Error Handling in ASP.Net:
Error handling in ASP.Net has three aspects:
1. Tracing - tracing the program run at page level or application level.
2. Error handling - handling standard errors or custom errors at application level or page level
3. Debugging - stepping through the program, setting break points to get the code
In this tutorial, we are going to define tracing and error handling and we will look into debugging in the next tutorial.
To check the concepts, makes the following sample application. It has a label control, a dropdown list and a link. The dropdown list loads in an array list of famous quotes and the chosen quote is given in the label below. It also has a hyperlink which has a nonexistent link.
<%@ Page Language="C#"
AutoEventWireup="true"
CodeBehind="Default.aspx.cs"
Inherits="errorhandling._Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"https://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="https://www.w3.org/1999/xhtml" >
<head runat="server">
<title>Tracing, debugging and error handling</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:Label ID="lblheading" runat="server"
Text="Tracing, Debuggin and Error Handling">
</asp:Label>
<br />
<br />
<asp:DropDownList ID="ddlquotes"
runat="server" AutoPostBack="True"
onselectedindexchanged="ddlquotes_SelectedIndexChanged">
</asp:DropDownList>
<br />
<br />
<asp:Label ID="lblquotes" runat="server">
</asp:Label>
<br />
<br />
<asp:HyperLink ID="HyperLink1" runat="server"
NavigateUrl="mylink.htm">Link to:</asp:HyperLink>
</div>
</form>
</body>
</html>
|
The code behind file:
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
string[,] quotes =
{
{"Imagination is more important than Knowledge.",
"Albert Einsten"},
{"Assume a virtue, if you have it not",
"Shakespeare"},
{"A man cannot be comfortable without his own
approval", "Mark Twain"},
{"Beware the young doctor and the old barber",
"Benjamin Franklin"},
{"Whatever begun in anger ends in shame",
"Benjamin Franklin"}
};
for (int i=0; i<quotes.GetLength(0); i++)
ddlquotes.Items.Add(new ListItem(quotes[i,0],
quotes[i,1]));
}
}
protected void ddlquotes_SelectedIndexChanged(object sender,
EventArgs e)
{
if (ddlquotes.SelectedIndex != -1)
{
lblquotes.Text = String.Format("{0}, Quote: {1}",
ddlquotes.SelectedItem.Text, ddlquotes.SelectedValue);
}
}
}
|
Tracing:
To enable page level tracing, you require to change the Page directive and include a Trace attribute as:
<%@ Page Language="C#" AutoEventWireup="true"
CodeBehind="Default.aspx.cs"
Inherits="errorhandling._Default"
Trace ="true" %>
|
Now when you execute the file, you get the tracing information:
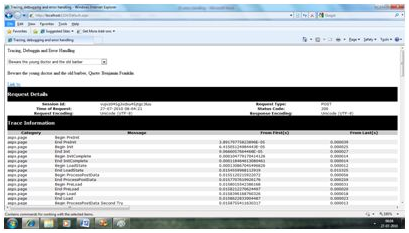
It gives the subsequent information at the top:
- Session ID
- Status Code
- Time of Request
- Type of Request
- Request and Response Encoding
The status code provides from the server, each time the page is requested indicates the name and time of error if any. The given table indicates the common HTTP status codes :
Number
|
Description
|
Informational (100 - 199)
|
100
|
Continue
|
101
|
Switching protocols
|
Successful (200 - 299)
|
200
|
OK
|
204
|
No content
|
Redirection (300 - 399)
|
301
|
Moved permanently
|
305
|
Use proxy
|
307
|
Temporary redirect
|
Client Errors (400 - 499)
|
400
|
Bad request
|
402
|
Payment required
|
404
|
Not found
|
408
|
Request timeout
|
417
|
Expectation failed
|
Server Errors (500 - 599)
|
500
|
Internal server error
|
503
|
Service unavailable
|
505
|
HTTP version not supported
|
Under the top level information is the Trace log, which gives details of page life cycle. It gives elapsed time in seconds since the page was initialized.
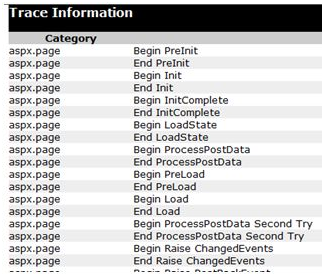
The next part is control tree, which lists all controls on the page in a hierarchical manner:
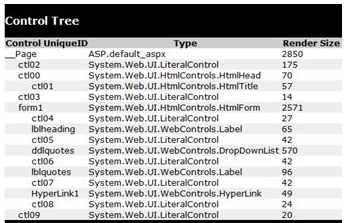
Last in the part and Application state summaries, headers and cookies collections, related by list of all server variables.
The Trace object allows you to include custom information to the trace output. It has two methods to complete this: the Write method and the Warn method.
Modify the Page_Load event handler to check the Write method:
protected void Page_Load(object sender, EventArgs e)
{
Trace.Write("Page Load");
if (!IsPostBack)
{
Trace.Write("Not Post Back, Page Load");
string[,] quotes =
.......................
|
Run to observe the effects:
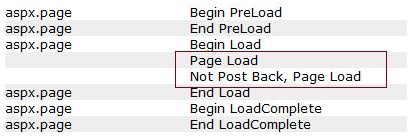
To calculate the Warn function, let us forcibly enter some erroneous code in the marked index modified event handler:
try
{
int a = 0;
int b = 9 / a;
}
catch (Exception e)
{
Trace.Warn("UserAction", "processing 9/a", e);
}
|
Try-Catch is a C# programming construct. The try block holds any code that can or may not give error and the catch block catches the error. When the program is executing, it sends the warning in the trace log.

Application level tracing applies to each and every page in the web site. It is derived by putting the subsequent code lines in the web.config file:
<system.web>
<trace enabled="true" />
</system.web>
|
Error Handling:
Although ASP.Net may check all runtime errors, still some subtle errors may still be occurred. Observing the errors by tracing is meant for the developers, not for the users.
Hence, to intercept such occurrence, you can include error handing settings in the web.config file of the application. It is application wide error handling. For example, you can include the subsequent lines in the web.config file:
<configuration>
<system.web>
<customErrors mode="RemoteOnly"
defaultRedirect="GenericErrorPage.htm">
<error statusCode="403" redirect="NoAccess.htm" />
<error statusCode="404" redirect="FileNotFound.htm" />
</customErrors>
</system.web>
<configuration>
|
The <customErrors> section has the possible attributes:
- Mode: It actives or disables custom error pages. It has the three possible types:
- On: shows the custom pages.
- Off: shows ASP.Net error pages (yellow pages)
- remoteOnly: shows custom errors to client, shows ASP.Net errors locally
- defaultRedirect: It has the URL of the page to be shown in case of unhandled errors.
To put different custom error pages for different kind of errors, the <error> sub tags are needed, where different error pages are given, based on the status code of the errors.
To shown page level error handling, the Page directive should be changed:
<%@ Page Language="C#" AutoEventWireup="true"
CodeBehind="Default.aspx.cs"
Inherits="errorhandling._Default"
Trace ="true"
ErrorPage="PageError.htm" %>
|
Email based ASP.Net assignment help - homework help at Expertsmind
Are you searching ASP.Net expert for help with Error Handling in ASP.Net questions? Error Handling in ASP.Net topic is not easier to learn without external help? We at www.expertsmind.com offer finest service of ASP.Net assignment help and ASP.Net homework help. Live tutors are available for 24x7 hours helping students in their Error Handling in ASP.Net related problems. Computer science programming assignments help making life easy for students. We provide step by step Error Handling in ASP.Net question's answers with 100% plagiarism free content. We prepare quality content and notes for Error Handling in ASP.Net topic under ASP.Net theory and study material. These are avail for subscribed users and they can get advantages anytime.
Why Expertsmind for assignment help
- Higher degree holder and experienced experts network
- Punctuality and responsibility of work
- Quality solution with 100% plagiarism free answers
- Time on Delivery
- Privacy of information and details
- Excellence in solving ASP.Net queries in excels and word format.
- Best tutoring assistance 24x7 hours