Managing state in ASP.Net:
HTTP ( Hyper Text Transfer Protocol) is a stateless protocol. When the client dis connects from the server, the ASP.Net engine dis cards the page objects. That way every web application may scale up to serve numerous requests simultaneously without running out of server storage.
However, there require to some method to store the information between requests and to get back it when required. This information i.e., the present value of all the controls and variables for the present user in the present session is called the State.
ASP.Net handles four types of state:
1. View State
2. Control State
3. Session State
4. Application State
View State:
The View State is the condition of the page and all its controls. It is manually maintained across posts by the ASP.Net framework.
When a page is sent back to the client, the modifies in the properties of the page and its controls are indomitable and stored in the value of a unknown input field named _VIEWSTATE. When the page is again post back the _VIEWSTATE field is launched to the server with the HTTP request.
The view state should be allow or disabled for:
- The entire application - by locating the EnableViewState property in the <pages> section of web.config file
- A page - by locating the EnableViewState attribute of the Page directive, as <%@ Page Language="C#" EnableViewState="false" %>
- A control - by locating the Control.EnableViewState property.
It is shown using a view state object described by the StateBag class which described a collection of view state items. The state bag is a data structure holding attribute/value pairs, stored as strings linked with objects.
The StateBag class has the subsequent properties:
Properties
|
Description
|
Item(name)
|
The value of the view state item with the particular name. This is the default property of the StateBag class
|
Count
|
The number of items in the view state set
|
Keys
|
Collection of keys for all the items in the set
|
Values
|
Collection of values for all the items in the set
|
The StateBag class has the subsequent methods
Methods
|
Description
|
Add(name, value)
|
Inserts an item to the view state collection and existing item is updated
|
Clear
|
Eliminates all the items from the collection
|
Equals(Object)
|
Calculates whether the particular object is equal to the current object.
|
Finalize
|
Allows it to free resources and do other cleanup operations.
|
GetEnumerator
|
takings an enumerator that iterates over all the key/value pairs of the StateItem objects accumulate in the StateBag object.
|
GetType
|
Gets the Type of the present instance.
|
IsItemDirty
|
Checks a StateItem object stored in the StateBag object to estimate whether it has been modified.
|
Remove(name)
|
Removes the particular item.
|
SetDirty
|
Sets the state of the StateBag object as well as the Dirty property of every of the StateItem objects contained by it.
|
SetItemDirty
|
Sets the Dirty property for the particular StateItem object in the StateBag object.
|
ToString
|
Returns a String showing the state bag object.
|
Example:
The following example shows the concept of storing view state. Let us take a counter, which is added each time the page is post back by clicking a button on the page. A label control indicates the value in the counter.
The markup file:
<%@ Page Language="C#"
AutoEventWireup="true"
CodeBehind="Default.aspx.cs"
Inherits="statedemo._Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"https://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="https://www.w3.org/1999/xhtml" >
<head runat="server">
<title>Untitled Page</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h3>View State demo</h3>
Page Counter:
<asp:Label ID="lblCounter" runat="server" />
<asp:Button ID="btnIncrement" runat="server"
Text="Add Count"
onclick="btnIncrement_Click" />
</div>
</form>
</body>
</html>
|
The code at the back file for the example is shown here:
public partial class _Default : System.Web.UI.Page
{
public int counter
{
get
{
if (ViewState["pcounter"] != null)
{
return ((int)ViewState["pcounter"]);
}
else
{
return 0;
}
}
set
{
ViewState["pcounter"] = value;
}
}
protected void Page_Load(object sender, EventArgs e)
{
lblCounter.Text = counter.ToString();
counter++;
}
}
|
This would construct following result:
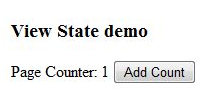
Control State:
Control state cannot be customized, accessed directly or disabled.
Session State:
When a user attaches to an ASP.Net website, a new session object is prepared. When session state is turned on, a new session state object is formed for each new request. This session state object becomes part of the context and it is existing through the page.
Session state is usually used for storing application data like inventory or supplier list, shopping cart or a customer record. It may also save information about the user and his preference and keep track of pending operations.
Sessions are denoted and tracked with a 120-bit SessionID, which is gone from client to server and back as cookie or a changed URL. The SessionID is globally related and random.
The session state object is prepared from the HttpSessionState class, which describes a collection of session state items.
The HttpSessionState class has the subsequent properties:
Properties
|
Description
|
SessionID
|
The single session identifier
|
Item(name)
|
The value of the session state item with the particular name. This is the default property of the HttpSessionState class
|
Count
|
The number of items in the session state collection
|
TimeOut
|
Sets and gets the amount of time, in minutes, allowed between requests previous to the session-state provider terminates the session.
|
The HttpSessionState class has the following methods:
Methods
|
Description
|
Add(name, value)
|
Includes an item to the session state collection
|
Clear
|
Eliminates all the items from session state collection
|
Remove(name)
|
Eliminates the specified item from the session state collection
|
RemoveAll
|
Eliminates all keys and values from the session-state collection.
|
RemoveAt
|
Eliminates an item at a particular index from the session-state collection.
|
The session state object is a name-value pair, to store and get back some information from the session state object the following code should be used:
void StoreSessionInfo()
{
String fromuser = TextBox1.Text;
Session["fromuser"] = fromuser;
}
void RetrieveSessionInfo()
{
String fromuser = Session["fromuser"];
Label1.Text = fromuser;
}
|
The above code saves only strings in the Session dictionary object, however, it may store all the primitive data types and arrays composed of primitive data, as well as the DataSet, HashTable, DataTable and Image objects, as well as any user described class that inherits from the ISerializable object.
Example:
The following example shows the concept of saving session state. There are two buttons on the page, a text box to enter string and a label to show the text stored from last session.
The mark up file:
<%@ Page Language="C#"
AutoEventWireup="true"
CodeFile="Default.aspx.cs"
Inherits="_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"https://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="https://www.w3.org/1999/xhtml" >
<head runat="server">
<title>Untitled Page</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<table style="width: 568px; height: 103px">
<tr>
<td style="width: 209px">
<asp:Label ID="lblstr" runat="server"
Text="Enter a String" Width="94px">
</asp:Label>
</td>
<td style="width: 317px">
<asp:TextBox ID="txtstr" runat="server" Width="227px">
</asp:TextBox>
</td>
</tr>
<tr>
<td style="width: 209px"></td>
<td style="width: 317px"></td>
</tr>
<tr>
<td style="width: 209px">
<asp:Button ID="btnnrm" runat="server"
Text="No action button" Width="128px" />
</td>
<td style="width: 317px">
<asp:Button ID="btnstr" runat="server"
OnClick="btnstr_Click" Text="Submit the String" />
</td>
</tr>
<tr>
<td style="width: 209px">
</td>
<td style="width: 317px">
</td>
</tr>
<tr>
<td style="width: 209px">
<asp:Label ID="lblsession" runat="server"
Width="231px">
</asp:Label>
</td>
<td style="width: 317px">
</td>
</tr>
<tr>
<td style="width: 209px">
<asp:Label ID="lblshstr" runat="server">
</asp:Label>
</td>
<td style="width: 317px">
</td>
</tr>
</table>
</div>
</form>
</body>
</html>
|
It could look like the following in design view:
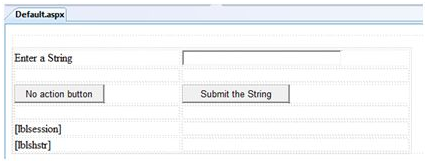
The code at the back file is given here:
public partial class _Default : System.Web.UI.Page
{
String mystr;
protected void Page_Load(object sender, EventArgs e)
{
this.lblshstr.Text = this.mystr;
this.lblsession.Text = (String)this.Session["str"];
}
protected void btnstr_Click(object sender, EventArgs e)
{
this.mystr = this.txtstr.Text;
this.Session["str"] = this.txtstr.Text;
this.lblshstr.Text = this.mystr;
this.lblsession.Text = (String)this.Session["str"];
}
}
|
Run the file and watch how it works:
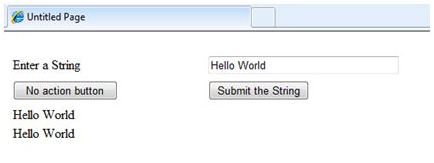
Application State
An ASP.Net application is the collection of all code, web pages and other files within a single virtual directory on a web server. When data is saved in application state, it is available to all the users.
To give for the use of application state, ASP.Net prepares an application state object for every application from the HTTPApplicationState class and saves this object in server memory. This object is shown by class file global.asax.
Application State is currently used to store hit counters and other statistical data, global application data like discount rate, tax rate etc and to keep track of users visiting the site.
The HttpApplicationState class has the subsequent properties:
Properties
|
Description
|
Item(name)
|
The value of the application state item with the particular name. This is the default property of the HttpApplicationState class.
|
Count
|
The number of items in the application state collection.
|
The HttpApplicationState class has the following methods:
Methods
|
Description
|
Add(name, value)
|
Includes an item to the application state collection .
|
Clear
|
Eliminates all the items from the application state collection.
|
Remove(name)
|
Eliminates the specified item from the application state collection.
|
RemoveAll
|
Eliminates all objects from an HttpApplicationState collection.
|
RemoveAt
|
Eliminates an HttpApplicationState object from a collection by index.
|
Lock()
|
Locks the application state collection so only the present user may access it.
|
Unlock()
|
Unlocks the application state collection so all the users may access it.
|
Application state data is usually organized by writing handlers for the events:
- Application_Start
- Application_End
- Application_Error
- Session_Start
- Session_End
The subsequent code snippet indicates the basic syntax for storing application state information:
Void Application_Start(object sender, EventArgs e)
{
Application["startMessage"] = "The application has started.";
}
Void Application_End(object sender, EventArgs e)
{
Application["endtMessage"] = "The application has ended.";
}
|
Email based ASP.Net assignment help - homework help at Expertsmind
Are you searching ASP.Net expert for help with Managing state in ASP.Net questions? Managing state in ASP.Net topic is not easier to learn without external help? We at www.expertsmind.com offer finest service of ASP.Net assignment help and ASP.Net homework help. Live tutors are available for 24x7 hours helping students in their Managing state in ASP.Net related problems. Computer science programming assignments help making life easy for students. We provide step by step Managing state in ASP.Net question's answers with 100% plagiarism free content. We prepare quality content and notes for Managing state in ASP.Net topic under ASP.Net theory and study material. These are avail for subscribed users and they can get advantages anytime.
Why Expertsmind for assignment help
- Higher degree holder and experienced experts network
- Punctuality and responsibility of work
- Quality solution with 100% plagiarism free answers
- Time on Delivery
- Privacy of information and details
- Excellence in solving ASP.Net queries in excels and word format.
- Best tutoring assistance 24x7 hours