Reference no: EM131115315
Details
For this assignment, you are required to develop a Menu Driven Console Java Program to demonstrate you can use Java constructs including input/output via GUI dialogs, Java primitive and built-in types, Java defined objects, arrays, selection and looping statements and various other Java commands. Your program must produce the correct results.
The code for the menu and option selection is supplied and is available on the course website, you must write the underlying code to implement the program. The menu selections are linked to appropriate methods in the given code. Please spend a bit of time looking at the given code to familiarise yourself with it and where you have to complete the code. You will need to write comments in the supplied code as well as your own additions.
What to submit for this assignment
The Java source code:
o Mark.java
o ProcessMarks.java
If you submit the source code with an incorrect name you will lose marks.
A report including an UML diagram of your Mark class, how long it took to create the whole program, any problems encountered and screen shots of the output produced. (Use Alt-PrtScrn to capture just the application window and you can paste it into your Word document) You should test every possibility in the program. You should also include a couple of screen shots of your participation in the weekly tutorial forums.
Assignment Specification
You have completed the console program for collecting statistics for the entry of student marks. We are going to extend this application so the student names and marks can be stored in an array of objects.
The program will run via a menu of options, the file ProcessMarks.java has been supplied (via the Moodle web site) which supplies the basic functionality of the menu system.
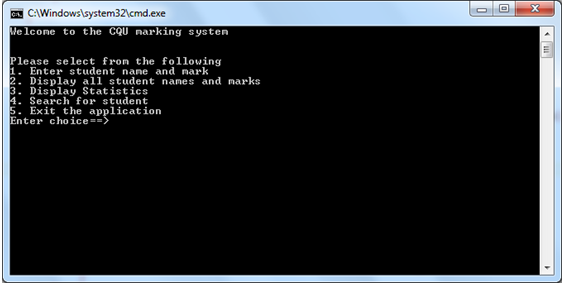
Look at the code supplied and trace the execution and you will see the menu is linked to blank methods (stubs) which you will implement the various choices in the menu.
Mark class
First step is to create a class called Mark (Mark.java).
The Mark class will be very simple it will contain two private instance variables:
o studentName as a String
o studentMark as an integer
The following public methods will have to be implemented:
o A default constructor
o A parameterised constructor
o Two set methods (mutators)
o Two get methods (accessors)
ProcessMarks class
Once the Mark class is implemented and fully tested we can now start to implement the functionality of the menu system.
Data structures
For this assignment we are going to store the student names and marks in an array of Mark objects. Declare an array of Mark objects as an instance variable of ProcessMarks class the array should hold twenty students.
We need another instance variable (integer) to keep track of the number of the student being entered and use this for the index into the array of Mark objects.
Menu options
1. Enter student name and mark: enterStudentMark()
For assignment two we are going to use the GUI dialog showInputDialog() for our input. You will need to create the following two dialogs to receive the input from the user. You will not implement the functionality of the cancel key (need to use exceptions for this).
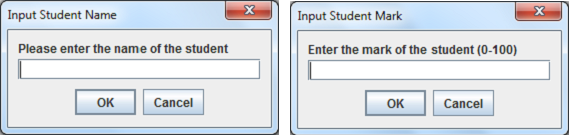
Data validation (you can implement this after you have got the basic functionality implemented) You will need to validate the user input using a validation loop.
The name cannot be blank i.e. not null and the mark needs to be between zero and one hundred (0- 100) inclusive.
Use the following pseudocode as a guide how to do this.
READ value
WHILE value is incorrect OUTPUT Error message READ value
END WHILE
Output the following error dialogs:
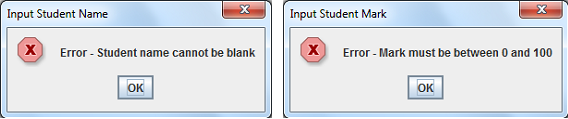
When the student name and mark has been entered successfully into two local variables you will need to add these values into the Mark object array, you will also need to increment a counter to keep track of the number of students you have entered and the position in the array of the next student to be entered.
When the maximum number of students is reached do not attempt to add any more students and give the following error message:
Error - maximum number of students to be entered has been reached
When the student details have been successfully entered display the details of the student on the console screen.
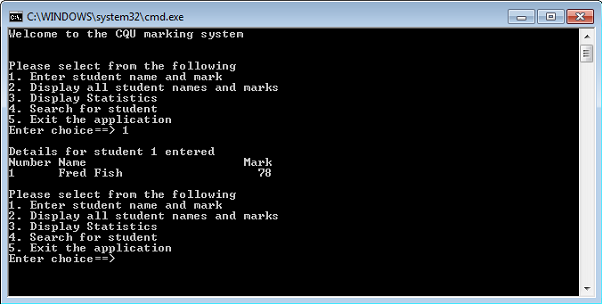
If no students have been entered the following menu options should display the following message:
You must enter at least one student to display all marks
2. Display all student names and marks: displayAllMarks()
When this option is selected display all of the students who have been entered so far.
Review the text p.933for the use of the printf method and using a format string. You could also use String.format() to format your output. Supplementary material to cover string formatting will be available in week 10.
3. Display statistics: displayStatistics()
When this option is selected you will display the statistics as per assignment one.
4. Search for student: searchStudent()
You can just use a simple linear search which will be case insensitive. Use the showInputDialog() method to input the name (you can share this functionality from enter student.
If the search is successful display the details about the student.
If the search is unsuccessful display an appropriate message.
Extra Hints
Your program should be well laid out, commented and uses appropriate and consistent names (camel notation) for all variables, methods and objects.
Make sure you have no repeated code (even writing headings in the output) Constants must be used for all numbers in your code.
Attachment:- JAVA.pdf