Reference no: EM131239091
Question 1:
The objectives of this question are
(a) to introduce subclasses in a case study
(b) to apply the various OOP concepts
A delivery company, SendFast Pte. Ltd. has invited your team to build a computerized system for its operations. You have been tasked to develop a prototype based on the user specifications and the design proposed by your team. Your prototype will focus on only maintaining information for packs and deliveries for calculating delivery charges and reimbursement should a pack is lost. The delivery charge on the pack depends on the types and weight of items as well as the type of delivery and the region the pack is to be delivered to. The reimbursement depends on the value of the items and the type of delivery.
A pack to be delivered can contain one or more items. An item is either a standard item (requiring standard level of care) or a special care item which requires extra care as the item is fragile such as antique, art piece or chinaware. It costs more to deliver a special care item than a standard item.
The test data for your prototype for the items in 3 different packs is shown in Table 1:
Pack 1 item list
Item Type
|
Name
|
Quantity
|
Unit Weight (gram)
|
Unit Value (Sing$)
|
Special Care Item
|
Chinaware
|
10
|
247
|
395.00
|
Art piece
|
1
|
852
|
795.00
|
Pack 2 item list
Item Type
|
Name
|
Quantity
|
Unit Weight (gram)
|
Unit Value (Sing$)
|
Standard Item
|
Running shoes
|
2
|
535
|
79.90
|
Duffel
|
1
|
857
|
119.90
|
Pack 3 item list
Item Type
|
Name
|
Quantity
|
Unit Weight (gram)
|
Unit Value (Sing$)
|
Standard Item
|
Winter Glove
|
4
|
150
|
49.90
|
Ski Glove
|
3
|
227
|
179.90
|
Special Care Item
|
Snow Goggles
|
2
|
455g
|
89.90
|
Table 1
Note that the weight and value maintained in the system are for one unit of the item. Thus, in pack 1, the chinaware contributes 1247g x 10 = 2470g to the weight of the pack and $395.00 x 10 = $3950.00 to the value of the pack. The art piece contributes 852g x 1 = 852g to the weight of the pack and $795.00 x 1 = $795.00 to the value of the pack.
Charges on standard item is $0.99 per 150g or part of, with a discount of 10% should the charge be at least $500 and a discount of 5% should the charge be at least $200.
Charges on special care item is $2.03 per 100g or part of, with a base charge of $10. Thus charges on the chinaware is calculated as follows:
2470/100 = 24.70, round up to 25. 2.03 x 25 = 50.75
Adding base charge of $10, the charge for the chinaware is 50.75 + 10 = $60.75
The charge for art piece can be similarly calculated as 852/100, rounding to 9. 2.03 x 9 + 10 = $28.27.
SendFast Pte. Ltd. provides two kinds of delivery: normal and enhanced liability delivery. An enhanced liability delivery costs 12% more but in an event of loss, SendFast Pte. Ltd. will reimburse 80% of the value of the pack (combined value of items in the pack), compared to 5% reimbursement for normal delivery.
The test data for your prototype for the delivery for the 3 different packs is shown in Table 2:
Pack
|
Delivery Type
|
Region
|
From Address
|
To Address
|
Date to Ship
|
Date to Arrive
|
Pack 1
|
Enhanced Liability
|
4
|
21 Western Mall,
Singapore 345678
|
795 E Market St, Tucson AZ 84703, USA
|
1 Nov 2016
|
3 Nov 2016
|
Pack 2
|
Normal
|
2
|
10 Business Park,
Singapore 123456
|
75 Kg Ramal Luar, 43000 Kajang, Selangor, Malaysia
|
30 Oct 2016
|
31 Oct 2016
|
Pack 3
|
Enhanced Liability
|
3
|
12 Elane Road,
Singapore 234567
|
Tokyo Central Office, 2- 11, Yaesu Chuo-ku,
Tokyo 100-8774
|
1 Nov 2016
|
2 Nov 2016
|
Table 2
There are 4 regions that SendFast Pte. Ltd. will deliver to, regions 1, 2, 3 and 4 incur a factor of 1, 1.2, 1.4 and 1.55 respectively. Taking Pack 1 as an example, after the charges for the items it contains are calculated, a factor of 1.55 is applied since the pack is delivered to region
4. Thus the final charges for pack 1 is (60.75 + 28.27) x 1.55 (region 4) x 1.12 (enhanced) = $154.54. The reimbursement if pack 1 is lost is (3950 + 795) x 0.8 (enhanced) = $3796
Additional Note: SendFast Pte. Ltd. requires that all decimal numbers be displayed with 2 decimal places.
Study carefully the partial UML diagram given in Figure 1 which focuses on packs, the items they contain and the type of delivery they have. Use object-oriented programming concepts to differentiate between reuse by inheritance and reuse by composition, and then build the system for SendFast Pte. Ltd.
Class Item:
This abstract class models an item to be delivered. It has one abstract method getCharges() which its subclasses will implement.
Class StandardItem:
This class is a subclass of Item. The item requires standard level of care.
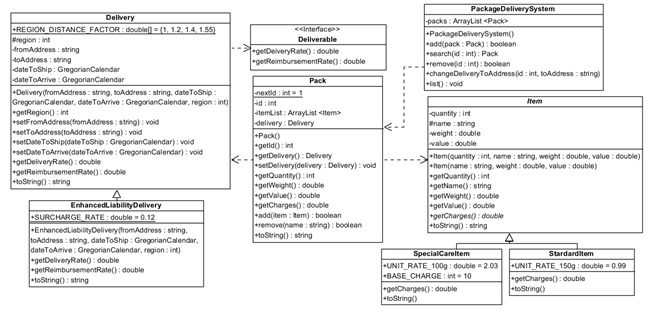
Figure 1
Class SpecialCareItem:
This class is a subclass of Item. The item requires special care and incurs higher charges.
Interface Deliverable:
A Deliverable must be able to return the delivery rate and the reimbursement rate.
Class Delivery:
This class implements Deliverable. It models a normal delivery for a pack.
Class EnhancedLiabilityDelivery:
This class is a subclass of Delivery. It models an enhanced liability delivery for a pack.
Class Pack:
This class models a pack to be delivered. A pack has a list of items and delivery.
Class PackageDeliverySystem:
This class models SendFast Pte. Ltd.
(a) Implement the Java class Item as an abstract class that includes the following:
- The instance variable(s), class variable(s) and constants (if any) according to the class diagram in Figure 1.
- Two constructors that will do all the necessary initializations. The second constructor gives quantity a default value of 1. Also, to avoid code duplication, make the first constructor call the second constructor.
- The get and set methods wherever required.
- The getCharges() method which is abstract.
- A toString() method that returns a string containing the values of all the attributes.
Here is an example in the required format:
(b) Implement the Java subclass StandardItem of Item that includes the following:
- The instance variable(s), class variable(s) and constants (if any) according to the class diagram in Figure 1.
- Two constructors that will do all the necessary initializations.
- The get and set methods wherever required.
- A getCharges() method that returns the calculated charges based on $0.99 per 150g or part of, with a discount of 10% should the charge be at least $500 and a discount of 5% should the charge be at least $200.
- A toString() method that returns a string containing the values of all the attributes. Here is an example in the required format:
(c) Implement the Java subclass SpecialCareItem of Item that includes the following:
- The instance variable(s), class variable(s) and constants (if any) according to the class diagram in Figure 1.
- Two constructors that will do all the necessary initializations.
- The get and set methods wherever required.
- A getCharges() method that returns the calculated charges based on $2.03 per 100g or part of, with a base charge of $10.
- A toString() method that returns a string containing the values of all the attributes. Here is an example in the required format:
(d) Implement the Java interface Deliverable that includes the following:
- A getDeliveryRate() method
- A getReimbursementRate() method
(e) Implement the Java class Delivery. This class implements the interface Deliverable
and includes the following:
- The instance variable(s), class variable(s) and constants (if any) according to the class diagram in Figure 1. The instance variable region stores the region the delivery is made to. The valid values for region are 1 to 4.
- A constructor that will do all the necessary initializations.
- The get and set methods wherever required
- A getDeliveryRate() method that returns one of the values stored in the REGION_DISTANCE_FACTOR array. The value returned depends on the instance variable region. If region stores 1, the first value in the array is returned, if region stores 2, the second value is returned, and so on.
- A getReimbursementRate() method that returns 5%.
- A toString() method that returns a string containing the values of all the attributes. Here is an example in the required format:
(f) Implement the Java subclass EnhancedLiabilityDelivery of Delivery that includes the following:
- The instance variable(s), class variable(s) and constants (if any) according to the class diagram in Figure 1.
- A constructor that will do all the necessary initializations.
- The get and set methods wherever required
- A getDeliveryRate() method that overrides the same method in its superclass by adding a surcharge rate of 12% to what is returned by getDeliveryRate() method in the superclass.
- A getReimbursementRate() method that returns 80%.
- A toString() method that returns a string containing the values of all the attributes.
Here is an example in the required format:
(g) Implement the Java class Pack that includes the following:
- The instance variable(s), class variable(s) and constants (if any) according to the class diagram in Figure 1. The pack id is auto-generated. The value is a running number starting from 1. Thus, the first created pack has id 1, the second pack has id 2 and so on.
- A constructor that does all the necessary initializations.
- The get and set methods wherever required.
- A getQuantity() method that returns the total number of the items in the pack.
- A getWeight() method that returns the sum of the weight of all the items in the pack.
- A getValue() method that returns the sum of the value of all the items in the pack.
- A getCharges() method that returns the sum of the charges of all the items in the pack.
- A add(Item) method that adds the item to the itemList of the pack.
- A remove(String) method that removes the item with the name matching the string from the itemList.
- A toString() method that returns a string containing the values of all the attributes.
Here is an example in the required format:
(h) Implement the Java class PackageDeliverySystem that includes the following:
- The instance variable(s), class variable(s) and constants (if any) according to the class diagram in Figure 1.
- A constructor that will do all the necessary initializations.
- The get and set methods wherever required.
- An add(Pack) method that adds a Pack to the collection of packs, packs.
- A search(int) method that searches the collection of packs, packs for a pack with the specified id and returns it. Return null if there is no such pack.
- An remove(int) method to remove a pack with the specified id from the collection of packs, packs.
- A changeDeliveryToAddress(int, String) method to search the collection of packs, packs for a pack with the specified id and change the toAddress of its delivery with the new address.
- A list() method that prints the collection of packs, packs in this format:
(i) Implement the Tester class in Java to test your implementations. You should include the following steps (in sequence) in the main method of this class:
- Create an instance of PackageDeliverySystem. Initialise the instance with the test data for vehicles and customers.
- Show the details of the packs already in the system.
- Change the toAddress of pack 1 to 793 E Market St, Tucson AZ 84703, USA
- Remove pack 2.
- Finally, show the details of packs already in the system.
The address of pack 1 should have been changed and pack 2 should not be displayed in the listing as shown here:
Submit the listings of all your classes together with screenshots showing the run of your program. Your screenshot must show your name as part of the program output.
Question 2:
The objective of this question is
(a) to use the Java exception handling facility in a program
(b) to define and use an exception class
(c) to write program code that will throw user-defined exceptions
After completing the prototype for SendFast Pte. Ltd., your project leader informed you to explore Exception which will be incorporated into the system. For your exploration, you are provided with this Trip class that your team mate has implemented.
import java.util.GregorianCalendar; import java.text.SimpleDateFormat; public class Trip {
private int region;
private GregorianCalendar date; private String address;
public Trip (int r, GregorianCalendar d, String a) { region = r; date = d; address = a;
}
public int getRegion () { return region; }
public GregorianCalendar getDate () { return date; } public String toString() {
SimpleDateFormat df = new SimpleDateFormat("EEE, dd MMM yyyy");
return df.format(date.getTime()) + "\tRegion: " + region + "\t" + address;
}
}
import java.util.GregorianCalendar; import java.text.SimpleDateFormat; public class Trip {
private int region;
private GregorianCalendar date; private String address;
public Trip (int r, GregorianCalendar d, String a) { region = r; date = d; address = a;
}
public int getRegion () { return region; }
public GregorianCalendar getDate () { return date; } public String toString() {
SimpleDateFormat df = new SimpleDateFormat("EEE, dd MMM yyyy"); return df.format(date.getTime()) + "\tRegion: " + region + "\t" + address;
}
}
Study carefully the partial UML diagram given in Figure 2 which focuses on couriers and trips they are to make.
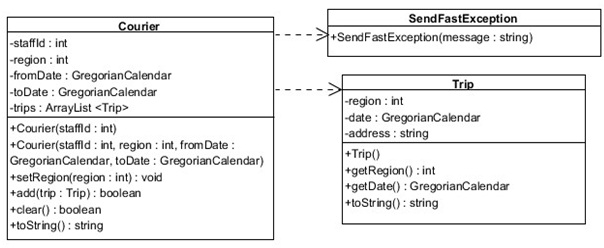
Figure 2
(a) Implement the Java class SendFastException class.
This class should be written as a subclass of Exception. An object of this class is created whenever your system raises an exception. The constructor of the SendFastException class sets the exception message to the message passed in.
(b) Implement the Java class Courier class with the following specifications :
- The instance variable(s), class variable(s) and constants (if any) according to the class diagram in Figure 2.
- Two constructors that will do all the necessary initializations.
o Courier(int) takes an integer value for initialising the staff id of the courier. The default values for the instance variables as as follows: the region assigned is 1, the period of attachment to region is 2 weeks, starting one week from the date of object creation.
o Courier(int, int, GregorianCalendar, GregorianCalendar)initialises all the instance variables. This constructor checks that fromDate is earlier than toDate. If not, the constructor must throw an SendFastException object with an appropriate message such as The end date cannot be before the start date.
Both constructors initialises the list of trips trips that must made in that period of attachment to the region, to empty. Again, to avoid code duplication, make the first constructor call the second constructor.
- A setRegion(int) method that allows the region is be changed only if list of trips trips is empty. Otherwise the method must throw an SendFastException object with an appropriate message such as Cannot change region as there are trips to be made!.
- A add(Trip) method that returns true if the trip is successfully added to the list. The method must throw an SendFastException object with an appropriate message for any of these cases:
o The trip to be added is in a different region from the region the courier is attached. Use an appropriate message such as Different region!.
o The date of the trip is after the last date of attachment. Use an appropriate message such as Past period!.
o The date of the trip is before the beginning of attachment. Use an appropriate message such as Before period!.
- A clear() method that returns true if all the trips in the trips are before or on the day the method is invoked, and so trips can successfully cleared of the trips. The method must throw an SendFastException object if there are one or more trips after the date the is to cleared. Use an appropriate message such as There are trips not completed yet, cannot clear trip collection!.
- A toString() method that returns a string containing the values of all the attributes. Here is an example in the required format:
(c) To test the Java classes SendFastException and Courier, implement the Java class Q2 with a main(String [] args)method . The method does the following:
- Creates a Courier object with staff id 1, region assigned 1, attached period from 1 December 2016 to 31 December 2016.
- Repeat the following steps 6 times to test all the possible cases:
o Prompt and read the information for a trip: region, date of trip and address.
o Create the trip and add to the courier.
o If the exception raised is caused by the trip being in a different region, attempt to clear the trips for the courier, change his region and add the trip. If the attempt is not successful, display the error message.
o If the exception raised is due to any other reason, display the error message.
o In every repetition, list the courier's details.
A sample run of the program is shown here with user input underlined:
Submit the listings of all your classes together with screenshots showing one run of the program. Your screenshot must show your name as part of the program output.
Question 3:
The objectives of this question are
(a) to use object-oriented programming concepts to build an application.
(b) to implement graphical user interface program in Java.
You are to implement a graphical user interface program with event handling.
(a) Design a simple graphical user interface (GUI) in Java that allows the user to enter an order of food items, each item had a name (string), a quantity (whole number) and unit price (decimal number), list the ordered items and then calculates payment.
Using suitable layout managers, implement a GUI that should be as close as possible to the one shown in Figure Q4.
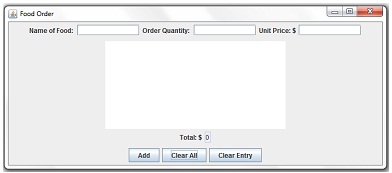
Figure Q4a
Figure Q4a shows how the GUI looks initially like, and also how it looks like when the Clear All button is pressed.
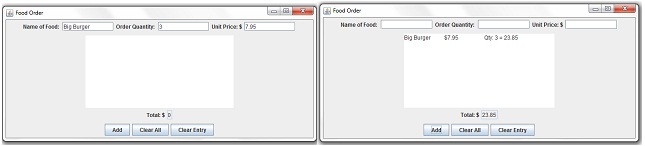
Figure Q4b
Figure Q4b shows how the GUI looks like before and after one food item has been entered in the relevant fields and the Add button is clicked.
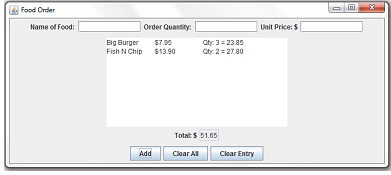
Figure Q4c
Figure Q4c shows how the GUI looks like after the second food item was added. Whenever a food item is added, the fields for data entry should be cleared.
The GUI has the following components:
- 3 editable text fields for item name, quantity and unit price, 1 non-editable text field for total and their corresponding labels.
- One text area for listing the items already in the order.
- 1 button labelled ADD which when pressed will update the ordered food list and the total, and clear the input fields.
- 1 button labelled Clear All which when pressed will clear the text fields and text area.
- 1 button labelled Clear Entry which when pressed will clear only the input text fields.
(b) Write a Tester class that creates and displays your GUI with size 700 by 300. The GUI must display your name and student name in the title bar. Set the GUI default close operation to exit the application and finally, make the GUI visible,
Include five screenshots in your submission, one to show the initial GUI and the other three to show the effect of pressing the various buttons.
Attachment:- ICT.rar