Reference no: EM131194127
Overall Assignment
For this assignment, you are to write a MatLab program to perform a two-dimensional Finite Element Analysis ( FEA ) of a computer motherboard, and to write a short memo describing your findings.
Background: Finite Element Analysis
There are many situations in which engineers need to determine properties ( e.g. temperature, pressure, concentrations, etc. ) of the interior of a defined space, given certain boundary conditions describing the properties at the edges of the space and/or certain internal conditions. Finite Element Analysis is a technique in which the interior space is broken up into very small ( yet finite ) elements, and then analyzing how those elements interact with each other. The following images from Fluent Inc show some of the capabilities of commercial finite element analysis software:
The general approach to FEA is to first estimate the internal conditions somehow, and to then successively refine the estimation until the "answer" is obtained, to within some acceptable level of approximation, i.e. until the refinement step does not cause any appreciable change in the estimation.
For our purposes, a very simple ( and not very efficient ) technique is to first initialize all the elements to some ( arbitrary ) value, and to then refine the estimate by setting the new value of each element to be the average of the current values of its immediate neighbors. So in the diagram below, element A would be set to the average of elements B, C, D, and E. ( In the general case FEA is performed in 3 dimensions of arbitrary geometry, but for this assignment we will be restricting ourselves to rectangular spaces in two dimensions. )
Anew = ( Bcurrent + Ccurrent + Dcurrent + Ecurrent ) / 4.0
Application Domain: Computer Motherboards
One of the big problems facing modern circuit board designers is that some components generate a lot of heat and therefore get quite hot, while other components on the circuit boards may be sensitive to high temperatures. In the particular domain of computer motherboards, modern CPUs and GPUs can get so hot that they have to be liquid cooled in some cases. Therefore, motherboard designers frequently experiment with different layouts of components, to try to minimize the impact of hot components on sensitive ones
For this assignment, you are to write a MatLab program that analyzes and plots the temperature distribution across a rectangular motherboard, given the motherboard and CPU dimensions, the ambient temperature of the edges of the motherboard, and the temperature and location of the CPU on the motherboard. For the base assignment we will further limit our analysis to a single hot component ( CPU ), lined up parallel to the edges of the motherboard. ( I.e. no chips mounted at angles like some of the examples above. )
( In practice the FEA of computer systems is a 3-dimensional and dynamic one, taking into account the locations of fans and vents as well as electrical components, but we will restrict ourselves to the two-dimensional static case for this assignment. )
Program Implementation
- Your MatLab program should ask the user for the following information. The values shown in parentheses are typical:
o The width and height of the motherboard, in inches. ( 10.0, 8.0 )
o The width and height of the CPU. ( 2.0, 2.0 )
o The location of the lower left corner of the CPU. ( 6.0, 2.0 ) ( The coordinate system should have 0, 0 at the lower left corner of the motherboard, with X increasing to the right and Y increasing to the top. )
o The temperature of the CPU. ( 50 degrees C or 120 degrees F )
o The ambient temperature, which defines the temperature along all edges of the motherboard. ( 25 degrees C or 75 degrees F )
o The dimension of an element, which will be the same in both X and Y directions. ( 1.0 initially, then 0.1 when the program is working. )
o The tolerance to be used for a stopping condition. ( 1.0E-03 )
o Maximum limit on iterations. ( 100 initially, then a larger number as needed. )
- Your program should then create TWO arrays the correct size to represent the motherboard with the desired resolution, and should set the boundary conditions ( the temperatures along the edges of the board and where the CPU sits ) for each of the arrays. In order to speed things up, the remainder of the array should be initialized with the average of the CPU temperature and the ambient temperature. ( It will probably be most efficient to set the entire array to a constant average temperature and then set the boundary conditions. ) These arrays then each contain a ( very poor ) estimate of the temperatures at every element on the motherboard.
- Your program will then repeatedly refine the estimate of the temperatures, until the maximum number of iterations is reached or until the estimate quits changing to within the desired tolerance.
o Two arrays are needed because you will read the old ( current ) values from one array while building an updated estimate in the other array. Then either copy the updated values back into the original array to repeat the process, or swap the roles of the two arrays for the next estimate refinement. ( I.e. either update array A to array B and then copy from B back to A, or else update from A to B and then update from B to A. )
o The boundary conditions should not be changed by the estimate updating procedure, which give you two options: (1) Be careful not to change the elements corresponding to the boundary conditions during the estimate updating process, or
(2) go ahead and change them along with the other elements, and then reset the boundaries back after the update. For this assignment, it is probably easiest to use method (1) for the edge boundaries and method (2) for the CPU boundary.
o For the stopping condition, determine the maximum of the absolute value of the change of any one element during the estimate update, and compare it against the stated tolerance. If the biggest such change is less than the tolerance, ( or if the maximum number of iterations has been reached ), then stop updating and report the final results.
Incremental Development
For any large programming task, it is best to develop and test the code in small incremental steps, rather than trying to do it all at once. For this assignment, it is recommended that you initially plot your matrix as soon as you have initialized it, and then set a very low maximum number of iterations and re-plot the matrix after each iteration. When your program is running you won't want to re-print the graph as frequently, as it will slow down the simulation.
Program Input
The program should ask the user for the values specified above.
Program Output
Your program should first print out your name and ID, and explain to the user what the program does. Then it should report problem results to the screen in the form of a well-labeled two- dimensional color plot, ( including a colorbar - see Matlab help for details. )
You should also write a short one to two-page "memo" outlining the results of your simulation, including images from your simulation. Please use Microsoft Word to write this memo, and hand in the Word format document with your other files. For this memo assume the scenario that you are an engineer working for a company that makes computer motherboards, and your boss has asked you to perform a temperature analysis on the company's latest motherboard design. Your memo should be addressed to the Head of Development and Research ( who may not be an engineer ) at Mélange Computer Industries, Inc.
What to Hand In:
1. Your code, including the requested memo and a readme file, should be handed in electronically using Blackboard. No hard copy is required for this assignment.
2. The purpose of the readme file is to make it as easy as possible for the grader to understand your program. If the readme file is too terse, then (s)he can't understand your code; If it is overly verbose, then it is extra work to read the readme file. It is up to you to provide the most effective level of documentation.
3. If there are problems that you know your program cannot handle, it is best to document them in the readme file, rather than have the TA wonder what is wrong with your program. In particular, if your program does not complete all of the steps outlined above, then you should document just exactly which portions of the project your program does accomplish.
4. No hard copy is required for this assignment. However you may hand in hard copy to the TA by the submission deadline if you have materials which are difficult or impossible to submit electronically.
5. Make sure that your name and your CS account name appear at the beginning of each of your files. Your program should also print this information when it runs.
Notes Regarding "Pcolor"
One of the tools you may want to use on this assignment is "pcolor", which has some interesting characteristics you should be aware of.
- If shading is set to "interp" (interpolated), then the colors within a cell are gradiated from corner to corner, and all the data values in the array are used.
- If shading is set to "faceted", which is the default, then each cell will be a constant color based on the data at one corner, and the last row and column are ignored.
- Pcolor will also take arguments specifying the axes, as opposed to default indices.
- For example, the following data matrix yielded the following plot as a starting point. ( Note that row 1 of the data appears on the top of the printed table, but at the bottom of the plotted image, and that the "extra" row and column of the data in the table are not represented in the plot. )
data =
|
|
25
|
25
|
25
|
25
|
25
|
25
|
25
|
25
|
25
|
25
|
25
|
25
|
0
|
0
|
0
|
0
|
0
|
0
|
0
|
0
|
25
|
25
|
25
|
0
|
0
|
0
|
0
|
0
|
50
|
50
|
0
|
25
|
25
|
25
|
0
|
0
|
0
|
0
|
0
|
50
|
50
|
0
|
25
|
25
|
25
|
0
|
0
|
0
|
0
|
0
|
0
|
0
|
0
|
25
|
25
|
25
|
0
|
0
|
0
|
0
|
0
|
0
|
0
|
0
|
25
|
25
|
25
|
0
|
0
|
0
|
0
|
0
|
0
|
0
|
0
|
25
|
25
|
25
|
25
|
25
|
25
|
25
|
25
|
25
|
25
|
25
|
25
|
25
|
25
|
25
|
25
|
25
|
25
|
25
|
25
|
25
|
25
|
25
|
25
|
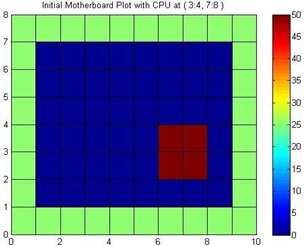
Optional Enhancements:
It is course policy that students may go above and beyond what is called for in the base assignment if they wish. These optional enhancements will not raise any student's score above 100 for any given assignment, but they may make up for points lost due to other reasons.
- Make use of Matlab functions, to break the overall problem down into steps that can be written and performed separately.
- Experiment with different plotting options, including 3-D plots, subplots, and specialized colormaps. Remember that your goal is not to just make something that looks cool, but rather to convey the important temperature information to the circuit board designer as efficiently and effectively as possible.
- Keep a history of the convergence of your algorithm, i.e. how it changes with each iteration, and display these results as a plot. Can you display this as a changing plot as the program runs without slowing it down too much? How much difference does it make to have a good starting point estimate?
- Allow the user to place multiple "hot" chips on the motherboard, possibly including one or more that are actually cooler than the ambient temperature instead of hotter ( e.g. a fan. )
- Allow users to place chips at angles, or include non-rectangular geometries.
- Use the program to perform comparative analyses, to determine the "best" place to put any given CPU on any given motherboard. How do you choose to define "best"? Can you get Matlab to find the "best" place for you, or do you need to run the program repeatedly and evaluate for yourself which is the "best" location? Include your results as a separate section of your memo. ( A multi-plot figure using subplot may be a good way to compare alternatives. If you determine a "value" function for determining which location is "best", you can show the "value" of each position, possibly in a plot or chart.)
- Other enhancements that you think of - Check with TA for acceptability.