Reference no: EM131234264
Objectives
This assignment requires you to create two programs in Java for computers to accomplish two tasks: manage a play list of songs and simulating a new card game called BlackJoker.
Upon completion of this assignment, you should be able to create programs to accomplish tasks by analysing problems, implementing class designs and creating client programs of user-defined classes with interaction of objects.
General Requirements:
- You should create your programs with good programming style and form using proper blank spaces, indentation and braces to make your code easy to read and understand;
- You should create identifiers with sensible names;
- You should make comments to describe your code segments where they are necessary for readers to understand what your code intends to achieve.
- Logical structures and statements are properly used for specific purposes.
- Other requirements as stated in Submission section.
Program 1: Play list
You will create a program called MyPlaylist.java that manage your play list of songs. You should first implement the Song and Playlist classes modelled in the following UML classes. You should implement the classes as specified exactly without removing or adding any class members of the classes. All members of the classes are useful and must be used at least once.
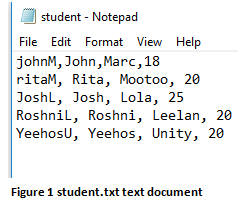
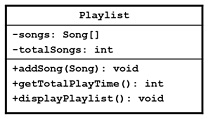
You will then create the main program MyPlaylist. The main program has the following interface and functionality as illustrated in the following sample run. You should implement the interface and the functionality in the main method by invoking appropriate methods of the classes modelled in the UML class diagrams. (User input is in red colour, the output of the playlist content in blue colour and prompt lines in black colour. Highlights in colours in this paper are for readability only.)
$java MyPlaylist
Welcome to my playlist!
1 - Add a new song
2 - List all songs
3 - Display total playtime
4 - exit
Please enter your choice:1
Title of the song: Closer
Name of the artist: Chainsmokers Duration (seconds): 240
1 - Add a new song
2 - List all songs
3 - Display total playtime
4 - exit
Please enter your choice:1
Title of the song: Heathens
Name of the artist: Twenty One Pilots Duration (seconds): 210
1 - Add a new song
2 - List all songs
3 - Display total playtime
4 - exit
Please enter your choice:1
Title of the song: Cold Water
Name of the artist: Major Lazer
Duration (seconds): 183
1 - Add a new song
2 - List all songs
3 - Display total playtime
4 - exit
Please enter your choice:2
Title: Closer Artist: Chainsmokers Duration(seconds): 240 Title: Heathens Artist: Twenty One Pilots Duration(seconds): 210 Title: Cold Water Artist: Major Lazer Duration(seconds): 183
1 - Add a new song
2 - List all songs
3 - Display total playtime
4 - exit
Please enter your choice:3 Total play time: 633
1 - Add a new song
2 - List all songs
3 - Display total playtime
4 - exit
Please enter your choice:4 Thank you. Bye!
When you are developing your program, you can run your program as follows to redirect the input file, input.txt, to the standard input for rapid testing.
$java MyPlaylist < input.txt
You need make sure your program can run properly with the provided input file redirected to the standard input as above.
Documentation
Provide documentation comments for all classes and methods using @author, @param and @return tags where appropriate.
Use the following command to create the documentation.
javadoc -author -d doc Song.java Playlist.java MyPlaylist.java
You do not need to submit the generated documents and do not do so. The documents will be generated when your code is marked.
Program 2: BlackJoker game
You will create a card game called BlackJoker, which is not Blackjack.
The game:
The cards:
- The cards have ranks from 2 to 10, J, Q, K and A with suits and the cards may not reappear.
- The hand values are the sum of card points according to the following table.
Rank
|
2
|
3
|
4
|
5
|
6
|
7
|
8
|
9
|
10
|
J
|
Q
|
K
|
A
|
Points
|
2
|
3
|
4
|
5
|
6
|
7
|
8
|
9
|
10
|
10
|
10
|
10
|
10
|
- The card suits are defined in the following table with their names, colours and symbols.
Suit
|
♠
|
♥
|
♦
|
♣
|
Name
|
Spades
|
Hearts
|
Diamonds
|
Clubs
|
Colour
|
Black
|
Red
|
Red
|
Black
|
Symbol
|
s
|
h
|
d
|
c
|
- The cards are in a random order of equiprobability.
The rules:
- The dealer receives its initial two cards and one card is face up.
- The player receives its initial two cards
- If the two initial cards in the hand received by either the dealer or the player are two cards from 10, J, Q, K or A in the same colour, it is called BlackJoker and the hand wins. The player wins a tie.
- If no one wins with BlackJoker, the game continues.
- The player makes a decision among two options: "Hit" or "Stand".
- If the player hits, it takes another card until it stands.
- If the player stands, it takes no more cards then the dealer will takes cards.
- The dealer will take cards until the hand busts or achieves a value of 17 or higher.
- If either the player or the dealer exceeds 20 points at any time, it busts and loses the game immediately.
- If no one busts, the hand of higher values wins and the player wins a tie.
Important Note: You must implement the above rules. If the game plays other rules such as the rules of Blackjack, you will receive 0 mark for this program.
Task 1: Implement Card, CardDeck and Player classes
You should implement all classes modelled in the following UML diagrams. You should implement the classes as specified exactly without removing or adding any class members of the classes. You need analyse the rules of the game and the gameplay as in the sample runs to determine the behaviours of the objects and the semantics of the methods as in the UML diagrams. All members of the classes are useful and must be used at least once. The ranks and suits members of the CardDeck class are used to define the values of card ranks and suits, respectively.
Task 2 (for CSIT111 students): Create a single player BlackJoker program
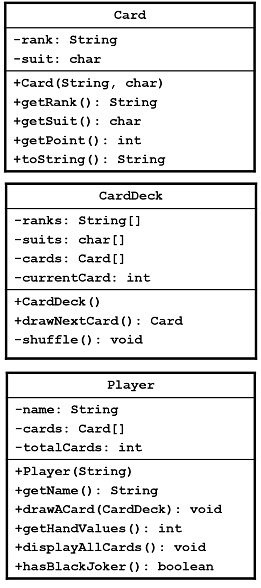
You will create a single player game in the file BlackJoker.java. You should implement the interface and the rules of the game in the main method by invoking appropriate methods of the classes modelled in Task 1.
Some sample runs of the program are as follows. (User input is in red colour, the card ranks and points in blue colour, prompt lines in black colour and results in green colour. Highlights in colours in this paper are for readability only.)
$java BlackJoker
Welcome to the game of BlackJoker!
What's your name? Peter
Dealer's hand: 10d Total: 10 Peter's hand: Ah 4c Total: 14
Hit(H or h) or Stand(S or s):h
Peter's hand: Ah 4c 5d Total: 19
Hit(H or h) or Stand(S or s):S
Dealer's hand: 10d Ks Total: 20 Dealer wins!
$java BlackJoker
Welcome to the game of BlackJoker!
What's your name? John
Dealer's hands: 6h Total: 6 John's hand: 9d Qc Total: 19
Hit(H or h) or Stand(S or s):s
Dealer's hand: 6h Ah Jd Total: 26 Dealer busts!
$java BlackJoker
Welcome to the game of BlackJoker!
What's your name? Peter Dealer's hand: 6s Total: 6 Peter's hand: Qh Jh Total: 20 Dealer's hand: 6s 9h Total: 15 Peter has BlackJoker! Peter wins
$java BlackJoker
Welcome to the game of BlackJoker!
What's your name? Peter Dealer's hand: Ad Total: 10 Peter's hand: 8h 3h Total: 11 Dealer's hand: Ad Qh Total: 20
Dealer has BlackJoker! The dealer wins
$java BlackJoker
Welcome to the game of BlackJoker!
What's your name? Bob
Dealer's hand: 2s Total: 2 Bob's hand: 9d 3c Total: 12
Hit(H or h) or Stand(S or s):H Bob's hand: 9d 3c Qs Total: 22 Bob busts!
Task 2 (for CSIT811 students): Create a multiplayer BlackJoker program
The extended rules for multiplayer games:
The multiplayer game follows all the rules specified in The rules section and the following extensions:
- All players play the game in sequence one after another after previous player stands;
- The game will continue unless all players have BlackJoker;
- If the dealer has BlackJoker, the dealer wins except players who have BlackJoker as well;
- If a player busts, it loses immediately;
You will create a multiplayer game in the file BlackJoker.java. You should implement the interface and these rules of the game in the main method by invoking appropriate methods of the classes modelled in Task 1.
Your program should first take the number of players and all players' name at the beginning as follows:
How many players? 2
Player 1's your name? Peter Player 2's your name? John
Then display the dealer's first card before each player plays the game. After all players finish their game, display the final results of all players who have not busted as follows:
Dealer's hand: 9s 9h Total: 18
Peter's hand: Qh Js Total: 20 Peter wins
John's hand: 2d Qc Total: 12 Dealer wins!
Submission
- All your source code files should include your Subject Code and assignment number, your name, your user ID, and your student number in the header.
- IMPORTANT: Any submission that cannot be decompressed may receive zero mark. Any submission that resulted in compiling errors may have 50% marks deducted.
- You should first zip your source files into a file called a3.zip. For this assignment you submit all your source files excluding all provided test and main programs, bytecode files and any files that may be generated by the IDE you might have used. Extra files may interfere with the marking process. Failing to do so may result in deduction of marks.
- Submit the file from your University UINX account on Banshee (banshee.uow.edu.au) as follows:
submit -u YourUserID -c CSIT111 -a a3 a3.zip