Data Binding in ASP.Net:
Every ASP.Net web form control adds the DataBind function from its parent Control class, which gives it an inherent capability to bind data to at least one of its attributes. This is known as simple data binding or inline data binding.
Simple data binding adds attaching any set (item collection) which denotes the the DataSet or IEnumerable interface and DataTable classes to the DataSource property of the control.
On the other hand, some controls can be bound records, columns or lists of data into their structure through a DataSource control. These controls gives from the BaseDataBoundControl class. This is called as declarative data binding.
The data source controls help the data-bound controls intiated functionalities like paging, sorting and editing data collections. We have normally taken declarative data binding in the last tutorial.
The BaseDataBoundControl is an abstract class, which is given by two more abstract classes:
- DataBoundControl
- HierarchicalDataBoundControl
The abstract class DataBoundControl is again included by two more abstract classes:
- ListControl
- CompositeDataBoundControl
The controls capable of simple data binding are started from the ListControl abstract class and these controls are:
- BulletedList
- CheckBoxList
- DropDownList
- ListBox
- RadioButtonList
The controls capable of declarative data relating (a more complex data binding) are joining from the abstract class CompositeDataBoundControl. These controls are:
- DetailsView
- FormView
- GridView
- RecordList
Simple Data Binding:
Simple data binding adds the read-only filed lists. These controls can bind to fields or an array list from a database. Selection lists gives two values from the data source or the database; one value is given by the list and the other is related as the value corresponding to the display.
Let us take up a small eg. to validate the concept. Make a web site with a bulleted list and a SqlDataSource control on it. Configure the data source control to give two values from your database.
Choosing a data source for the bulleted list control involves:
- Choosing the data source control
- Choosing a field to display, which is called the data field
- Choosing a field for the value
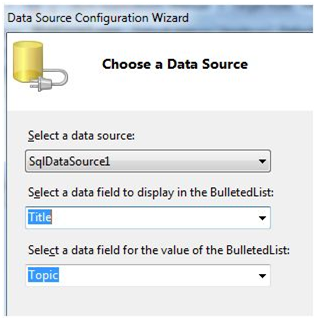
When the application is execute, check that the entire title column is bound to the bulleted list and shown.
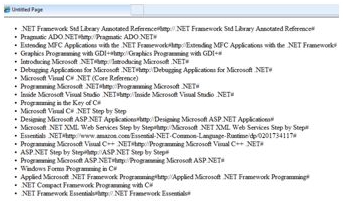
Declarative Data Binding:
We have already used declarative data binding in the last tutorial using GridView control. The other composite data bound buttons capable of showing and changing data in a tabular manner are the DetailsView, FormView and RecordList control.
However, the data binding adds the following objects:
- A dataset that saves the data gets from the database
- The data provider, which gets data from the database, using a command over a connection
- The data adapter that issues choose statement stored in the command object; it is also capable of update the data in a database by issuing Insert, Update, and Delete statements.
Relation between the data binding objects:
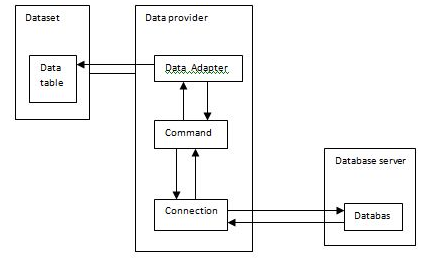
Example:
Take the subsequent steps:
Step (1): Make a new website. Include a class named booklist by right clicking on the solution name in the Solution Explorer and selected the item 'Class' from the 'Add Item' dialog box. Name it booklist.cs
using System;
using System.Data;
using System.Configuration;
using System.Linq;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.HtmlControls;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Xml.Linq;
namespace databinding
{
public class booklist
{
protected String bookname;
protected String authorname;
public booklist(String bname, String aname)
{
this.bookname = bname;
this.authorname = aname;
}
public String Book
{
get
{
return this.bookname;
}
set
{
this.bookname = value;
}
}
public String Author
{
get
{
return this.authorname;
}
set
{
this.authorname = value;
}
}
}
}
|
Step (2): Included four list controls on the page-a list box control, a radio button list, a check box list and a drop down list and four labels along with these list controls. The page could look like this in design view:
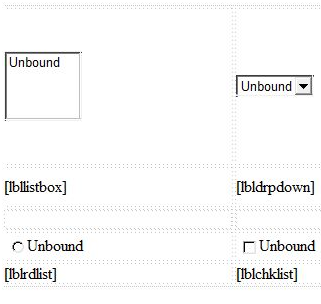
The source file could look as the following:
<form id="form1" runat="server">
<div>
<table style="width: 559px">
<tr>
<td style="width: 228px; height: 157px;">
<asp:ListBox ID="ListBox1" runat="server" AutoPostBack="True"
OnSelectedIndexChanged="ListBox1_SelectedIndexChanged">
</asp:ListBox></td>
<td style="height: 157px">
<asp:DropDownList ID="DropDownList1" runat="server"
AutoPostBack="True"
OnSelectedIndexChanged="DropDownList1_SelectedIndexChanged">
</asp:DropDownList>
</td>
</tr>
<tr>
<td style="width: 228px; height: 40px;">
<asp:Label ID="lbllistbox" runat="server"></asp:Label>
</td>
<td style="height: 40px">
<asp:Label ID="lbldrpdown" runat="server">
</asp:Label>
</td>
</tr>
<tr>
<td style="width: 228px; height: 21px">
</td>
<td style="height: 21px">
</td>
</tr>
<tr>
<td style="width: 228px; height: 21px">
<asp:RadioButtonList ID="RadioButtonList1" runat="server"
AutoPostBack="True"
OnSelectedIndexChanged="RadioButtonList1_SelectedIndexChanged">
</asp:RadioButtonList></td>
<td style="height: 21px">
<asp:CheckBoxList ID="CheckBoxList1" runat="server"
AutoPostBack="True"
OnSelectedIndexChanged="CheckBoxList1_SelectedIndexChanged">
</asp:CheckBoxList></td>
</tr>
<tr>
<td style="width: 228px; height: 21px">
<asp:Label ID="lblrdlist" runat="server">
</asp:Label></td>
<td style="height: 21px">
<asp:Label ID="lblchklist" runat="server">
</asp:Label></td>
</tr>
</table>
</div>
</form>
|
Step (3): Finally write the subsequent code at the back routines for the application:
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
IList bklist = createbooklist();
if (!this.IsPostBack)
{
this.ListBox1.DataSource = bklist;
this.ListBox1.DataTextField = "Book";
this.ListBox1.DataValueField = "Author";
this.DropDownList1.DataSource = bklist;
this.DropDownList1.DataTextField = "Book";
this.DropDownList1.DataValueField = "Author";
this.RadioButtonList1.DataSource = bklist;
this.RadioButtonList1.DataTextField = "Book";
this.RadioButtonList1.DataValueField = "Author";
this.CheckBoxList1.DataSource = bklist;
this.CheckBoxList1.DataTextField = "Book";
this.CheckBoxList1.DataValueField = "Author";
this.DataBind();
}
}
protected IList createbooklist()
{
ArrayList allbooks = new ArrayList();
booklist bl;
bl = new booklist("UNIX CONCEPTS", "SUMITABHA DAS");
allbooks.Add(bl);
bl = new booklist("PROGRAMMING IN C", "RICHI KERNIGHAN");
allbooks.Add(bl);
bl = new booklist("DATA STRUCTURE", "TANENBAUM");
allbooks.Add(bl);
bl = new booklist("NETWORKING CONCEPTS", "FOROUZAN");
allbooks.Add(bl);
bl = new booklist("PROGRAMMING IN C++", "B. STROUSTROUP");
allbooks.Add(bl);
bl = new booklist("ADVANCED JAVA", "SUMITABHA DAS");
allbooks.Add(bl);
return allbooks;
}
protected void ListBox1_SelectedIndexChanged(object sender,
EventArgs e)
{
this.lbllistbox.Text = this.ListBox1.SelectedValue;
}
protected void DropDownList1_SelectedIndexChanged(
object sender, EventArgs e)
{
this.lbldrpdown.Text = this.DropDownList1.SelectedValue;
}
protected void RadioButtonList1_SelectedIndexChanged(
object sender, EventArgs e)
{
this.lblrdlist.Text = this.RadioButtonList1.SelectedValue;
}
protected void CheckBoxList1_SelectedIndexChanged(
object sender, EventArgs e)
{
this.lblchklist.Text = this.CheckBoxList1.SelectedValue;
}
}
|
Observe the subsequent:
- The booklist class has two properties authorname and bookname.
- The createbooklist function is a user describes method that makes an array of booklist objects named allbooks.
- The Page_Load event handler makes sure that a list of books is prepared. The list is of IList type, which shows the IEnumerable interface and capable of being related to the list controls. The page load event handler relates the IList object 'bklist' with the list controls. The bookname property is to be shown and the authorname property is taken as the value.
- When the page is execute, if the user chooses a book, its name is chosen and shown by the list controls whereas the corresponding labels show the author name, which is the related value for the selected index of the list control.
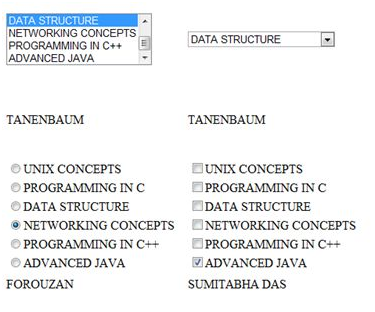
Email based ASP.Net assignment help - homework help at Expertsmind
Are you searching ASP.Net expert for help with Data Binding in ASP.Net questions? Data Binding in ASP.Net topic is not easier to learn without external help? We at www.expertsmind.com offer finest service of ASP.Net assignment help and ASP.Net homework help. Live tutors are available for 24x7 hours helping students in their Data Binding in ASP.Net related problems. Computer science programming assignments help making life easy for students. We provide step by step Data Binding in ASP.Net question's answers with 100% plagiarism free content. We prepare quality content and notes for Data Binding in ASP.Net topic under ASP.Net theory and study material. These are avail for subscribed users and they can get advantages anytime.
Why Expertsmind for assignment help
- Higher degree holder and experienced experts network
- Punctuality and responsibility of work
- Quality solution with 100% plagiarism free answers
- Time on Delivery
- Privacy of information and details
- Excellence in solving ASP.Net queries in excels and word format.
- Best tutoring assistance 24x7 hours