Reference no: EM131064237
Assignment Brief:
You are tasked with improving and designing part of the code for the Haunted House game. Please read thishandout and the code provided on Moodle.
To start
Play the game first!
To win the game, pick up five items (any item) from the ground by moving around the map and go back to when you started (Location 0). Use command "GET object" to get any object from the ground and "INVENTORY" to check how many items you currently have.
Section 1
The first four tasksin this section can be completed by modifying only the code within the ProcessStatement() and Game() functions. This section typically requires knowledge on lists, loops and if's.
1) Modify and display the Help message in the following fashion so it is easier for player to read and understand.
Recommended format:
Help - Display all possible actions you can carry out in this game
Score - Display your current score
Inventory - Display your inventory
... etc
2) Add a shortcut to all commands. If a command is one word, the shortcut should be made of the first letter and the last letter of the command. For example, hp for help, gt for get ...etc. If the command is two words, the shortcut should be made of the first letter of the two words. For example, od for open door ...etc.
3) Create a cheat commands in the game so player can teleport to any location in the map. The name of the command has to be 'tp'. Player has to entere a location number with the command e.g.tp 3will take player to location 3. If location number entered is not valid (not 0-63), player should stay at the same location and an error message should be displayed.
4) Modify the game so player can quit the game by entering 'quit'. You can not use exit() or quit() functions in this task. You have to exit the while loop!
5) Create a cheat commands in the game so player can pick up any item he wants from any location in the map. You have to name this cheat command 'giveme'. If wrong item name is entered, it should display an appropriate message and nothing will be given.
Example:
WHAT DO YOU WANT TO DO NEXT?giveme
POSSIBLE ITEMS TO GET:
PAINTING, RING, MAGIC SPELLS, GOBLET, SCROLL, COINS, STATUE, CANDLESTICK, MATCHES, VACUUM, BATTERIES, SHOVEL, AXE, ROPE, BOAT, AEROSOL, CANDLE, KEY
NAME THE ITEM YOU NEED: painting
PAINTING SUDDENLY APPEARED IN YOUR BAG
6) Make a menu with 3 options at the start of the game: New game, Continue and Quit. It should validate user's input so anything other than these three commands are not accepted and need to be re-entered.
Section 2
In addition to the knowledge required in section 1, this section requires knowledge on function, testing and file input/output.
7) Display your current location on the map with two asteriks (**). You need to modify the DisplayMap function for this task.
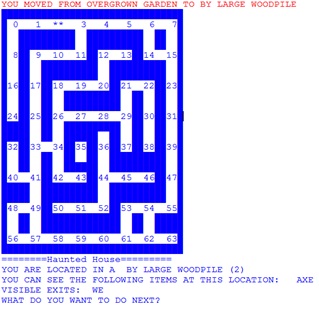
8) Add two new functionalities to the game: Save game and Load game. Player should be able to save the game state so that the game can be continued at a later stage (by loading it back). First you need to create two new commands: save and load.
What you need to save:
1. Player's current location,
2. Player's visited locations
3. Player's inventory.
9) Make a test plan for your game. It has to include 3 checkpoints for task 1, 2, 4 and 3 test cases for task 3 and 5. A template for this test plan will be provided to you.
Test your game before you submit your code using this test plan and record the result on the plan.
10) Design and implement 3 mini-missionsin the haunted house. This missions should involve player going to a special location on map, examining items, carrying out actions to find hints to win, new items or new exits. You have to document all 3 mini-missions in the documentation template given to you. You cannot reuse the ones that are already given to you in the code,but you can modify them to make your own.
a) The first mini-mission should involve player going to a special location on the map to examine something in order to find an item. The item should be hidden until the action is successfully carried out.
For example, player has to go to Library to examine books to find a map (item name)
b) The second mini-mission should involve player going to another special location on the map and carry out an action with an item to reveal a new exit. The exit should be hidden until the action is successfully carried out.
For example, player has to go to the study to read map to discover a new exit to the east (new exit should be revealed after map is read!).
c) The third mini-mission should involve player going to two separate places to find two different items and perform an action with those two items (in the inventory) at a special location. When this mission is successfully carried out, player should win the game.
For example, player has to go to the storage to find vaccum machine and then go to thestudy to find heavy duty batteries and do start vaccuming tokill the ghost insidethe Dark room.
Note. Player should be able to complete the game without reading any manual or documentation, so give enough hints along the way to playerto keep the game flowing and interesting. Display hints to help player findkey items and key places.