithtown Memorial Hospital", 225);
MaleIn m1("Sarah", 3, "Jones", Patient::M + Patient::IN, Patient::CODE13);
MaleOut m2("Jane", Date(12,15,98), "Evans", Patient::M + Patient::OUT, Patient::CODE11); FemaleIn f1("Bob", 2, "Simmons", Patient::F + Patient::IN, Patient::CODE7); general.admit(m1);
general.admit(m2); memorial.admit(f1);
general.display(Patient::ALL); memorial.display(Patient::ALL);
general.display(Patient::IN); memorial.display(Patient::OUT + Patient::M);
}
Attachment:- Waterbury Hospital patients records.csv