Data Source Control in ASP.Net:
A data source control communicates with the data-bound controls and hides the complex data binding methods. These are the tools that give data to the data bound controls and support execution of operations like deletions, sorting, insertions and updates.
Each data source control wraps a specific data gives-relational databases, XML documents or custom classes and helps in:
- Managing connection.
- Selection of data
- Managing presentation aspects like paging, caching etc.
- Manipulation of data
There are several data source controls available in ASP.Net for taking data from SQL Server, from ODBC or OLE DB servers, from XML files and from business objects.
Lied on type of data, these controls should be spited into two categories: hierarchical data source controls and table-based data source controls.
The data source controls needed for hierarchical data are:
- XMLDataSource-allows binding to strings and XML files with or without schema information
- SiteMapDataSource-allows binding to a provider that supplies site map data
The data source controls used for tabular data are:
Data source controls
|
Description
|
SqlDataSource
|
shows a connection to an ADO.Net data provider that returns SQL data, including data sources accessible via OLEDB and QDBC
|
ObjectDataSource
|
gives binding to a custom .Net business object that returns data
|
LinqdataSource
|
gives binding to the results of a Linq-to-SQL query (supported by ASP.Net 3.5 only)
|
AccessDataSource
|
shows connection to a Microsoft Access database
|
The Data Source Views
Data source views are objects of the DataSourceView class and show a customized view of data for different data operations like filtering, sorting etc.
The DataSourceView class serves as the base class for all data source view classes, which describes the capabilities of data source controls.
Following table gives the properties of the DataSourceView class:
Properties
|
Description
|
CanDelete
|
Shows whether deletion is allowed on the underlying data source.
|
CanInsert
|
Shows whether insertion is allowed on the underlying data source.
|
CanPage
|
Shows whether paging is allowed on the underlying data source.
|
CanRetrieveTotalRowCount
|
Shows whether total row count information is available.
|
CanSort
|
Shows whether the data could be sorted.
|
CanUpdate
|
Shows whether updates are allowed on the underlying data source.
|
Events
|
Gets a list of event-handler delegates for the data source view.
|
Name
|
Name of the view on the page.
|
subsequent table gives the methods of the DataSourceView class:
Methods
|
Description
|
CanExecute
|
Calculates whether the specified command can be executed.
|
ExecuteCommand
|
Run the specific command.
|
ExecuteDelete
|
Operates a delete operation on the list of data that the DataSourceView object shows.
|
ExecuteInsert
|
Operates an insert operation on the list of data that the DataSourceView object shows.
|
ExecuteSelect
|
Gets a list of data from the underlying data storage.
|
ExecuteUpdate
|
Performs an update operation on the list of data that the DataSourceView object shows.
|
Delete
|
Operates a delete operation on the data associated with the view.
|
Insert
|
Operates an insert operation on the data associated with the view.
|
Select
|
Returns the queried data.
|
Update
|
Operates an update operation on the data associated with the view.
|
OnDataSourceViewChanged
|
Increased the DataSourceViewChanged event.
|
RaiseUnsupportedCapabilitiesError
|
Called by the RaiseUnsupportedCapabilitiesError function to compare the capabilities requested for an ExecuteSelect operation against those that the view supports.
|
The SqlDataSource Control
The SqlDataSource control shows a connection to a relational database such as SQL Server or Oracle database, or data accessible through Open Database Connectivity (ODBC) or OLEDB. Connection to data is prepared through two important properties ProviderName and ConnectionString.
The subsequent code snippet gives the basic syntax for the control:
<asp:SqlDataSource runat="server" ID="MySqlSource"
ProviderName='<%$ ConnectionStrings:LocalNWind.ProviderName %>'
ConnectionString='<%$ ConnectionStrings:LocalNWind %>'
SelectionCommand= "SELECT * FROM EMPLOYEES" />
<asp:GridView ID="GridView1" runat="server"
DataSourceID="MySqlSource">
|
Configuring several data operations on the underlying data lies upon the several properties (property groups) of the data source control.
The subsequent table saves the related sets of properties of the SqlDataSource control, which gives the programming interface of the control:
Property Group
|
Description
|
DeleteCommand, DeleteParameters, DeleteCommandType
|
Sets or gets the SQL statement, parameters and type for deleting rows in the underlying data.
|
FilterExpression, FilterParameters
|
Sets or gets the data filtering string and parameters.
|
InsertCommand, InsertParameters, InsertCommandType
|
Sets or gets the SQL statement, parameters and type for inserting rows in the underlying database.
|
SelectCommand, SelectParameters, SelectCommandType
|
Sets or gets the SQL statement, parameters and type for retrieving rows from the underlying database.
|
SortParameterName
|
Sets or gets the name of an input parameter that the command's stored procedure will use to sort data
|
UpdateCommand, UpdateParameters, UpdateCommandType
|
Sets or gets the SQL statement, parameters and type for updating rows in the underlying data store.
|
The subsequent code snippet shows a data source control enabled for data manipulation:
<asp:SqlDataSource runat="server" ID= "MySqlSource"
ProviderName='<%$ ConnectionStrings:LocalNWind.ProviderName %>'
ConnectionString=' <%$ ConnectionStrings:LocalNWind %>'
SelectCommand= "SELECT * FROM EMPLOYEES"
UpdateCommand= "UPDATE EMPLOYEES SET LASTNAME=@lame"
DeleteCommand= "DELETE FROM EMPLOYEES WHERE EMPLOYEEID=@eid"
FilterExpression= "EMPLOYEEID > 10">
.....
.....
</asp:SqlDataSource>
|
The ObjectDataSource Control:
The ObjectDataSource Control actives user-defined classes to relate the output of their function to data bound controls. The programming interface of this class is almost similar as the SqlDataSource control.
subsequent are two important parts of binding business objects:
- The bindable class could have a default constructor, be stateless, and have function that may be mapped to select, update, insert and delete semantics.
- The object must modify one item at a time, batch operations are not executed.
Let us go directly to an example to execute with this control. The student class is our class to be used with an object data source. This class has three basic properties: a student id, name and city. It has a default constructor and a GetStudents function to be used for retrieving data.
The student class:
public class Student
{
public int StudentID { get; set; }
public string Name { get; set; }
public string City { get; set; }
public Student()
{ }
public DataSet GetStudents()
{
DataSet ds = new DataSet();
DataTable dt = new DataTable("Students");
dt.Columns.Add("StudentID", typeof(System.Int32));
dt.Columns.Add("StudentName", typeof(System.String));
dt.Columns.Add("StudentCity", typeof(System.String));
dt.Rows.Add(new object[] { 1, "M. H. Kabir", "Calcutta" });
dt.Rows.Add(new object[] { 2, "Ayan J. Sarkar", "Calcutta" });
ds.Tables.Add(dt);
return ds;
}
}
|
Take the subsequent steps to relate the object with an object data source and save data:
- Makes a new web site. Include a class (Students.cs) to it by right clicking the project from the Solution Explorer, including a class template and placing the above code in it.
- Prepare the solution so that the application may use the reference to the class.
- Place a object data source control in the web page.
- Configure the data source by choosing the object.
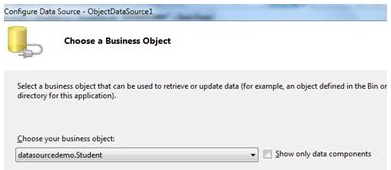
- Choose a data method(s) for different functions on data. In this example, there is only one function.
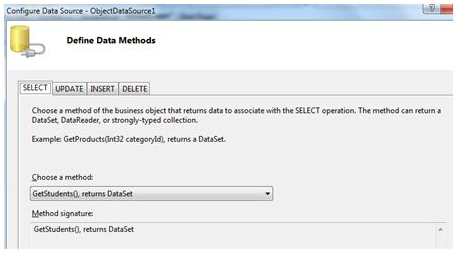
- Place a data bound control like grid view on the page and choose the object data source as its underlying data source
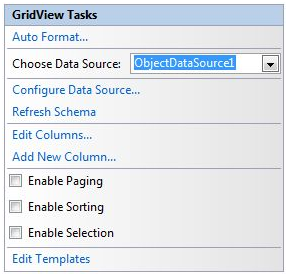
- At that part, the design view could look like the following:
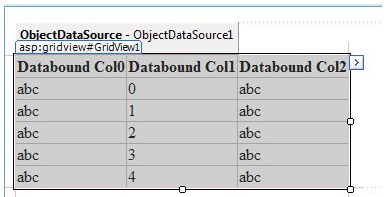
- Execute the project, it retrieves the hard coded tuples from the students class.
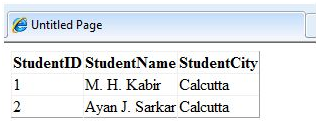
The AccessDataSource Control:
The AccessDataSource control shows a connection to an Access database. It is related on the SqlDataSource control and gives simpler programming interface. The subsequent code snippet gives the basic syntax for the data source:
<asp:AccessDataSource ID="AccessDataSource1"
runat="server"
DataFile="~/App_Data/ASPDotNetStepByStep.mdb"
SelectCommand="SELECT * FROM [DotNetReferences]">
</asp:AccessDataSource>
|
The AccessDataSource control read the database in read-only mode. However, it may also be used for performing insert, delete or update operations. This is done using the ADO.Net parameter collection and commands.
Updates are problematic for Access databases from within an ASP.Net application due to an Access database is a plain file and the default part of the ASP.Net application may not have the rights to write to the database file.
Email based ASP.Net assignment help - homework help at Expertsmind
Are you searching ASP.Net expert for help with Data Source Control in ASP.Net questions? Data Source Control in ASP.Net topic is not easier to learn without external help? We at www.expertsmind.com offer finest service of ASP.Net assignment help and ASP.Net homework help. Live tutors are available for 24x7 hours helping students in their Data Source Control in ASP.Net related problems. Computer science programming assignments help making life easy for students. We provide step by step Data Source Control in ASP.Net question's answers with 100% plagiarism free content. We prepare quality content and notes for Data Source Control in ASP.Net topic under ASP.Net theory and study material. These are avail for subscribed users and they can get advantages anytime.
Why Expertsmind for assignment help
- Higher degree holder and experienced experts network
- Punctuality and responsibility of work
- Quality solution with 100% plagiarism free answers
- Time on Delivery
- Privacy of information and details
- Excellence in solving ASP.Net queries in excels and word format.
- Best tutoring assistance 24x7 hours