Reference no: EM13185763
In this question, you will test, using a backtracking algorithm, if a mouse can escape from a rectangular maze.
The backtracking algorithm helps the mouse by systematically trying all the routes through the maze until it either finds the exit or exhausts all possible routes (and concludes that the mouse is trapped in the maze). If the backtracking algorithm finds a dead end, it retraces its path until it reaches a position from which there is an untried path. The backtracking algorithm always tries all directions from any position, and always in the same order.
The input to the algorithm is a maze with walls (represented by '1' characters) and open passage ways (represented by '0' characters). The starting position of the mouse is represented by 'm' and the exit from the maze by 'e'. Your program should read the maze in from a file, and the name of the file should be a command-line argument. The first line of the input will contain the number of rows and the number of columns in a maze. Thus, the input might look like the following:
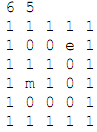
The maze will always have a wall around the outside, so you need not be concerned about the mouse falling off the maze as it explores all directions.
The backtracking algorithm keeps a stack of positions that are the beginnings of paths it has yet to try. From the current position, the algorithm pushes onto the stack any untried open neighboring positions (if there are any), always looking forward, backward, left and right from the current position. At each step, the algorithm pops the top position off the stack and pushes the untried neighboring positions onto the stack. The algorithm must mark each visited position with a period to avoid revisiting positions --- so that it will not
loop forever trying the same routes.
The backtracking algorithm works as follows:
read in the maze;
initialize the stack;
goalCell = the position of the exit in the maze;
startCell = the initial position of the mouse in the maze;
currentCell = startCell;
while currentCell is not the goalCell
mark currentCell as visited;
push onto the stack the unvisited open neighbours of currentCell;
if the stack is empty
the mouse is trapped: we tried all routes and failed to find the exit;
else
pop a cell from the stack and make it currentCell;
end while;
the mouse can escape the maze: we reached the goal cell.
Because the backtracking algorithm needs a stack, you should implement a stack class (or you can use that from COSC 2006). Each item in the stack is the position of a cell in the maze --- that is, the row and column number of the cell.
Your program should print out the maze after each cell is visited, showing which cells have already been visited. Finally, your program must print out a message indicating whether the exit was found or that no route to the exit could be found (the mouse is trapped).
To develop your program, you can use the input file testMaze.txt, which contains the above maze.
- Hand in your complete Java source code; and a copy of the results produced
- Upload your source code to CMS
- Demonstrate your program to TA before/on the due day
What would be the standard deviation
: A poll of 52 students found that 50% were in favor of raising tution to build a new math building. The standard deviation of this poll is 8%. What would be the standard deviation if the sample size were increased from 52 to 116?
|
State what is the mass percentage of iron
: What is the mass percentage of iron in a sample if titration of 1.2284g of the sample requires 57.91 mL of .1018M of Ce(NH4)2(NO3)6 for complete reaction?
|
How many hamburgers were sold on friday
: On Friday, a local hamburger shop sold a combined total of 416 hamburgers and cheeseburgers. The number of cheeseburgers sold was three times the number of hamburgers sold. How many hamburgers were sold on Friday?
|
An oxcart for a dowry
: In "An Oxcart for a Dowry" by . Wang Zhenhe. Ahao is an unusual female character who does not embody the characteristics of good womanhood. Please point out at least three of her subversive behaviors and explain if her doings are completely wrong.
|
Problem on backtracking algorithm
: Your program should print out the maze after each cell is visited, showing which cells have already been visited. Finally, your program must print out a message indicating whether the exit was found or that no route to the exit could be found
|
What is an algebraic expression for the area of the garden
: A gardener wants to create a rectangular garden with length of 9x - 5y ft. and width of x - 6y ft. What is an algebraic expression for the area of the garden? Be sure to multiply this out, express in simplest correct mathematical form, and include..
|
What is the speed of the current
: A boat can travel 46 mph in still water. If it travels 270 miles with the current in the same length of time it travels 190 miles against the current, what is the speed of the current?
|
Explain expect a change in cooperativity
: A mutant hemoglobin has His146 of the beta chain replaced with a threonine residue. predict what the consequence of this mutation might be. specifically:
|
Explain a congressman asserts the argument
: Explain. A Congressman asserts the following argument: we need to produce more oil here in the United States. We consume 20 about million barrels of oil per day. If we promoted oil drilling in the United States, we could easily increase our domest..
|