Reference no: EM131067649
Task 1-
For this task you will create a Subject class, whose instances will represent the subjects for study at a university. A subject will have a name, just a String, and a subject code, which is a six-character String. The first three characters of a subject code are alphabetic and the last three are numeric. The first three characters define the subject's discipline area. A subject code must be unique.
You will also write a TestSubject class to test the use of your Subject class. In particular this will maintain an array of subjects. In order to manage the uniqueness of the subject codes, your program will need to display information about existing subject codes as well as checking that any new subject code supplied by the user is not the same as any existing subject code.
The following state and functionality should be provided for the Subject class:
- Two fields will hold the subject's name and the six-character subject code.
- A constructor will allow a name and a new, validated subject code to be provided when a new subject is created.
- Getters will provide access to the attributes.
- An accessor method called getDiscipline will return a string containing the first three characters of the subject code.
- Another accessor method called codeMatches will return a boolean value indicating if the subject's code matches the string argument provided. "Matches" is used here in the same sense as for the matches method of the String class.
- A toString method will return a string containing the subject code and subject name.
To assist with managing subject codes and their uniqueness you will provide the Subject class with some class methods as follows:
- An allDisciplines method will accept an array of Subject objects. It will return an array containing the different 3-character discipline codes represented in the array of subjects in alphabetically order.
- A codesPerDiscipline method will accept an array of Subject objects and a 3-character discipline code. It will return an array containing the different subject codes represented in the array of subjects for the particular discipline.
- An isValidCode method will accept a string that is a possible new subject code, and return a boolean indicating whether it satisfies the structural requirements for a subject code.
- A codeExists method will accept an array of Subject objects and a possible new subject code. It will return a boolean indicating whether that code has already been allocated to one of the subjects in the array.
- A sortDisciplines method will accept an array of Subjects objects. It will return the sorted array of subjects in alphabetically order.
Your TestSubject program will perform the following sequence of actions, using good design techniques such as in the appropriate use of methods:
- An initial array of Subject objects will be created from any data in a file that was previously saved by the program.
- The user interaction will then proceed to allow the user to add one or more new subjects to the array. If the user wishes to add new subjects, the discipline areas of existing subjects should be displayed in alphabetically order. The user will then enter a discipline code to which the program will respond by displaying any existing subject codes in that discipline. This procedure simplifies the user's task of choosing subject codes that do not already exist, but does not prevent user mistake. Each subject code entered by the user should be checked. The user can enter any new subjects in that discipline (or indeed in other disciplines). The user should be given the choice of repeating the processing for other discipline areas.
- When the user has finished adding subjects, and only if subjects have indeed been added, the program will overwrite the data file with the updated data.
Note:
- You may use an ArrayList to implement an array if you prefer and it is appropriate.
Task 2
Design a class named Octagon that extends GeometricObject class and implements the Comparable and Cloneable interface. Assume that all eight sides of the octagon are of equal size. The area can be computed using following formula:
Area = (2 + 4/√2) x side x side
Draw the UML diagram that involves Octagon, GeometricObjects, Comparable, and Cloneable. Write a test program that creates an Octagon object with side values 5 and display its area and perimeter. Create a new object using clone method and compare the two objects using compareTo method.
GeometricObject class can be found in textbook. It is also given below.
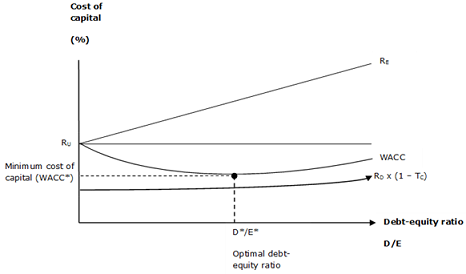
Task 3-
Write a Java programs which displays a frame that contains three labels. Each label displays a card, as shown in the figure below. The card image files are named 1.png, 2.png, ..., 54.png and stored in the image/card directory. All three cards are distinct and selected randomly.
The image icons used in the questions are available in the Resource folder of Interact
The image icons used in the questions are available.
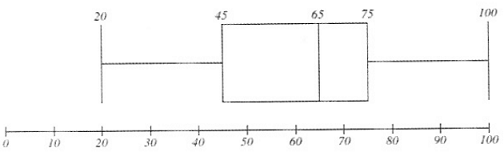
Your Task:
1. Create three JLabel and set their icons using the images.
2. Display three images from 54 image cards randomly.
Attachment:- Card.7z
Privileged members in society
: Name the method consists of the ability to interact in a manner that is customary to the more privileged members in society.
|
What can you say about the numbers a, b, c, and d
: What can you say about the numbers a, b, c, and d?
|
Submit a succinct abstract
: Please submit a succinct abstract of what you have in mind for the Project. All you need to send are two short paragraphs. In the first, put in the quote you will use as your "Theoretical Framework," and attribute it properly.
|
What are some benefits and drawbacks of the expository
: What are some benefits and drawbacks of the expository, guided inquiry, and free discovery teaching methodologies? Discuss.
|
Create three jlabel and set their icons using the images
: Write a Java programs which displays a frame that contains three labels. Each label displays a card, as shown in the figure below. Create three JLabel and set their icons using the images. Display three images from 54 image cards randomly
|
Explain why develop written goals prioritize your work
: Discuss the techniques you use to manage your time wisely. Explain why Develop Written goals and objectives, prioritize your Work and Work Efficiently on Team Project are useful for building time management techniques.
|
Research-transnational motherhood and paid domestic work
: 1) Please do provide me 8 points of "Research/ transnational Motherhood and Paid domestic work" 2) Transnational mothering 6 points on Netherland
|
A result of ethical and regulatory issues-failures
: The written report/ paper should be between six to eight pages in length, doubled space with 12 point font; plus an additional/ separate page with at least three references.
|
Write a system of four equations whose solution estimates
: The temperature at a node is approximately equal to the average of the four nearest nodes-to the left, above, to the right, and below.3 For instance
|