Web Services in ASP.Net:
A web service is a web-related functionality given using the protocols of the web to be needed by the web applications. There are three types of web service development:
- Preparing the web service
- Making a proxy
- Using the web service
Creating the Web Sevice:
A web service is a web application which is normally a class consisting of methods that should be used by other applications. It also gives a code-behind architecture like the ASP.Net web pages, although it could not have an user interface.
To study the concept let us make a web service that will give stock price information. The clients may query about the price and name of a stock based on the stock symbol. To take this example simple, the values are hardcoded in a two-dimensional array. This web service application can have three methods:
- A defaullt HelloWorld method
- A GetName Method
- A GetPrice Method
Take the subsequent steps to prepare the web service:
Step (1): Select File--> New --> Web Site in Visual Studio, and then choose ASP.Net Web Service.
Step (2): A web service file known as Service.asmx and its code after that file, Service.cs is prepared in the App_Code directory of the project.
Step (3): modification the names of the files to StockService.cs and StockService.asmx.
Step (4): The .asmx file has normally a WebService directive on it:
<%@ WebService Language="C#"
CodeBehind="~/App_Code/StockService.cs"
Class="StockService" %>
|
Step (5): Open the StockService.cs file, the code prepared in it is the basic Hello World application. The default web service code after file looks like the subsequent:
using System;
using System.Collections;
using System.ComponentModel;
using System.Data;
using System.Linq;
using System.Web;
using System.Web.Services;
using System.Web.Services.Protocols;
using System.Xml.Linq;
namespace StockService
{
/// <summary>
/// Summary description for Service1
/// <summary>
[WebService(Namespace = "https://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[ToolboxItem(false)]
// To allow this Web Service to be called from script,
// using ASP.NET AJAX, uncomment the following line.
// [System.Web.Script.Services.ScriptService]
public class Service1 : System.Web.Services.WebService
{
[WebMethod]
public string HelloWorld()
{
return "Hello World";
}
}
}
|
Step (6): modify the code behind file to include the two dimensional array of strings for stock symbol, price and name and two web functions for getting the stock data.
using System;
using System.Linq;
using System.Web;
using System.Web.Services;
using System.Web.Services.Protocols;
using System.Xml.Linq;
[WebService(Namespace = "https://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
// To allow this Web Service to be called from script,
// using ASP.NET AJAX, uncomment the following line.
// [System.Web.Script.Services.ScriptService]
public class StockService : System.Web.Services.WebService
{
public StockService () {
//Uncomment the following if using designed components
//InitializeComponent();
}
string[,] stocks =
{
{"RELIND", "Reliance Industries", "1060.15"},
{"ICICI", "ICICI Bank", "911.55"},
{"JSW", "JSW Steel", "1201.25"},
{"WIPRO", "Wipro Limited", "1194.65"},
{"SATYAM", "Satyam Computers", "91.10"}
};
[WebMethod]
public string HelloWorld() {
return "Hello World";
}
[WebMethod]
public double GetPrice(string symbol)
{
//it takes the symbol as parameter and returns price
for (int i = 0; i < stocks.GetLength(0); i++)
{
if (String.Compare(symbol, stocks[i, 0], true) == 0)
return Convert.ToDouble(stocks[i, 2]);
}
return 0;
}
[WebMethod]
public string GetName(string symbol)
{
// It takes the symbol as parameter and
// returns name of the stock
for (int i = 0; i < stocks.GetLength(0); i++)
{
if (String.Compare(symbol, stocks[i, 0], true) == 0)
return stocks[i, 1];
}
return "Stock Not Found";
}
}
|
Step (7) : Executing the web service application provides a web service test page, which gives testing the service function.
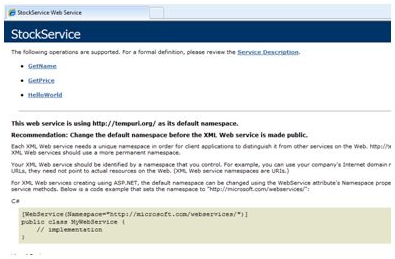
Step (8) : Click on a function name, and check whether it execute properly.
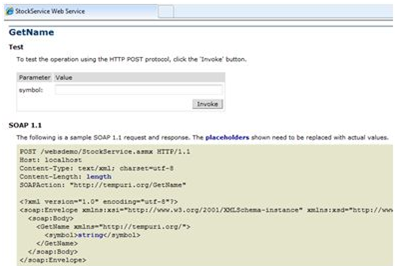
Step (9): For testing the GetName method, give one of the stock symbols, which are hard programmed, it gives the name of the stock

Consuming the Web Service:
For using the web application service, make a web site under the same solution. This should be done by right clicking on the Solution name in the Solution Explorer. The web page executing the web service could have a label control to show the returned results and two button controls one for post back and another for naming the service.
The content file for the web application is as given:
<%@ Page Language="C#"
AutoEventWireup="true"
CodeBehind="Default.aspx.cs"
Inherits="wsclient._Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"https://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="https://www.w3.org/1999/xhtml" >
<head runat="server">
<title>Untitled Page</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h3>Using the Stock Service</h3>
<br />
<br />
<asp:Label ID="lblmessage" runat="server"></asp:Label>
<br />
<br />
<asp:Button ID="btnpostback" runat="server"
onclick="Button1_Click"
Text="Post Back" Width="132px" />
<asp:Button ID="btnservice" runat="server"
onclick="btnservice_Click"
Text="Get Stock" Width="99px" />
</div>
</form>
</body>
</html>
|
The code inside the file for the web application is as given:
using System;
using System.Collections;
using System.Configuration;
using System.Data;
using System.Linq;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.HtmlControls;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Xml.Linq;
//this is the proxy
using localhost;
namespace wsclient
{
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
lblmessage.Text = "First Loading Time: " +
DateTime.Now.ToLongTimeString();
else
lblmessage.Text = "PostBack at: " +
DateTime.Now.ToLongTimeString();
}
protected void btnservice_Click(object sender, EventArgs e)
{
StockService proxy = new StockService();
lblmessage.Text = String.Format("Current SATYAM Price:{0}",
proxy.GetPrice("SATYAM").ToString());
}
}
}
|
Creating the Proxy:
A proxy is a stand-in for the web application service codes. Before needing the web service, a proxy has to be prepared. The proxy is send with the client application. Then the client application creates the calls to the web application service as it were using a local function.
The proxy gives the calls, wraps it in proper format and transmit it as a SOAP request to the server. SOAP stands for Simple Object Access Protocol. This process is used for interchanging web service data.
When the server gives the SOAP package to the client, the proxy converts everything and shows it to the client application.
Before naming the web application service using the btnservice_Click, a web reference could be included to the application. This makes a proxy class transparently, which is needed by the btnservice_Click event.
protected void btnservice_Click(object sender, EventArgs e)
{
StockService proxy = new StockService();
lblmessage.Text = String.Format("Current SATYAM Price: {0}",
proxy.GetPrice("SATYAM").ToString());
}
|
Take the subsequent steps for building the proxy:
Step (1): Right click on the web application entry in the Solution Explorer and press on 'Add Web Reference'.
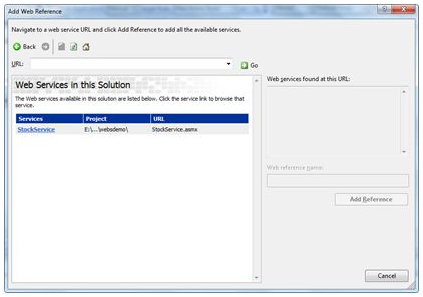
Step (2): Choose 'Web Services in this solution'. It gives the StockService reference.
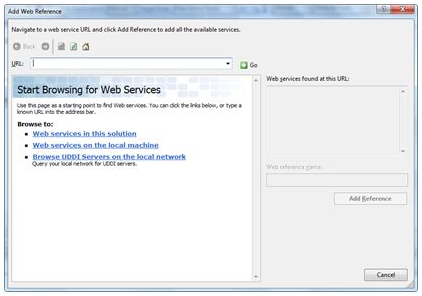
Step (3): Clicking on the service starts the test web page. By default the proxy prepared is known as 'localhost', you may name it. Click on 'Add Reference' to include the proxy to the client application.
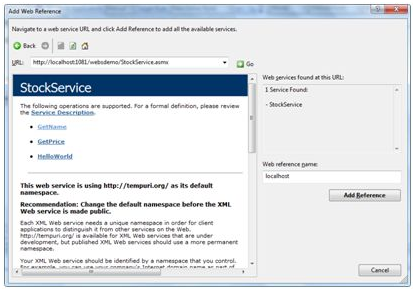
Adding the proxy in the code under file by adding:
Email based ASP.Net assignment help - homework help at Expertsmind
Are you searching ASP.Net expert for help with Web Services in ASP.Net questions? Web Services in ASP.Net topic is not easier to learn without external help? We at www.expertsmind.com offer finest service of ASP.Net assignment help and ASP.Net homework help. Live tutors are available for 24x7 hours helping students in their Web Services in ASP.Net related problems. Computer science programming assignments help making life easy for students. We provide step by step Web Services in ASP.Net question's answers with 100% plagiarism free content. We prepare quality content and notes for Web Services in ASP.Net topic under ASP.Net theory and study material. These are avail for subscribed users and they can get advantages anytime.
Why Expertsmind for assignment help
- Higher degree holder and experienced experts network
- Punctuality and responsibility of work
- Quality solution with 100% plagiarism free answers
- Time on Delivery
- Privacy of information and details
- Excellence in solving ASP.Net queries in excels and word format.
- Best tutoring assistance 24x7 hours