Reference no: EM13849494
Part 1:
How to validate data
1. Code a try/catch statement that catches and handles invalid data entered by the user. The code should prompt the user to enter a quantity and then retrieve the value the user enters and store it in an int variable named quantity. If the value the user enters can't be converted to an integer, discard the value, display an error message indicating that the entry was invalid and that the user should enter another value, and repeat the while statement that contains this code (you don't need to code the while statement). Assume that a Scanner object named sc has already been declared and initialized.
2. Write code that prompts the user to enter a quantity and then checks if the user entered a value at the console and if that value can be converted to an int type. If the user enters a valid int, retrieve the value and store it in an int variable named quantity. Otherwise, discard any data the user entered, display an error message indicating that the entry was invalid and that the user should enter another value, and repeat the while statement that contains this code (you don't need to code the while statement). Assume that a Scanner object named sc has already been declared and initialized.
3. Given an String object named shippingType, code an if statement that sets a double variable named shippingBase to 4.95 if shippingType is equal to "FEDEX" and to 5.95 if shippingType contains any other value. Include the code necessary to prevent a NullPointerException.
4. Code a while statement that prompts the user to enter a quantity until the value of a boolean variable named isValid is true. To be valid, the value must be an integer between 1 and 99. If it isn't, display an appropriate error message. Assume that a Scanner object named sc has already been declared and initialized. Be sure to discard any unusable data the user enters at the console.
5. Code a public method named getInt that accepts a Scanner object and a String object that represents the prompt to be displayed and returns the int value the user enters at the console. This method should prompt the user for an integer value until a valid value is entered. Each time an invalid value is entered, an appropriate error message should be displayed. Be sure to discard any unusable data the user enters at the console.
6. Code a public method named getIntWithinRange that accepts a Scanner object, a String object that represents the prompt to be displayed at the console, an int that represents the minimum acceptable value, and an int that represents the maximum acceptable value. This method should contain a while loop that's executed until the user enters a valid integer within the specified range. To get a valid integer, it should call the getInt method described in problem 5-5. If that integer falls outside the specified range, it should display an appropriate error message. When a valid value is entered, the method should return it to the calling method.
Part 2:
7 Choose any four exercises from the following below to complete with meaningful comments
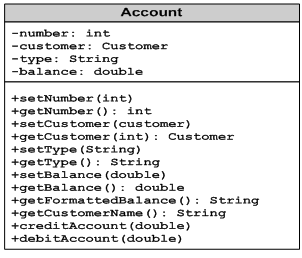
1. (Refer to class diagram 6-1.) Declare the Account class and its instance variables. Then, code a constructor that assigns default values to these variables. The default value you assign to the customer variable should prevent a NullPointerException. Write this code as concisely as possible.
2. (Refer to class diagram 6-1.) Code a constructor for the Account class that assigns values to the instance variables based on the values that are passed to it. The parameters of this constructor should be given the same names as the instance variables.
3. (Refer to class diagram 6-1.) Code a constructor for the Account class that assigns values to the instance variables based on the values that are passed to it. The parameters of this constructor should be given the names n, c, t, and b respectively. Write this code as concisely as possible.
4. (Refer to class diagram 6-1.) Code a constructor for the Account class that assigns values to the number, type, and balance instance variables based on the values that are passed to it, and assigns a value to the customer instance variable by calling the getCustomer method. The parameters of this constructor should be given the same names as the instance variables. The getCustomer method should accept the number variable as a parameter and return a Customer object. Write this code as concisely as possible.
5. (Refer to class diagram 6-1.) Write the code for the getType method. This method should simply return the value of the type instance variable.
6. (Refer to class diagram 6-1.) Write the code for the setType method. This method should simply set the value of the type instance variable to the value that's passed to it.
7. (Refer to class diagram 6-1.) Write the code for the getCustomerName method. This method should get a customer's name from the customer instance variable by calling a method of the Customer class named getName that returns the name instance variable of the Customer object. Write this method as concisely as possible.
8. (Refer to class diagram 6-1.) Write the code for the getFormattedBalance method. This method should return the value of the balance instance variable formatted as currency with two decimal places.
9. (Refer to class diagram 6-1.) Write the code for the creditAccount and debitAccount methods. The creditAccount method should add the amount that's passed to it to the balance instance variable, and the debitAccount method should subtract the amount that's passed to it from the balance instance variable.
10. (Refer to class diagram 6-1.) Assume that the Account class includes an additional instance variable named lastTranDate. Write two methods that overload the creditAccount and debitAccount methods that accept and update this instance variable. (Hint: You can use a variable created from the Date class to store a date.)
11. (Refer to class diagram 6-1.) Given a constructor that accepts values for the number, type, and balance instance variables and assigns those values to the instance variables, code another constructor that calls this constructor and passes default values to it.
12. (Refer to class diagram 6-1.) Code a statement that creates an instance of the Account class using the constructor that doesn't accept any parameters. Store the object that's created in a variable named account.
13. (Refer to class diagram 6-1.) Code a statement that creates an instance of the Account class using the constructor that accepts values for the number, type, and balance instance variables. Assume that variables named number, type, and balance have already declared and assigned values in the class that's creating the object. Store the object that's created in a variable named account.
14. (Refer to class diagram 6-1.) Code a statement that sets the value of the type instance variable by calling the setType method. Assume that an instance of the Account class has already been created and stored in a variable named account and that a variable named type that stores the account type has already been declared and assigned a value in the calling class.
15. (Refer to class diagram 6-1.) Code a statement that calls the getFormattedBalance method and stores the String object that's returned in a variable named formattedBalance. Assume that an Account object named account has already been created in the calling class.
16. (Refer to class diagram 6-1.) Code a statement that creates a String object named message that consists of the string "Customer name: " followed by the customer's name. Assume that an Account object named account has already been created in the calling class.
17. (Refer to class diagram 6-1.) Declare a static field named tranCount that can be used to track the number of debits and credits that are posted to all of the account objects created from the Account class. Assume that this field will be updated only from the creditAccount and debitAccount methods of the class.
18. (Refer to class diagram 6-1.) Code a method named getTranCount that can be used by other classes to get the value of the tranCount field.
19. (Refer to class diagram 6-1.) Code a statement that calls the static getTranCount method to get the number of transactions that have been posted. Store the value that's returned by this method in an int variable named count.
20. Code a statement that declares a static constant named SALES_TAX_PERCENT. This constant should be declared with the double data type and given an initial value of .0775. It should also be declared so that it can be accessed directly from other classes.
21. Code a statement that uses a static constant named SALES_TAX_PERCENT that's stored in a class named Sales. This statement should use this constant to calculate the sales tax for a double variable named subtotal and store it in another double variable named salesTax.
Part 3:
Inheritance hierarchy
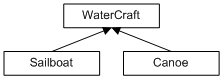
1. (Refer to inheritance hierarchy 7-2.) Write the code for the WaterCraft class. This class should include instance variables named id (int), type (string), and length (double) that can only be accessed by the class. Code a single constructor that assigns default values to the instance variables, and code get and set methods for the instance variables.
2. (Refer to inheritance hierarchy 7-2.) Write the code for the Sailboat class. This class should extend the WaterCraft class by adding an instance variable named sails (int) and get and set methods for this variable. It's constructor should initialize the instance variables defined by the WaterCraft class by calling the constructor of that class, and it should assign a default value to the sails instance variable.
3. (Refer to inheritance hierarchy 7-2.) Assume that you want to add a static variable named count (int) to the WaterCraft class that can be accessed by the Sailboat class but not by other classes. Code the declaration for this variable so that it's initialized to a value of 0.
4. (Refer to inheritance hierarchy 7-2.) Code a public method for the WaterCraft class that overrides the toString method of the Object class. This method should return a string that contains the id, type, and length variables in this format:
ID: 1234
Type: Sailboat
Length: 19'
5. (Refer to inheritance hierarchy 7-2.) Code a public method for the Sailboat class that overrides the toString method of the WaterCraft class. This method should return a string that contains the information returned by the toString method of the WaterCraft class appended with the number of sails in this format:
Sails: 3
6. (Refer to inheritance hierarchy 7-2.) Assume that you have created a Sailboat object named s and assigned it to a WaterCraft object named w. Write a statement that will print the type of the WaterCraft object to the console.
7. (Refer to inheritance hierarchy 7-2.) Assume that you have created a WaterCraft object named w. Write an if clause that will check if the object is a Sailboat object, using the easiest technique available.
8. (Refer to inheritance hierarchy 7-2.) Code an equals method for the WaterCraft class that overrides the equals method of the Object class. This method should accept an Object object and return a boolean that indicates if the object is a WaterCraft object with the same id, type, and length values as the current object.
9. (Refer to inheritance hierarchy 7-2.) Assume that the WaterCraft class has been defined as abstract. Add an abstract method to this class named getDisplayText that accepts no parameters and returns a string.
10. (Refer to inheritance hierarchy 7-2.) Write the code for a method in the Sailboat class that overrides the abstract getDisplayText method in the WaterCraft class (see problem 9). The value that's returned by this method should include the length and type fields from the WaterCraft class and the sails field from the Sailboat class formatted like this:
19.0' Sailboat with 3 sails
Declare this method so that it can't be overridden by any class that inherits this class.
Class diagram 8-1
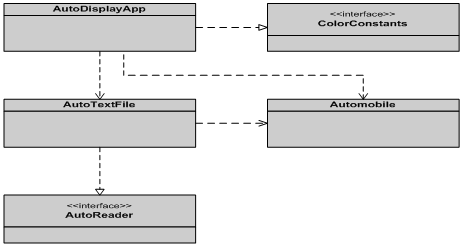
1. (Refer to class diagram 8-1.) Write the code for the ColorConstants interface. This interface should declare constants for the colors red, white, and black and assign the int values 1, 2, and 3 to those constants.
2. (Refer to class diagram 8-1.) Write the code for the AutoReader interface. This interface should declare two methods. The first one, named getAuto, should accept an id and return an Automobile object for the automobile with that id. The second one, named getAutosByColor, should accept an integer that represents a color in the ColorConstant interface and return a string that contains the ids of all the automobiles that are that color.
3. (Refer to class diagram 8-1.) Write the code for the AutoTextFile class. Simulate the getAuto method by creating a new Automobile object with the id that's passed to the method. Don't worry about setting the values of the other instance variables. Simulate the getAutosByColor method by using a switch statement to return a string of numbers depending on which color is specified (you choose the numbers). If an invalid color number is specified, return a null.
4. (Refer to class diagram 8-1.) Write code for the AutoDisplayApp class that uses the getAuto method defined by the AutoTextFile class to get an Automobile object with a specified id and then prints a message with the id in this format:
Auto 1234 read successfully.
Assume that a long variable named id has already been declared and assigned a value.
5. (Refer to class diagram 8-1.) Write code that uses the getAutosByColor method defined by the AutoTextFile class to get the ids for all the autos with a specified color. If one or more autos are found, a message should be printed in this format:
Red automobiles: 1,4,7,10
Otherwise, this message should be printed:
There are no automobiles with that color.
Assume that an int variable named color has already been declared and assigned a color value. Use the constants in the ColorConstants interface in your code.
Class diagram 8-2
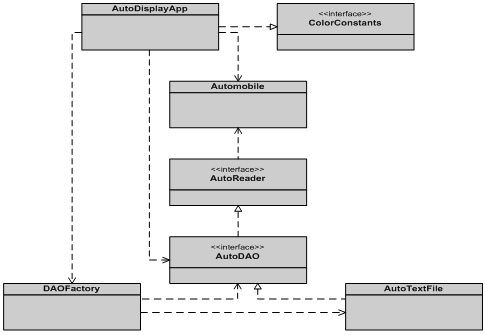
6. (Refer to class diagram 8-2.) Write the code for the AutoDAO interface. This interface should inherit the AutoReader class but not define any constants or methods.
7. (Refer to class diagram 8-2.) Write the code for the DAOFactory class. This class should contain a single static method named getAutoDAO that returns an AutoDAO object that is an instance of the AutoTextFile class. Note that the AutoTextFile implements the AutoDAO interface.
8. (Refer to class diagram 8-2.) Write code that uses the DAOFactory class to get an autoDAO object. Then, use that object to call the getAuto method defined by the AutoTextFile class to get the auto with a specified id. Assume that an int variable id has already been declared and assigned a value.
Class diagram 8-3
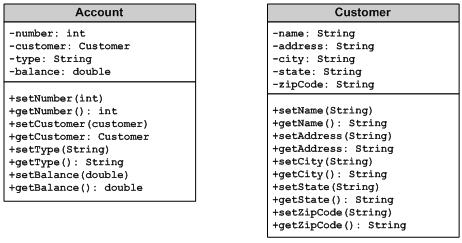
9. (Refer to class diagram 8-3.) Write the code for the Customer class so that it can be cloned. You don't need to code a constructor or get and set methods.
10. (Refer to class diagram 8-3.) Write code that creates a Customer object named c1 and then clones it and stores the clone in an object named c2.
11. (Refer to class diagram 8-3.) Write the code for the Account class so that it can be cloned. You don't need to code a constructor or get and set methods, but you should assume that they are coded for each instance variable. You can also assume that the Customer class implements the Cloneable interface.
Part 4:
Directory structure 9-1
invoice
InvoiceApp.java
murach
business
Invoice.java
LineItem.java
Product.java
database
ProductDB.java
presentation
Validator.java
1. (Refer to directory structure 9-1.) Code a statement that will include the ProductDB class in the package indicated by the directory structure.
2. (Refer to directory structure 9-1.) Code the statement that's required for you to use the ProductDB class in another class.
3. Code an HTML tag that will display the text "Invoice" in a monospaced font.
4. Code a javadoc tag that will display this parameter description:
salesTax - A double for the sales tax
5. Code the declarations for two classes named Invoice and LineItem within the same file. The Invoice class should be declared first.
6. Code the declarations for two classes named Invoice and LineItem within the same file. The LineItem class should be nested within the Invoice class.
Enumeration 9-1
Consider an enumeration named Terms that contains three constants named NET_30_DAYS, NET_60_DAYS, and NET_90_DAYS.
7. (Refer to enumeration 9-1.) Write the code for the Terms enumeration.
8. (Refer to enumeration 9-1.) Code a statement that assigns the NET_60_DAYS constant of the Terms enumeration to a variable named terms.
9. (Refer to enumeration 9-1.) Add a method to the Terms enumeration that overrides the toString method of the Object class. This method should return a string that specifies the terms for an invoice like this:
Net due 30 days
10. (Refer to enumeration 9-1.) Code a statement that will make the constants in the Terms enumeration available to a class without qualification. Assume that the enumeration is stored in the murach.business package.
Chapter 11 Questions
Complete any ten questions from the ones below:
1. Code the declaration for an array of ints named counts.
2. Declare and instantiate an array of Strings named titles that contains 4 elements.
3. Code a statement that assigns the value "Star Wars" to the first element of an array of strings named titles.
4. Code a statement that assigns a Customer object to the third element of an array of Customer objects named customers. Assume that the Customer class contains a constructor that assigns default values to all of the instance variables.
5. Code a statement that creates an array of doubles named miles and assigns the values 27.2, 33.5, 12.8, and 56.4 to its elements.
6. Given a one-dimensional array named monthSales with 12 double values, code a for loop that calculates the total of all the sales and stores it in a variable named yearSales.
7. Given a one-dimensional array named monthSales with 12 double values, code an enhanced for loop that calculates the total of all the sales and stores it in a variable named yearSales.
8. Given a one-dimensional array of strings named ratings, code a statement that uses the fill method of the Arrays class to assign the value "Not rated" to each element of the array.
9. Given a one-dimensional array of doubles named mileages, code a statement that uses the fill method of the Arrays class to assign the value 25.0 to the first 6 elements of the array.
10. Given two arrays named numbers1 and numbers2, code an if clause that tests if the two arrays are of the same type and have elements with the same values.
11. Given an array of strings named vendors, code a statement that uses the sort method of the Arrays class to sort the elements in alphabetical order.
12. Given an array of strings named vendors that has been sorted into alphabetical sequence, code a statement that uses the binarySearch method of the Arrays class to search for an element with the value "PG&E". Store the value that's returned in an int variable named index.
13. Given the following code for a class named Movie, implement the Comparable interface for this class by coding the compareTo method. This method should compare the current Movie object with the Movie object that's passed to it based on the id field.
public class Movie implements Comparable
{
private int id;
private String title;
public Movie(int id, String title)
{
this.id = id;
this.title = title;
}
public int getId()
{
return id;
}
public String getTitle()
{
return title;
}
}
14. Code a statement that creates a rectangular array of ints named values that contains 3 rows and 4 columns.
15. Code a statement that assigns the value 100 to the element in the second row and fourth column of an array of ints named values.
16. Code a statement that declares a rectangular array of strings named foods that has two rows and three columns and assigns values to each element. Assign the values "carrots", "broccoli", and "corn" to the elements in the first row, and assign the values "apples", "oranges", and "bananas" to the elements in the second row.
17. Given a rectangular array named sales with 5 rows and 12 columns of double values, code a for loop that calculates the total of the sales in each element of the array and stores it in a variable named totalSales.
18. Given a rectangular array named sales with 5 rows and 12 columns of double values, code an enhanced for loop that calculates the total of the sales in each element of the array and stores it in a variable named totalSales.
19. Write code that creates a jagged array of ints named values that contain 3 rows. The first row should contain 10 columns, the second row should contain 20 columns, and the third row should contain 30 columns.
20. Code a statement that declares a jagged array of strings named foods that have two rows. The first row should contain two elements with the values "carrots" and "corn", and the second row should contain three elements with the values "apples", "oranges", and "bananas".
Part 5:
Choose three from each from Array list below to complete:
Array list 11-1
Assume that you want to create an array list named enrollments that will hold the following integer values:
25
32
27
29
1. (See array list 11-1.) Code a statement that declares and initializes the enrollments array list.
2. (Refer to array list 11-1.) Code statements that add the four values to the enrollments array list.
3. (Refer to array list 11-1.) Code a for loop that prints each element of the enrollments array list on a separate line.
4. (Refer to array list 11-1.) Code a statement that changes the value of the second element of the array list to 30.
5. (Refer to array list 11-1.) Code a statement that deletes the third element from the array list.
6. (Refer to array list 11-1.) Code a statement that prints the index of the element with a value of 29.
7. (Refer to array list 11-1.) Code a statement that prints the elements of the enrollments array list in this format:
[25, 30, 29]
Linked list 11-1
Assume that you want to create a linked list named subjects that will hold the following string values:
Mathematics
Science
English
History
8. (Refer to linked list 11-1.) Code a statement that declares and initializes the subjects linked list.
9. (Refer to linked list 11-1.) Code statements that add the four values to the subjects linked list.
10. (Refer to linked list 11-1.) Code a statement that adds an element with the value "PE" following the second element of the subjects linked list.
11. (Refer to linked list 11-1.) Code a statement that changes the value of the first element of the subjects linked list to "Algebra".
12. (Refer to linked list 11-1.) Code a statement that gets the value of the first element of the subjects linked list and stores it in a string variable named firstSubject.
13. (Refer to linked list 11-1.) Code a statement that deletes the last element of the subjects linked list and stores the value of that element in a string variable named lastSubject.
14. (Refer to linked list 11-1.) Code an enhanced for loop that prints each element of the subjects linked list on a separate line.
Stack 11-1
Assume that you want to define a class named GenericStack that you can use to work with stacks that contain items of varying types. (A stack is like a queue except that the last item that's added to it is the first one that's retrieved.) The stack will include three methods: the push method will add an item to the beginning of the stack; the pop method will return the first item in the stack and remove it from the stack; and the size method will return the number of items in the stack.
15. (Refer to stack 11-1.) Code the declaration for the GenericStack class using a type variable with the name E.
16. (Refer to stack 11-1.) Code a statement that declares and initializes a linked list named stack that will hold the items of a GenericStack object. This linked list should not be accessible from outside the class.
17. (Refer to stack 11-1.) Code the push method of the class.
18. (Refer to stack 11-1.) Code the pop method of the class.
19. (Refer to stack 11-1.) Code the size method of the class.
20. (Refer to stack 11-1.) Code a statement that declares and initializes a GenericStack object named shipNoStack that can hold integer values.
21. (Refer to stack 11-1.) Code statements to add the values 1, 2, and 3 to the shipNoStack object.
22. (Refer to stack 11-1.) Code a statement that removes the first item from the shipNoStack object and prints its value at the console.
23. (Refer to stack 11-1.) Code a statement that prints the number of items in the stack at the console.
Hash map 11-1
Assume that you want to create a hash map named cars with the following keys and values:
Key
|
Value
|
2378
|
1999 Chevy Lumina
|
1796
|
2001 Dodge Durango Sport
|
3282
|
2000 Pontiac Grand Prix
|
1967
|
2003 Honda Accord LX
|
24. (Refer to hash map 11-1.) Code a statement that declares and initializes the cars hash map.
25. (Refer to hash map 11-1.) Code a statement that adds the first element to the cars hash map.
26. (Refer to hash map 11-1.) Code a statement that gets the description of the car whose key value is 1796 and stores it in a variable named description.
27. (Refer to hash map 11-1.) Code an enhanced for loop that prints each element of the cars hash map on a separate line in this format:
2378: 1999 Chevy Lumina
ArrayList 11-2
Assume that you want to create an untyped collection named departments from the ArrayList class. This collection will hold objects created from a class named Department that contains a constructor that accepts values for its id, name, and manager instance variables. The objects in this collection should have the following values:
id
|
name
|
manager
|
10
|
Electronics
|
John Thompson
|
20
|
Small appliances
|
Alyssa Keenan
|
30
|
Computers
|
Kimberly Lund
|
28. (Refer to array list 11-2.) Code a statement that declares and initializes the departments array list.
29. (Refer to array list 11-2.) Code statements to add the Department objects to the departments array list.
30. (Refer to array list 11-2.) Code a statement to get the first object in the array list and store it in a Department variable named dept.
ArrayList 11-3
ArrayList averages = new ArrayList();
31. (Refer to array list 11-3.) Code a statement to add the double value 34.5 to the averages array list.
32. (Refer to array list 11-3.) Code a statement to get the value of the first element of the averages array list and store it in an int variable named avg.